Execution Control
Overview
SimulationBehaviour
and NetworkBehaviour
derived components expose properties which can be used for conditional execution of code.
The SimulationBehaviourAttribute
may also be used to conditionally disable the FixedUpdateNetwork()
callback entirely for a particular Simulation Loop (Stage) or peer type (Mode).
Network Runner Conditionals
Simulation Loop Reference
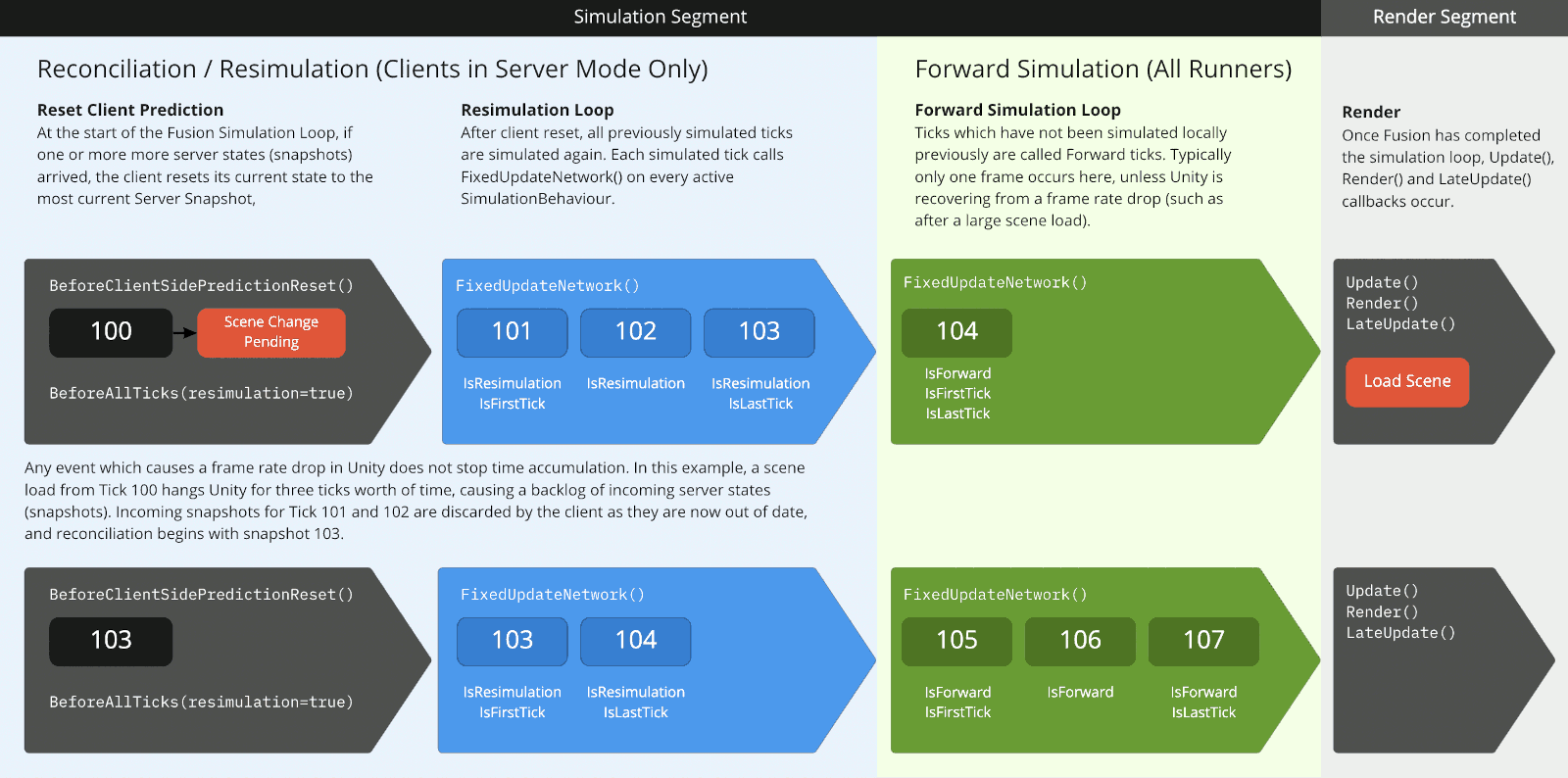
Simulation Stage
These properties are only valid inside of the FixedUpdateNetwork()
callback, and can be used to get information about the current tick being simulated in relation to the entire Simulation Loop.
Runner.IsResimulation
: Current tick has been simulated previously and is being simulated again now as part of client prediction reconciliation.Runner.IsForward
: Current tick is being simulated for the first time.Runner.IsFirstTick
: Current tick is the first tick of the Resimulation or Forward Loop.Runner.IsLastTick
: Current tick is the last tick of the Resimulation or Forward Loop.
C#
public override void FixedUpdateNetwork()
{
if(Runner.IsResimulation)
{
// execute code only if this tick has already been simulated before
}
if(Runner.IsForward && Runner.IsFirstTick)
{
// execute code only if this is the first tick of the forward simulation loop
}
}
Note: IsFirstTick
and IsLastTick
may be true twice during Fusion’s Simulation Loop; once during the Resimulation Loop and once during the Forward Loop. If resimulation is possible (Clients in Server Mode), these properties must be used in conjunction with Runner.IsForward
or Runner.IsResimulation
to determine which loop.
Note: It is possible for a simulated tick to be both IsFirstTick
and IsLastTick
if there is only one tick being processed in the loop. This typically is the case for the Forward Simulation Loop.
Simulation Mode (Peer Type)
These properties are valid in all timing segments after Spawned() has been called.
Runner.IsServer
: Runner represents a Server peer of any type (Dedicated, Host, or a Shared Mode plug-in).Runner.IsHost
: Runner represents the Server peer with a Local Player.Runner.IsClient
: Runner represents a Client peer with a Local Player.Runner.IsPlayer
: Runner represents a Player in the GameSession. True for Host and Client, False for non-Host ServersRunner.IsSharedModeMasterClient
- (Only valid in Shared Mode) Runner is flagged as the Master Client.
C#
public override void FixedUpdateNetwork()
{
if(Runner.IsServer)
{
// Execute code only on the game server
}
}
SimulationBehaviourAttribute
An alternative to testing the above properties inside of callbacks, it is possible to conditionally exclude entire SimulationBehaviour
classes from executing FixedUpdateNetwork()
with the [SimulationBehaviour]
attribute.
[SimulationBehaviour(Stages= )]
: Supply aSimulationStages
enum flag to specify ifFixedUpdateNetwork()
is to be called during the Resimulation Loop or Forward Loop. Default value of 0 is treated asStages = SimulationStages.Resimulation | SimulationStages.Forward
.SimulationBehaviour(Modes= )]
: Supply aSimulationModes
enum flag to specify ifFixedUpdateNetwork()
should only be called on specific peer types. Default value of 0 is treated as all flags set.
C#
[SimulationBehaviour(
Stages = SimulationStages.Forward,
Modes = SimulationModes.Server | SimulationModes.Host
)]
public class TestNetworkBehaviour : NetworkBehaviour, IBeforeAllTicks
{
public override FixedUpdateNetwork()
{
// This will only execute if Runner.IsForward and if Runner.IsServer
}
}
Note: SimulationModes
is an enum flag, and Server and Host are treated as distinct. To include ALL server types, union the two (SimulationModes.Server | SimulationModes.Host
).
Connection Type
These members return ConnectionType
(Relayed, Direct, None), which indicates if a client is connected directly to the server, or if messages are being relayed through the Photon Cloud.
Runner.CurrentConnectionType
: IfRunner
is a Client, returns the type of connection to the Server. Will returnConnectionType.None
ifRunner
is not a Client.Runner.GetPlayerConnectionType(PlayerRef player)
: IfRunner
is the Server, this returns the type of connection a Client (identified by its PlayerRef) has to this Runner. Will returnConnectionType.None
ifRunner
is not the Server.
C#
public override void Spawned()
{
if(Runner.CurrentConnectionType == ConnectionType.Relayed)
{
// Special handling for cases where messages to and from
// this peer are being relayed through the Photon server.
}
}
Game Mode (Topology)
The Runner.GameMode
value can be used to selectively execute code based on the Game Session topology. In most cases this is not needed as the other conditionals described on this page are much more appropriate (such as Runner.IsServer
or Runner.IsClient
). There are some cases though where you may want specialized handling for particular game modes, such as Single Player.
C#
if(Runner.GameMode == GameMode.Single)
{
// Execute code only if in running as single player
// Note that this value can be cached,
// as it cannot change once the Runner has been started.
}
if(Runner.GameMode == GameMode.Shared)
{
// Execute code only if running in shared mode.
// Typically this is only useful for testing,
// if you have code designed to work in both Server and Shared Modes.
}
Network Object Conditionals
Object Authority
Object.HasStateAuthority
: The associatedObject.Runner
has State Authority over thisNetworkObject
.Object.HasInputAuthority
: The associatedObject.Runner
has Input Authority over thisNetworkObject
.Object.IsProxy
: The associated NetworkRunner has neither Input Authority nor State Authority over thisNetworkObject
. (Inputs for this object are not available on this peer).
C#
if(Object.HasStateAuthority)
{
// Execute only if the associated Runner has State Authority over this object
}
if(Object.HasInputAuthority)
{
// Execute only if the associated Runner has Input Authority over this object
}
if(Object.IsProxy)
{
// Execute only if the associated Runner has neither State Authority nor Input Authority over this object
}
Note: These properties are only valid if the associated NetworkObject
is valid, as authority is per NetworkObject
. If the Object is not valid, these properties will all return false without throwing exceptions or log warnings. In use cases where it is possible for the NetworkObject
to be invalid and this needs to be accounted for, use Object.IsValid
.
Other Object Conditionals
Object.IsValid
: The associatedNetworkObject
is not null, and is attached to an activeNetworkRunner
.Object.IsInSimulation
: The associatedNetworkObject
is currently participating in its associated Simulation.Object.IsSceneObject
: The associatedNetworkObject
was attached to the network state as part of a scene, rather than having been created with aNetworkRunner.Spawn()
operation.