Getting Started
Available in the Gaming Circle and Industries Circle

Creating character with KCC prefab variant
The easiest way to create custom character is to create a prefab variant from default KCC
prefab:
- Right click on
KCC
prefab (inAssets/Photon/QuantumAddons/KCC/AssetDB/Entities
). - Select
Create > Prefab Variant
.

- Add your own visual, add custom components.
- The character is ready for use. Continue with Moving the character
Creating character from scratch
- Create a new player prefab.
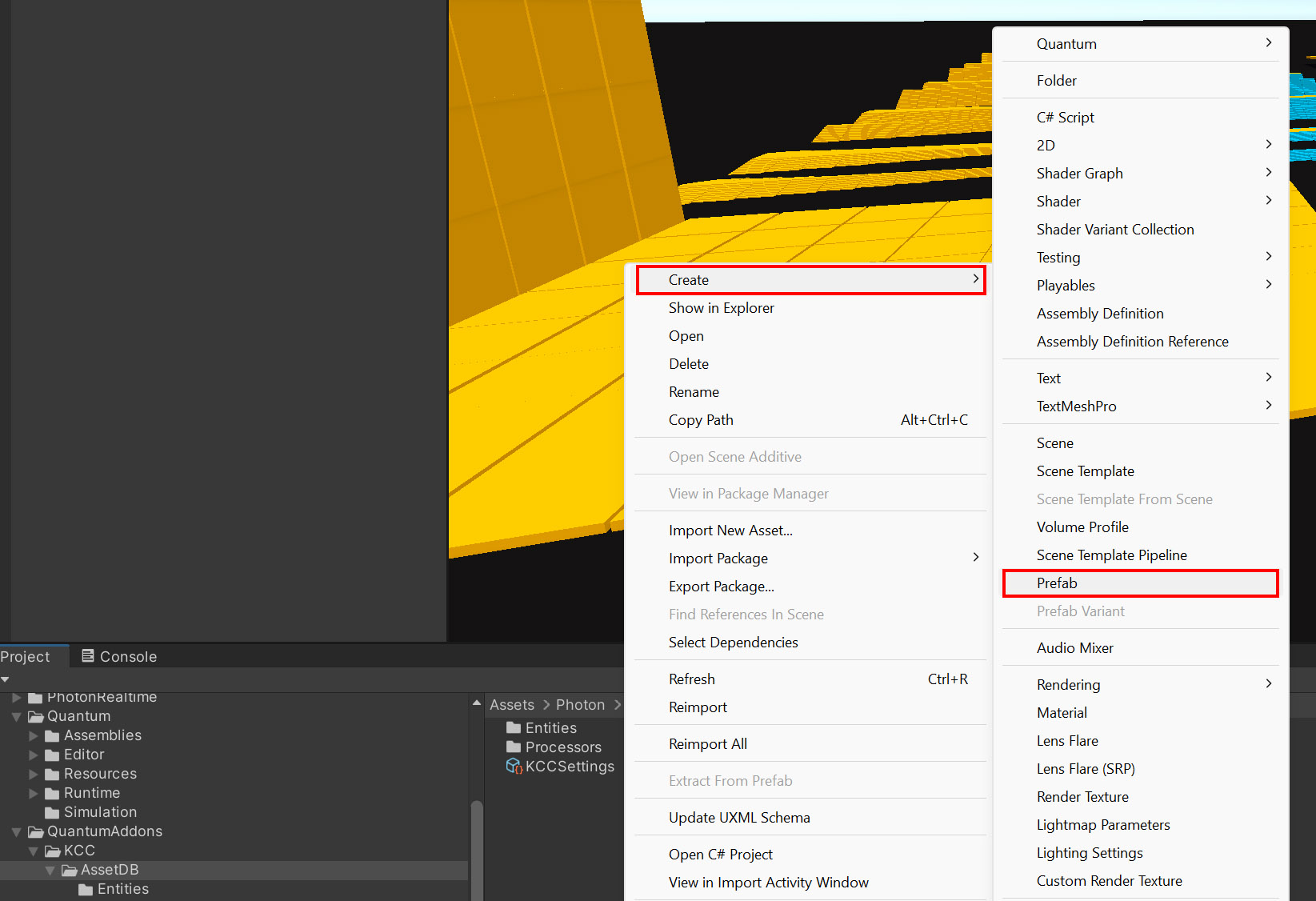
- Add
Quantum Entity View
andQ Prototype KCC
components to the root game object. Optionally addCapsule Collider
.
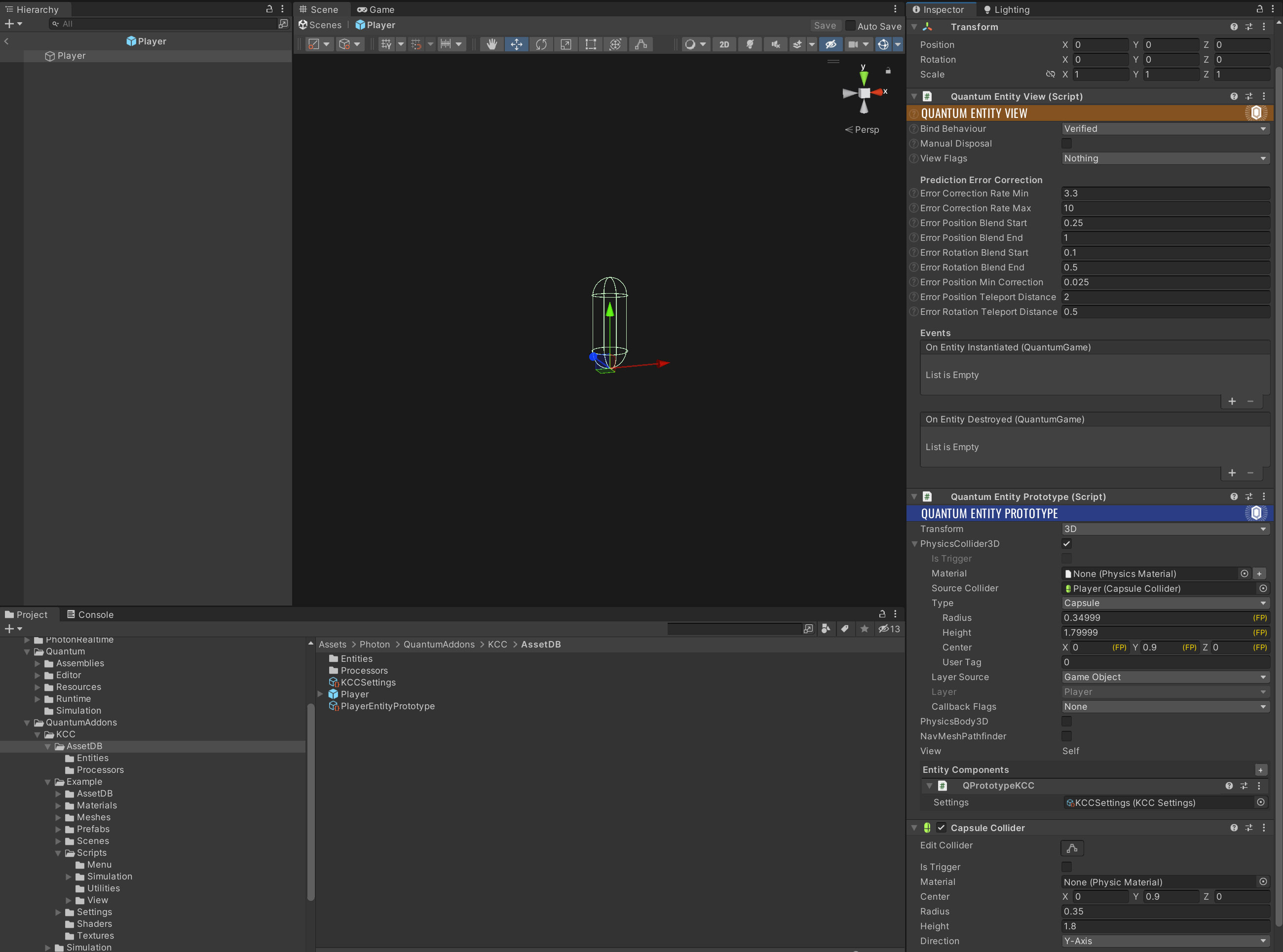
- Configure the game object
- Set game object layer (optional).
- On
Quantum Entity View
setBind Behaviour
toVerified
. - On
Quantum Entity Prototype
setTransform
to3D
. - Enable
PhysicsCollider3D
and link previously createdCapsule Collider
toSourceCollider
(optional). - On
Q Prototype KCC
set reference to aKCC Settings
asset.
- The character is ready for use. Continue with Moving the character
Configuring KCC behavior
The KCC addon contains some pre-configured assets.
- Select
Assets/Photon/QuantumAddons/KCC/AssetDB/KCCSettings.asset
to configure defaults (radius, height, collision layer mask). These should match values in capsule collider created above.
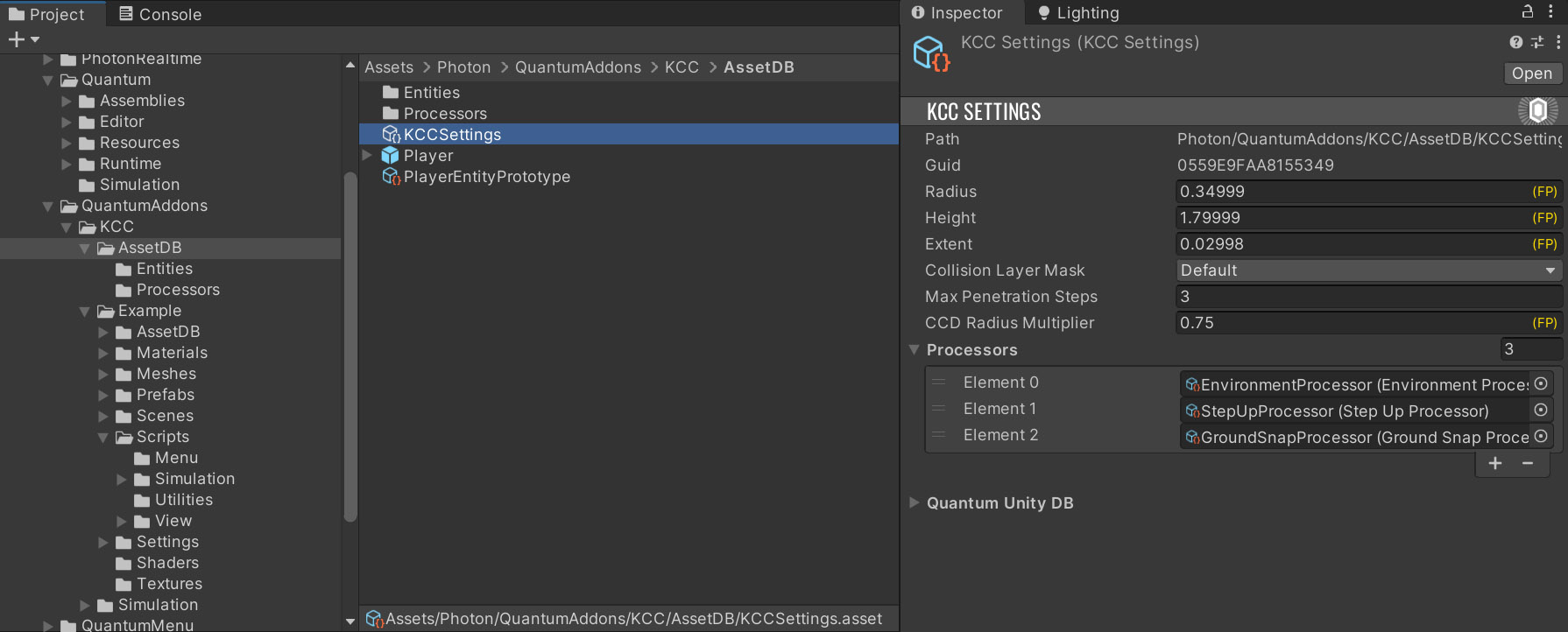
- Link KCC processors if you are creating new
KCC Settings
asset. These are responsible for actual movement logic, more info can be found in Processors section. Default processors are located inAssets/Photon/QuantumAddons/KCC/AssetDB/Processors
.
Moving the character
The movement is processed in KCC
component update. This is managed by KCC System
, therefore it must be added to your Systems Config
.
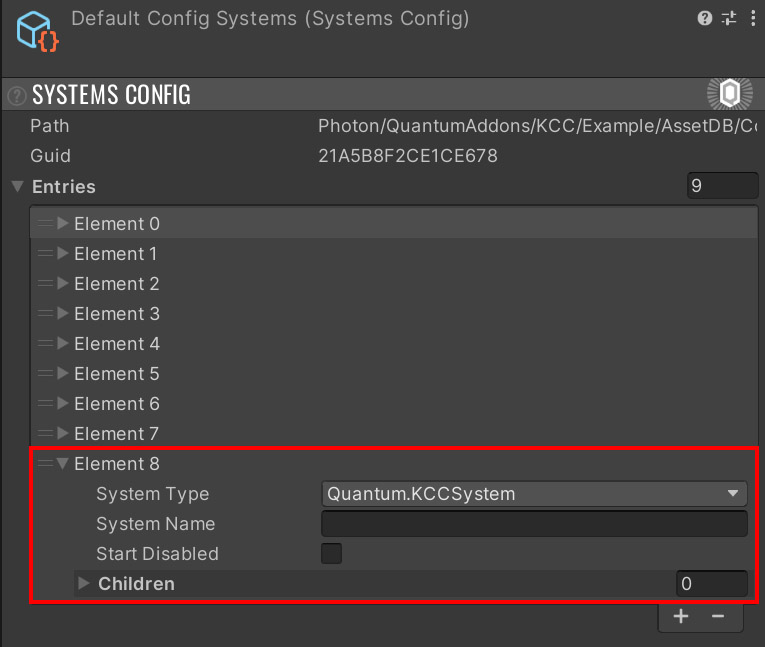
Following code example sets character look rotation and input direction for KCC
which is later processed by EnvironmentProcessor
(or your own processor).
C#
public unsafe class PlayerSystem : SystemMainThreadFilter<PlayerSystem.Filter>
{
public struct Filter
{
public EntityRef Entity;
public Player* Player;
public KCC* KCC;
}
public override void Update(Frame frame, ref Filter filter)
{
Player* player = filter.Player;
if (player->PlayerRef.IsValid == false)
return;
KCC* kcc = filter.KCC;
Input* input = frame.GetPlayerInput(player->PlayerRef);
kcc->AddLookRotation(input->LookRotationDelta.X, input->LookRotationDelta.Y);
kcc->SetInputDirection(kcc->Data.TransformRotation * input->MoveDirection.XOY);
if (input->Jump.WasPressed == true && kcc->IsGrounded == true)
{
kcc->Jump(FPVector3.Up * player->JumpForce);
}
}
}
It is also possible to skip processors functionality completely and simply set the velocity using kcc->SetKinematicVelocity();
.
More movement code examples can be found in Sample Project.
Back to top