Simple KCC

Overview
The Simple KCC is a user-friendly and intuitive 3D character controller addon. It is designed to bring simplicity and ease-to-use characters to your game projects using Fusion.
Creating a smooth and responsive character controller is a critical aspect of any game project. Traditional character controller solutions can be complex and challenging to integrate, especially for developers seeking a streamlined and efficient workflow. This is where Simple KCC shines — by offering a simplified yet powerful solution for character movement.
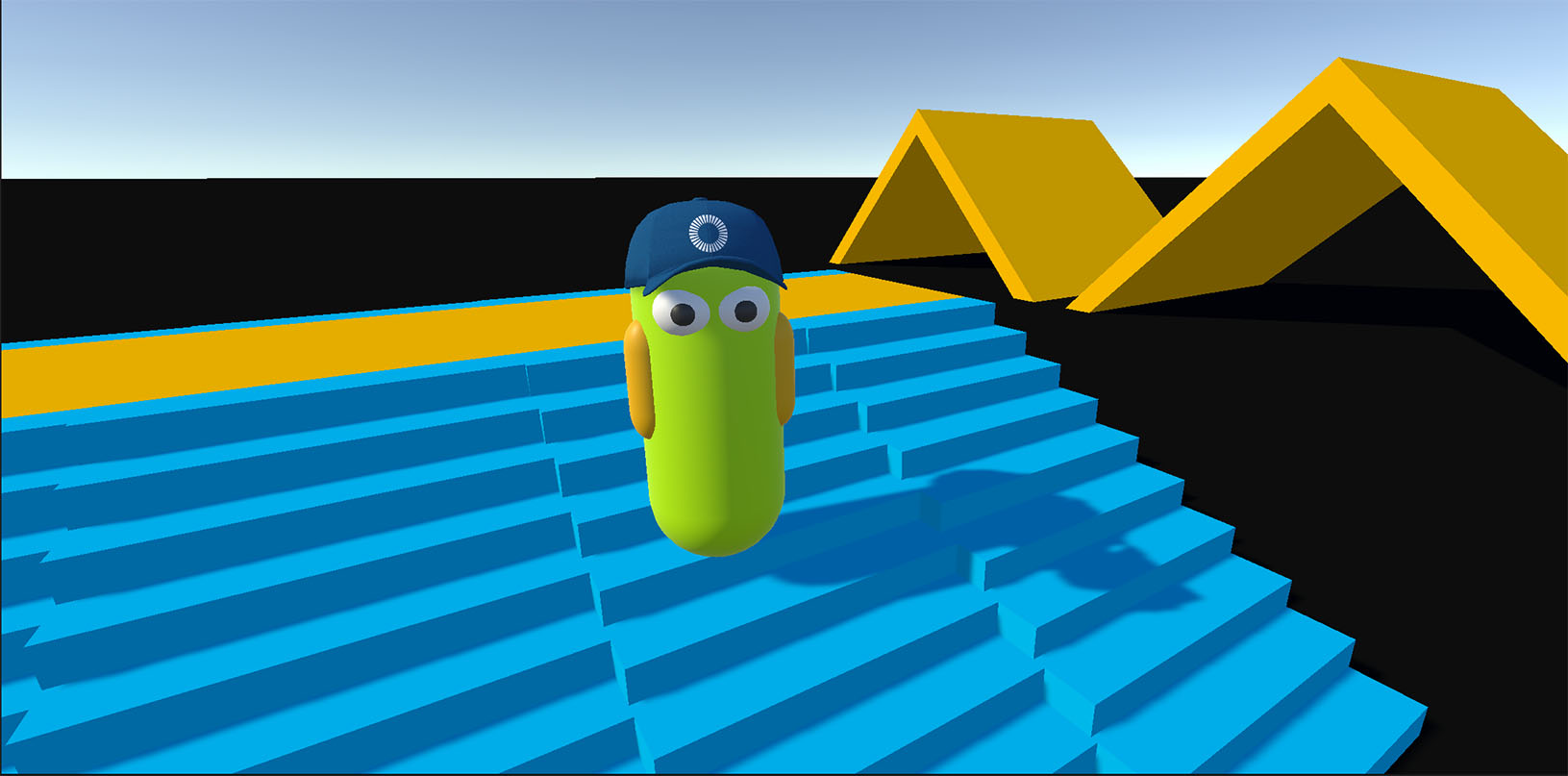
Features
- Easy integration and configuration.
- Control over position and look rotation (pitch + yaw).
- Capsule based physics query and depenetration.
- Velocity based movement.
- Support for Gravity.
- Support for Jump.
- Basic ground detection.
- Continuous collision detection (CCD) out of the box.
- Separate game object for collider (created at runtime as child game object).
- Support for ignoring child colliders.
- Step detection and ground snapping.
- Platform independent, mobile friendly.
- Network & performance optimized.
- Pooling compatible.
- Fully commented sample with testing playground.
Download - Sample
Version | Release Date | Download | |
---|---|---|---|
2.0.10 | Apr 18, 2024 | Fusion Simple KCC Sample 2.0.10 Build 493 |
Download - Addon
Version | Release Date | Download | |
---|---|---|---|
2.0.7 | Apr 18, 2024 | Fusion Simple KCC 2.0.7 Build 492 |
Requirements
- Unity 2021.3
- Fusion AppId: To run the sample, first create a Fusion AppId in the PhotonEngine Dashboard and paste it into the
App Id Fusion
field in Real Time Settings (reachable from the Fusion menu in Unity editor). Continue with instructions in First Steps section.
Sample Controls
- Use
W
,S
,A
,D
for movement,SPACE
for jumping, andMouse
to look. - Lock or release your cursor inside the Unity Editor using the
ENTER
key.
Open Assets/Scenes/Playground
and play. The scene can be used for evaluation (how the character and its parameters behave) or to prototype movement for your game.
First Steps
The best way to start with Simple KCC is downloading sample project which is pre-configured and ready to be tested with multiple players.
In the following step-by-step guide, we'll walk you through the process of integrating Simple KCC addon into your game project from scratch.
- Import Simple KCC addon package into your Fusion 2.0 project.
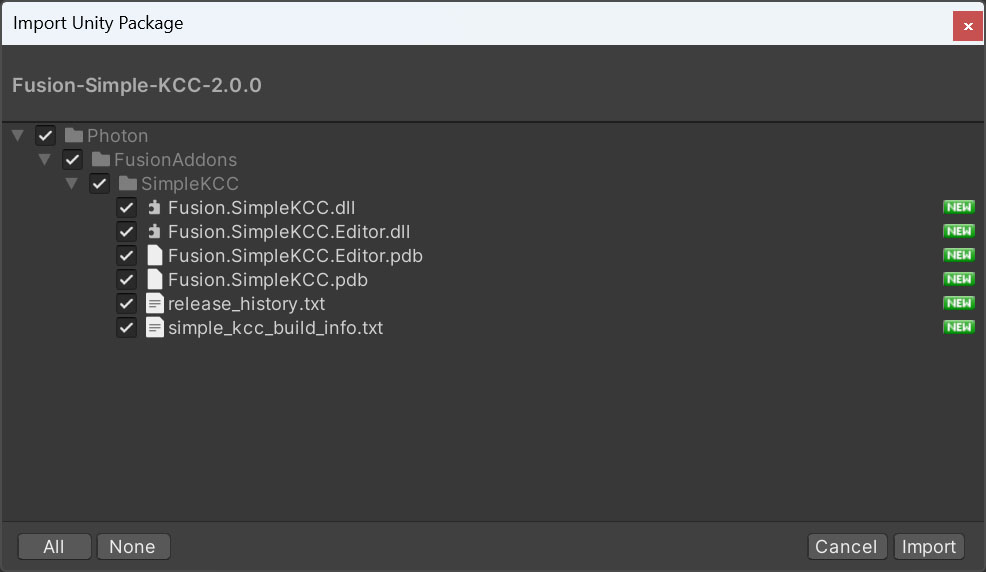
- Create a player prefab.
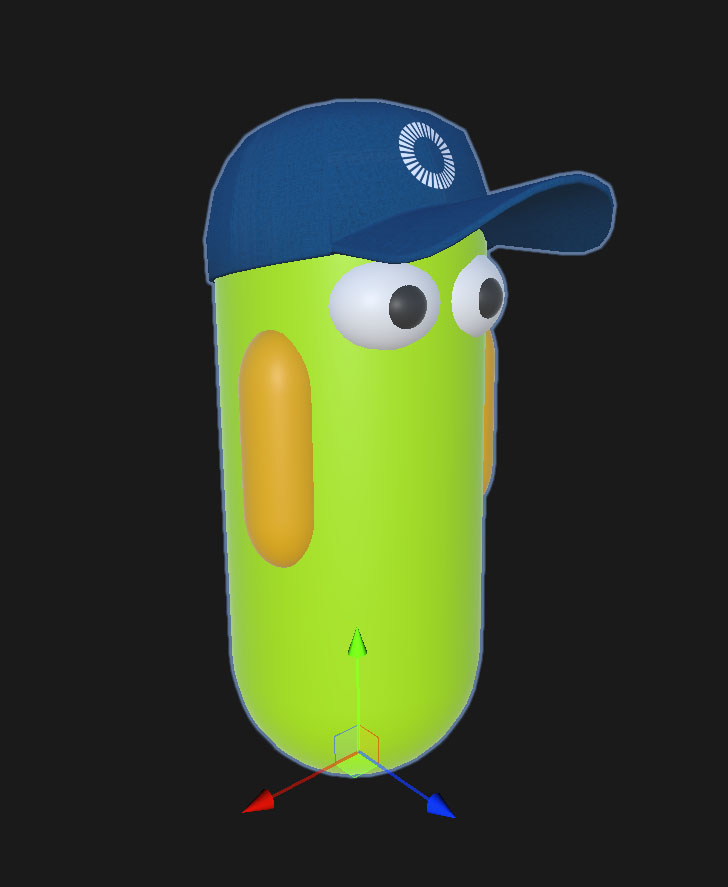
- Add
NetworkObject
andSimpleKCC
components to the root game object. EnableIs Kinematic
option onRigidBody
component.
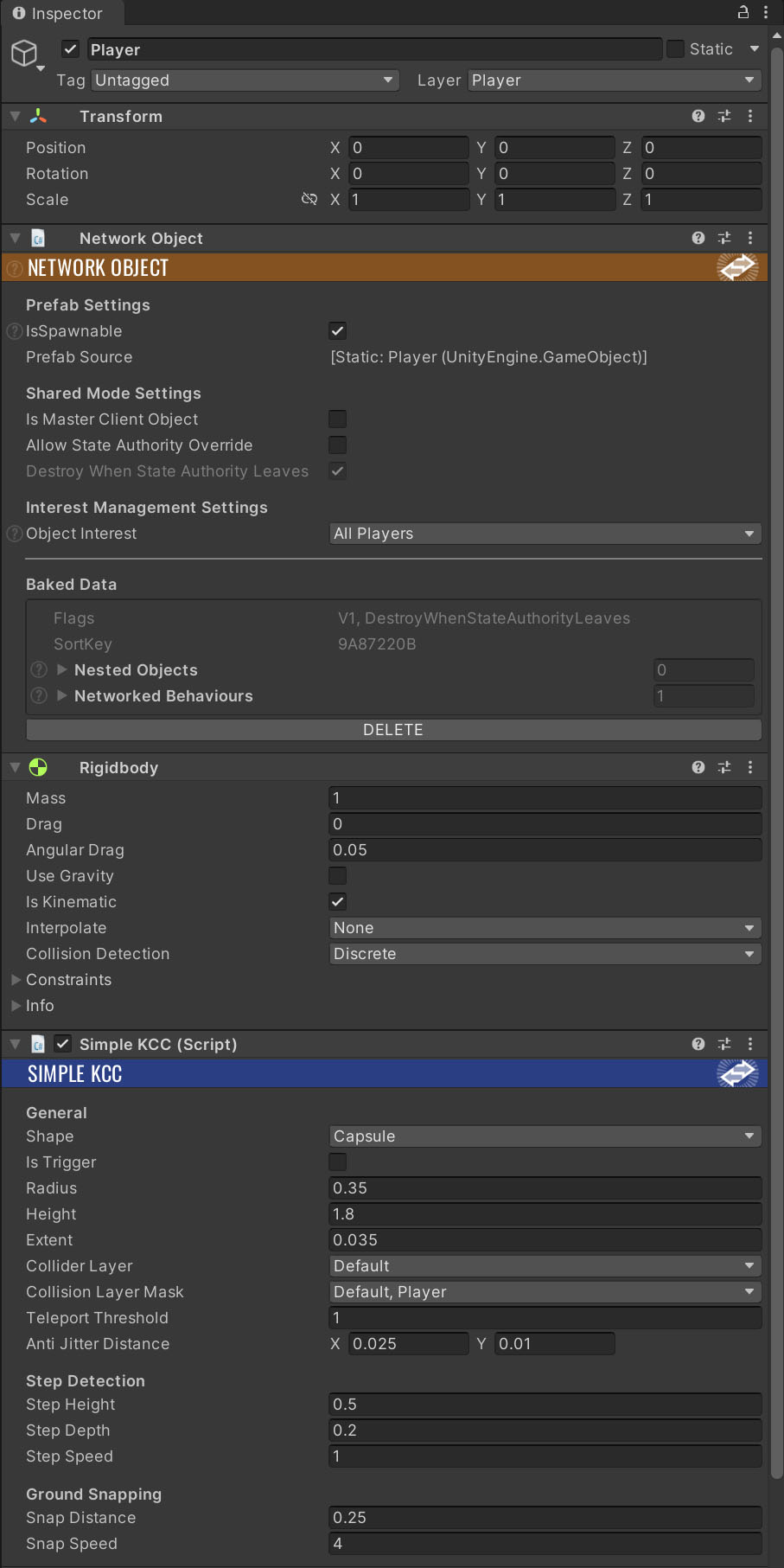
- Configure parameters (radius, height, collision mask, ...). More details in Configuration section.
- Link the player prefab to your spawn manager.
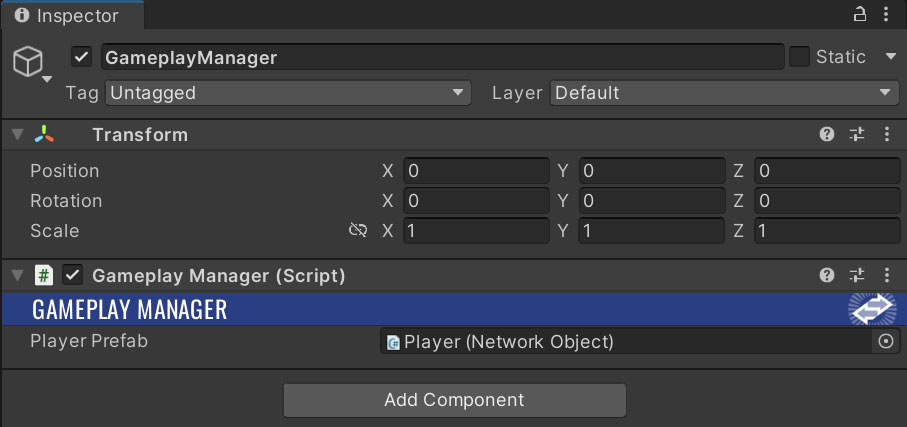
- Move with the character from code. More details in Movement section.
Configuration
The Simple KCC
component inspector provides a set of options to fine-tune behavior.
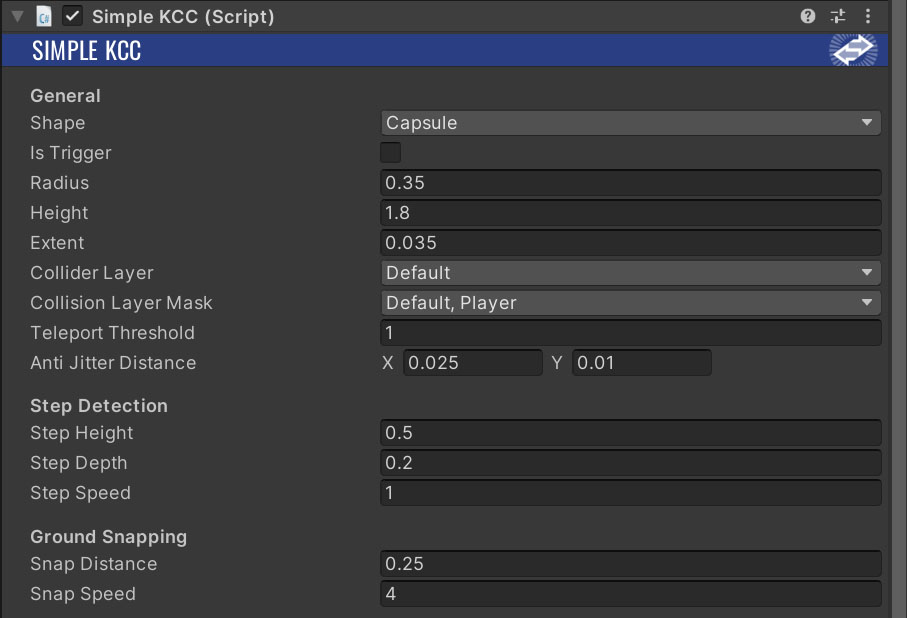
The parameters are:
Shape
- Defines character physics behavior.None
- Skips internal physics query, collider is despawned.Capsule
- Full physics processing, Capsule collider spawned.
Is Trigger
- The collider is non-blocking if enabled.Radius
- Collider radius.Height
- Collider height.Extent
- Defines additional radius extent for ground detection and and other features. Recommended range is 1-2% of radius.Collider Layer
- Layer of collider game object.Collision Layer Mask
- Layer mask the character collides with.Teleport Threshold
- Defines minimum distance the character must move in a single tick to treat the movement as instant (teleport).Anti Jitter Distance
- Defines position distance tolerance to smooth out jitter. Higher values may introduce noticeable delay when switching move direction.X
- Horizontal axis.Y
- Vertical axis.
Step Height
- Maximum obstacle height to step on it.Step Depth
- Maximum depth of the step check.Step Speed
- Multiplier of unapplied movement projected to step up. This helps traversing obstacles faster.Snap Distance
- Maximum ground check distance for snapping.Snap Speed
- Ground snapping speed per second.
Movement
- Initialize KCC properties.
C#
public override void Spawned()
{
// Set custom gravity.
_simpleKCC.SetGravity(Physics.gravity * 4.0f);
}
- Movement and look of the character.
C#
public override void FixedUpdateNetwork()
{
if (Runner.TryGetInputForPlayer(Object.InputAuthority, out GameplayInput input) == true)
{
// Apply look rotation delta. This propagates to Transform component immediately.
_simpleKCC.AddLookRotation(input.LookRotationDelta);
// Set default world space velocity and jump impulse.
Vector3 moveVelocity = _simpleKCC.TransformRotation * new Vector3(input.MoveDirection.x, 0.0f, input.MoveDirection.y) * 10.0f;
Vector3 jumpImpulse = default;
if (input.Jump == true && _simpleKCC.IsGrounded == true)
{
// Set world space jump vector.
jumpImpulse = Vector3.up * 10.0f;
}
_simpleKCC.Move(moveVelocity, jumpImpulse);
}
}
- Camera synchronization.
C#
public void LateUpdate()
{
// Only InputAuthority needs to update camera.
if (Object == null || Object.HasInputAuthority == false)
return;
// Update camera pivot and transfer properties from camera handle to Main Camera.
// LateUpdate() is called after all Render() calls - the character is already interpolated.
Vector2 pitchRotation = _simpleKCC.GetLookRotation(true, false);
_cameraPivot.localRotation = Quaternion.Euler(pitchRotation);
Camera.main.transform.SetPositionAndRotation(_cameraHandle.position, _cameraHandle.rotation);
}
API Overview
Properties
bool IsActive
- Controls execution of the KCC.Transform Transform
- Reference to cachedTransform
component.Rigidbody Rigidbody
- Reference to attachedRigidbody
component.CapsuleCollider Collider
- Reference to KCC collider. Can be null ifShape
is set toEKCCShape.None
.KCCSettings Settings
- Basic KCC settings.Vector3 Position
- Calculated position which is propagated toTransform
.Quaternion LookRotation
- Combination of lookPitch
andYaw
.Vector3 LookDirection
- Look direction based on look rotation.Quaternion TransformRotation
- Transform rotation based onYaw
look rotation.Vector3 TransformDirection
- Transform direction based on transform rotation.float RealSpeed
- Speed calculated from real position change.Vector3 RealVelocity
- Velocity calculated from real position change.bool HasJumped
- Flag that indicates KCC has jumped in current tick.bool IsGrounded
- Flag that indicates KCC is touching a collider with normal angle lower thanMaxGroundAngle
.Vector3 GroundNormal
- Combined normal of all touching colliders. Normals less distant from up direction have bigger impacton final normal.Func<KCC, Collider, bool> ResolveCollision
- Custom collision resolver callback. Use this to apply extra filtering.
Methods
void SetActive(bool isActive)
- Controls execution of the KCC.void SetPosition(Vector3 position)
- Set position of the KCC and immediately synchronizeTransform
.void SetGravity(Vector3 gravity)
- Set gravity vector.void SetHeight(float height)
- UpdateHeight
inSettings
and immediately synchronize withCollider
.void SetRadius(float radius)
- UpdateRadius
inSettings
and immediately synchronize withCollider
.void SetShape(EKCCShape shape, float radius = 0.0f, float height = 0.0f)
- UpdateShape
,Radius
(optional),Height
(optional) inSettings
and immediately synchronize withCollider
.void SetTrigger(bool isTrigger)
- UpdateIs Trigger
flag inSettings
and immediately synchronize with Collider.void SetColliderLayer(int layer)
- UpdateCollider Layer
inSettings
and immediately synchronize with Collider.void SetCollisionLayerMask(LayerMask layerMask)
- UpdateCollision Layer Mask
inSettings
.void SetMaxGroundAngle(float maxGroundAngle)
- Set maximum walkable ground angle (in degrees).void RefreshChildColliders()
- Refresh child colliders list, used for collision filtering. Child colliders are ignored completely, triggers are treated as valid collision.Vector2 GetLookRotation(bool pitch = true, bool yaw = true)
- Returns current look rotation.void SetLookRotation(float pitch, float yaw)
- Set pitch and yaw look rotation. Values are clamped to <-90, 90> (pitch) and <-180, 180> (yaw).void SetLookRotation(Vector2 lookRotation)
- Set pitch and yaw look rotation. Values are clamped to <-90, 90> (pitch) and <-180, 180> (yaw).void SetLookRotation(Quaternion lookRotation, bool preservePitch = false, bool preserveYaw = false)
- Set pitch and yaw look rotation. Roll is ignored (not supported). Values are clamped to <-90, 90> (pitch) and <-180, 180> (yaw).void AddLookRotation(float pitchDelta, float yawDelta)
- Add pitch and yaw look rotation. Resulting values are clamped to <-90, 90> (pitch) and <-180, 180> (yaw).void AddLookRotation(float pitchDelta, float yawDelta, float minPitch, float maxPitch)
- Add pitch and yaw look rotation. Resulting values are clamped to <minPitch, maxPitch> (pitch) and <-180, 180> (yaw).void AddLookRotation(Vector2 lookRotationDelta)
- Add pitch (x) and yaw (y) look rotation. Resulting values are clamped to <-90, 90> (pitch) and <-180, 180> (yaw).void AddLookRotation(Vector2 lookRotationDelta, float minPitch, float maxPitch)
- Add pitch (x) and yaw (y) look rotation. Resulting values are clamped to <minPitch, maxPitch> (pitch) and <-180, 180> (yaw).void Move(Vector3 kinematicVelocity = default, Vector3 jumpImpulse = default)
- Move function. The KCC can move with zero velocity - depenetration from overlapping geometry, gravity, ...void Log(params object[] messages)
- Logs info message into console with frame/tick metadata.
Advanced KCC
If you've enjoyed the simplicity and ease-of-use of Simple KCC but find yourself yearning for more functionalities and customization options, feel free to explore Advanced KCC. The Advanced KCC is an unconstrained version of Simple KCC and provides precise control over the character movement and many powerful features.
💡 It also contains some general topics worth checking, for example Input Smoothing and Debugging.
Back to top