Interactions

Overview
Interaction objects are scene objects with which the player can interact. The interaction process is triggered when the player aims at an object that has a collider with the Interaction
layer and contains a component that implements the IInteraction
interface.
Item Boxes
Item Boxes are scattered around the map to provide players with weapons, grenades, ammo, shield packs, health packs and jetpack fuel. Items in the box are regular pickups that are spawned when the box is opened by the player. Items are randomly selected based on probabilities specified in the item box prefab. The box is automatically closed after some time and unavailable for further opening until the cooldown expires.
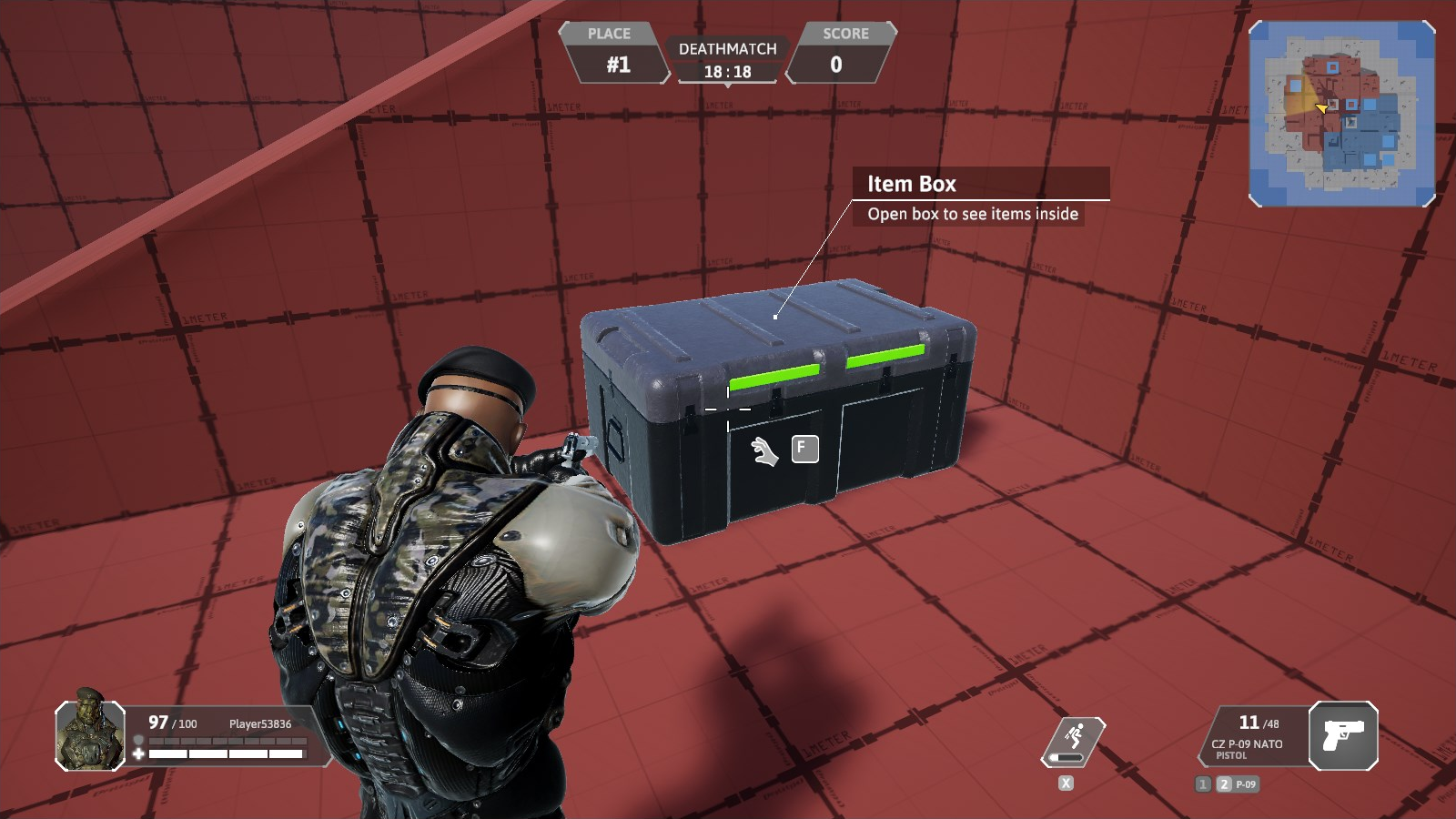
Pickups
Items that can be picked up with an interaction action or are picked up automatically when the player presses the pickup button. In the current version of Fusion BR, there are two types of pickups. Pickups in the item boxes and pickups that are dropped by players (weapons, grenades).
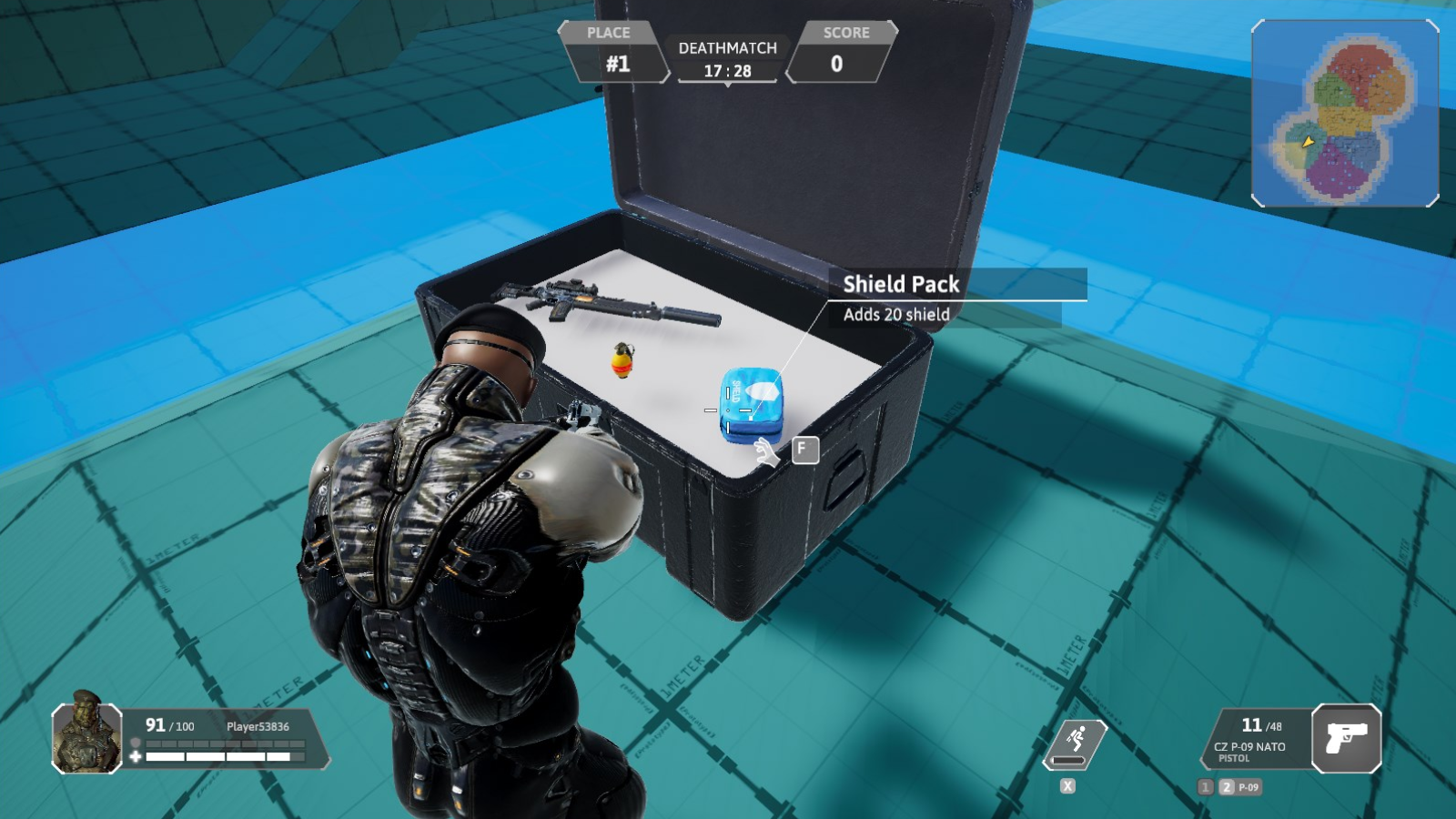
Weapon Drops
In Fusion BR players can drop their weapons to the ground. The drop is initiated either by holding the interaction key (F), picking up a weapon that matches an already occupied slot or when the player dies, all the weapons of the player are dropped.
When the drop is initiated, a special DynamicPickup
object is spawned. DynamicPickup
acts as a wrapper for any object that the player can drop. It contains the essential setup for the drop (specifically a NetworkRigidbody
component). Since every weapon in Fusion BR is a separate network object, the entire weapon GameObject is parented to the DynamicPickup
and tossed out to the world. This ensures that when another player picks up the weapon, it retains the properties from its previous owner such as visuals, ammo, or any other weapon state.
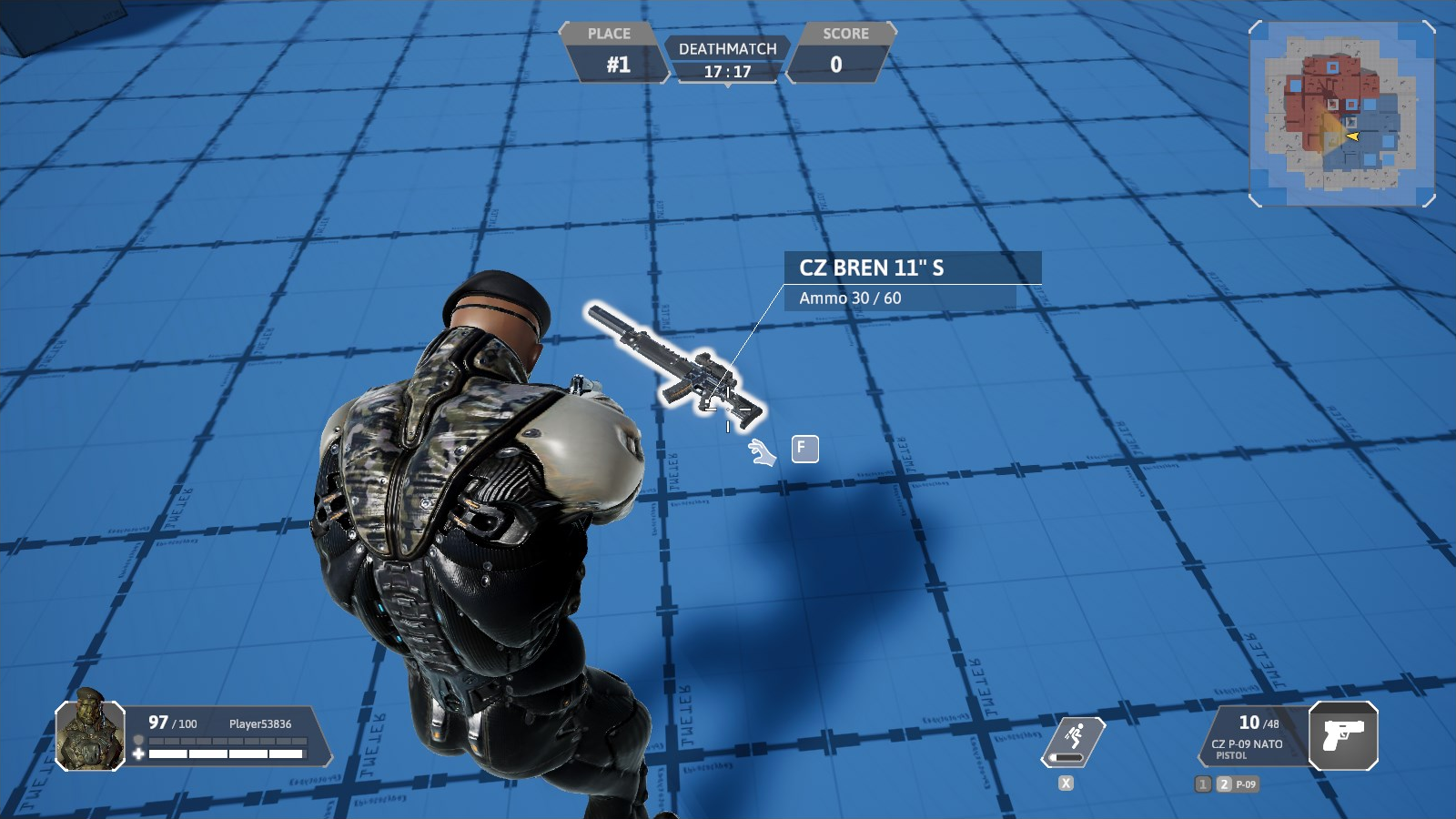
Weapon provides to DynamicPickup
essential information through the IDynamicPickupProvider
interface. For example the name and description that will be used for the interaction UI or the weapon collider that is enabled before the drop to ensure correct rigidbody behavior.
C#
public interface IDynamicPickupProvider
{
public string Name { get; }
public string Description { get; }
public Transform InterpolationTarget { get; }
public Collider Collider { get; }
public float DespawnTime { get; }
}
When the new owner picks up the weapon, it simply unpacks the weapon object from the DynamicPickup
, adds the weapon into his weapons list, and assigns his input authority to the weapon.
Footsteps
Footstep sounds are handled by the AgentFootsteps
component and are completely calculated (there is no support for animation events in the tick accurate animations addon). Foot transform position changes are simply checked to detect when the foot actually touched the ground. When a touch is detected, a small raycast is fired to get information about the surface. The Unity Tag assigned to the surface collider gameobject is used to differentiate between different surface types.
C#
public class FootstepSetup : ScriptableObject
{
[SerializeField]
private AudioSetup _fallbackWalkSound;
[SerializeField]
private AudioSetup _fallbackRunSound;
[SerializeField]
private FootstepData[] _footsteps;
[Serializable]
private class FootstepData
{
public string Tag;
public AudioSetup SoundWalk;
public AudioSetup SoundRun;
}
}
Every agent can have an assigned footstep setup that specifies what sound should be played for each surface. The footstep setup also contains variants for a walk or a run, so different sounds or different volumes can be achieved based on the movement speed.