Input Smoothing
To get perfectly smooth movement and camera, it is mandatory to apply input signal correctly. Following sections focus on camera look rotation based on data get from mouse.
Getting Input
The mouse delta is obtained using:
UnityEngine.Input.GetAxis("Mouse X")
UnityEngine.Input.GetAxisRaw("Mouse X")
UnityEngine.InputSystem.Mouse.current.delta.ReadValue()
All methods above return position delta polled from mouse without any post-processing. Depending on mouse polling rate (common is 125Hz, gaming mouses usually 1000Hz), you get these values for Mouse X
when moving with the mouse:
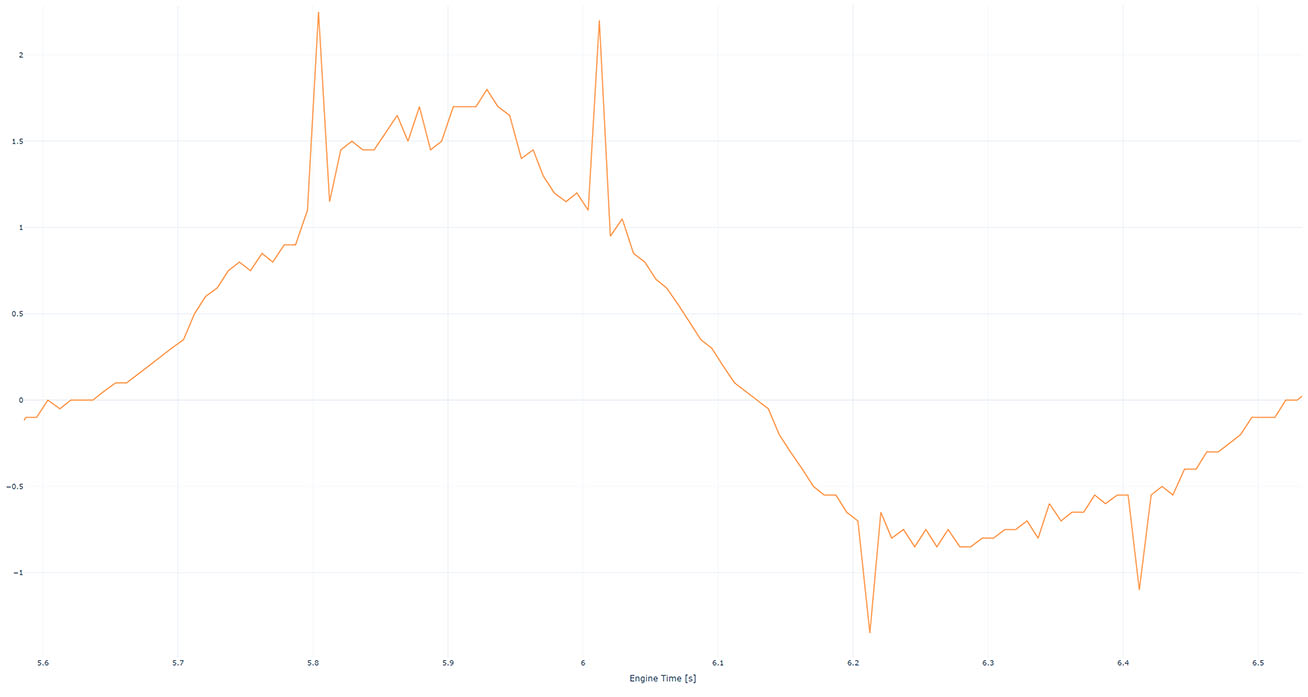
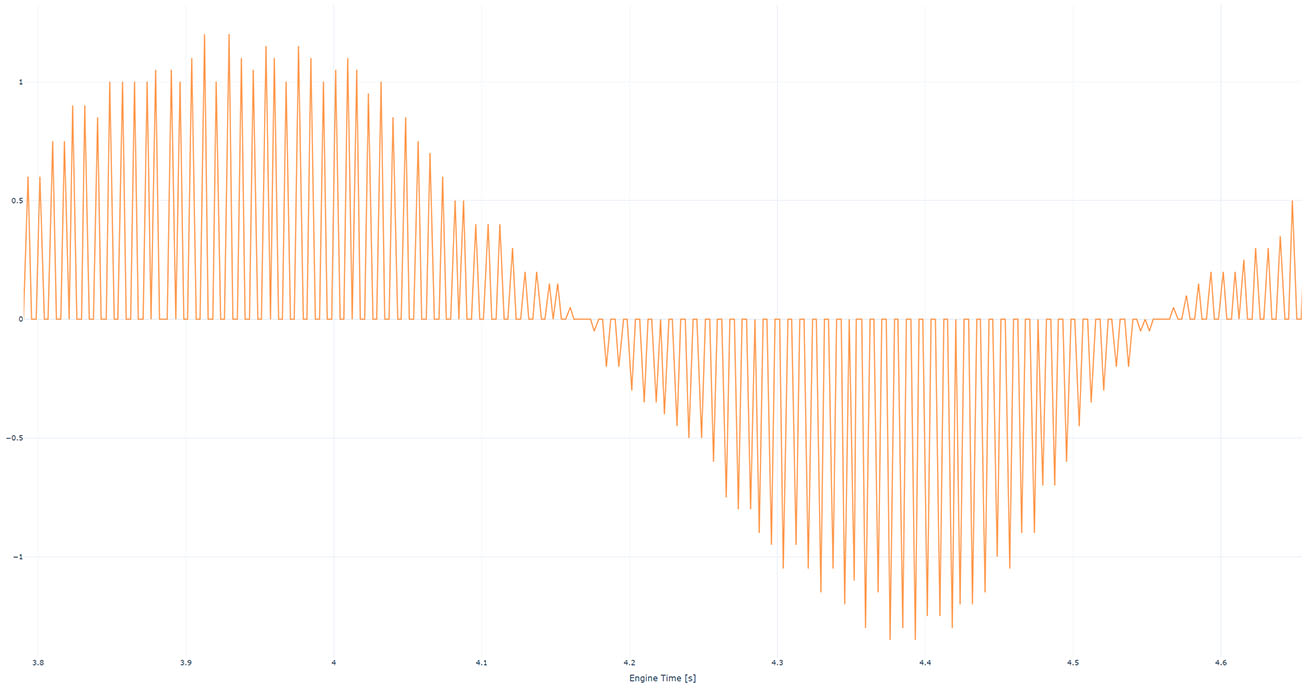
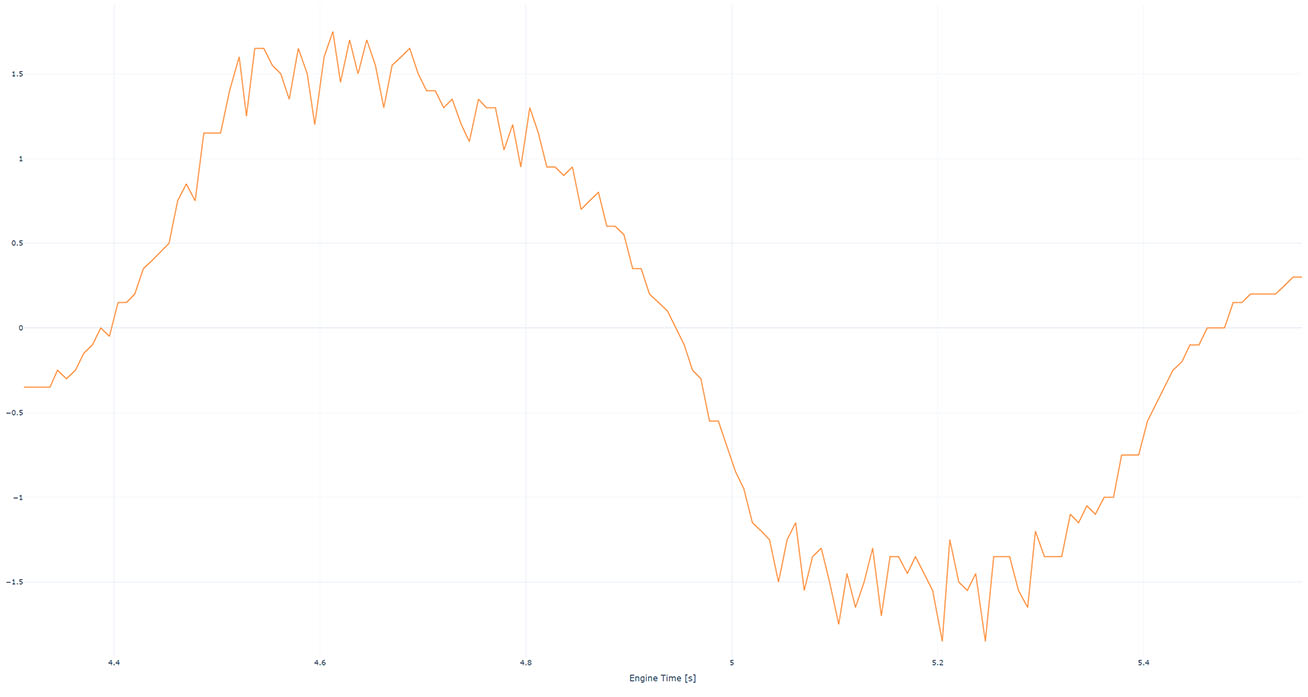
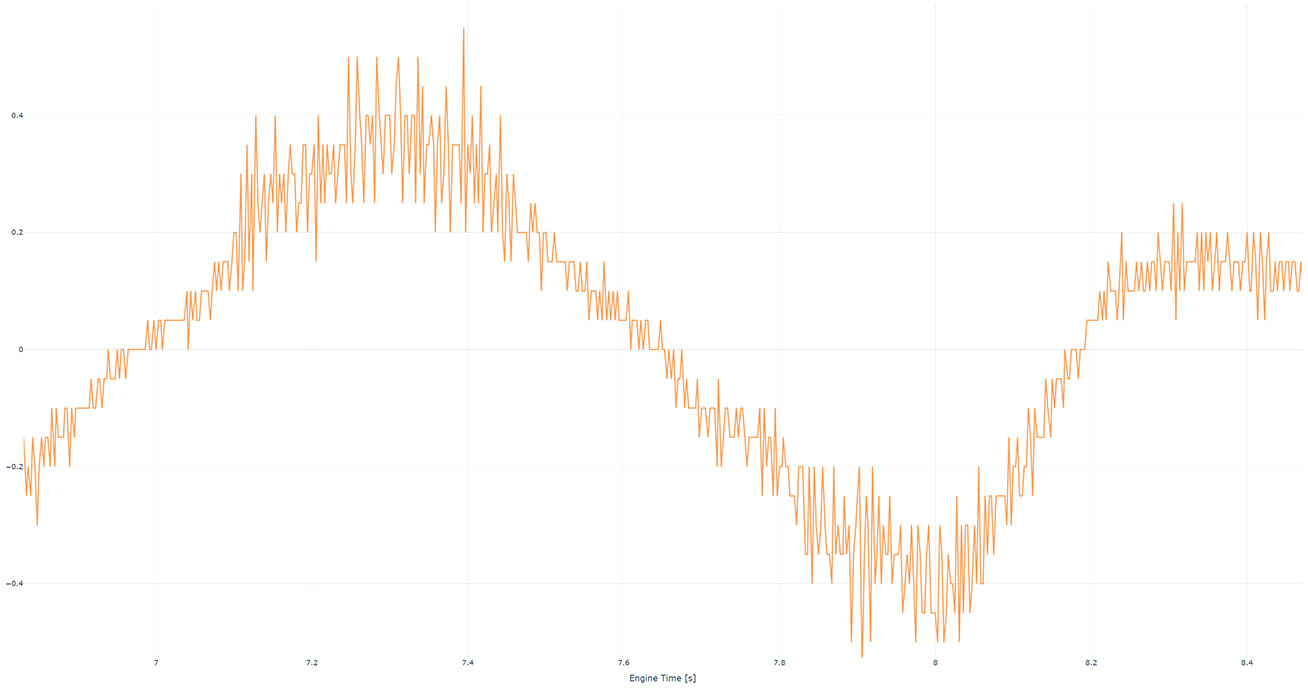
As you can see, each case produces different results. In almost all cases propagation directly to camera rotation results in looking not being smooth. You can test it directly in Sample Project.
Smoothing
To get perfectly smooth look, there are 2 possible solutions:
- Mouse polling, engine update rate and monitor refresh rate must be aligned - for example 360Hz mouse polling, 360 FPS engine update rate, 360Hz monitor refresh rate. Not realistic solution.
- Input smoothing - gives almost perfectly smooth results (the difference is noticeable also on high-end gaming setup) at the cost of increased input lag by few milliseconds.
The KCC provides an utility script SmoothValue
and its variants, SmoothFloat
, SmoothVector2
, SmoothVector3
.
Following code shows usage of SmoothVector2
to calculate smooth mouse position delta from values in last 10 milliseconds.
C#
public class PlayerInput : MonoBehaviour
{
// Creates a SmoothVector2 with 128 samples.
private SmoothVector2 _mouseDeltaValues = new SmoothVector2(128);
private void Update()
{
// Read mouse position delta.
Vector2 mouseDelta = Mouse.current.delta.ReadValue();
// Add new value for current frame with delta time since last frame.
_mouseDeltaValues.AddValue(Time.frameCount, Time.unscaledDeltaTime, mouseDelta);
// Calculate smooth mouse delta based on values in last 10 milliseconds.
Vector2 smoothMouseDelta = _mouseDeltaValues.CalculateSmoothValue(0.01, Time.unscaledDeltaTime);
}
}
Following images show mouse delta being propagated to accumulated look rotation (roughly 90°) with various mouse polling rates, engine update rates and smoothing windows:
Purple
- No smoothing applied.Cyan
- 10ms smoothing window.Green
- 20ms smoothing window.Yellow
- 30ms smoothing window.Blue
- 40ms smoothing window.
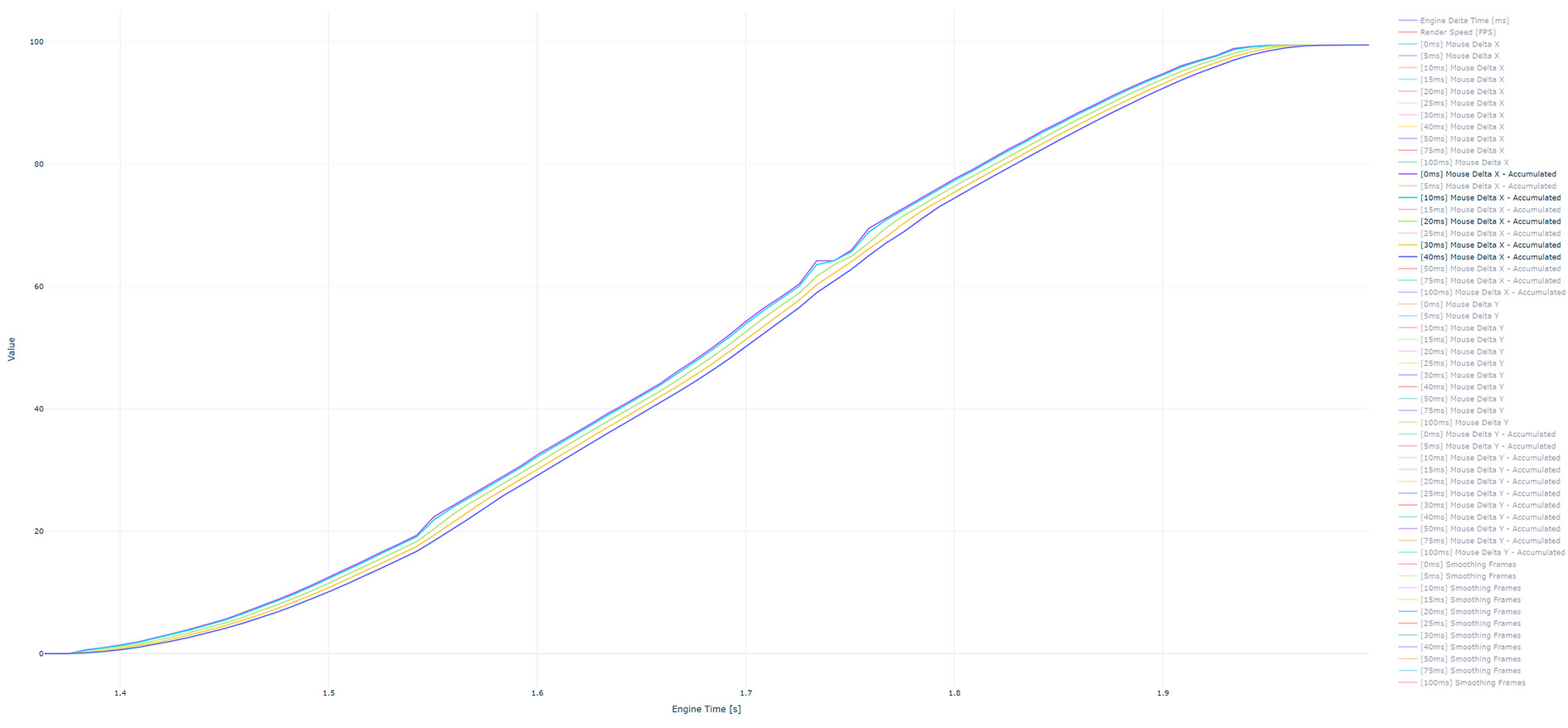
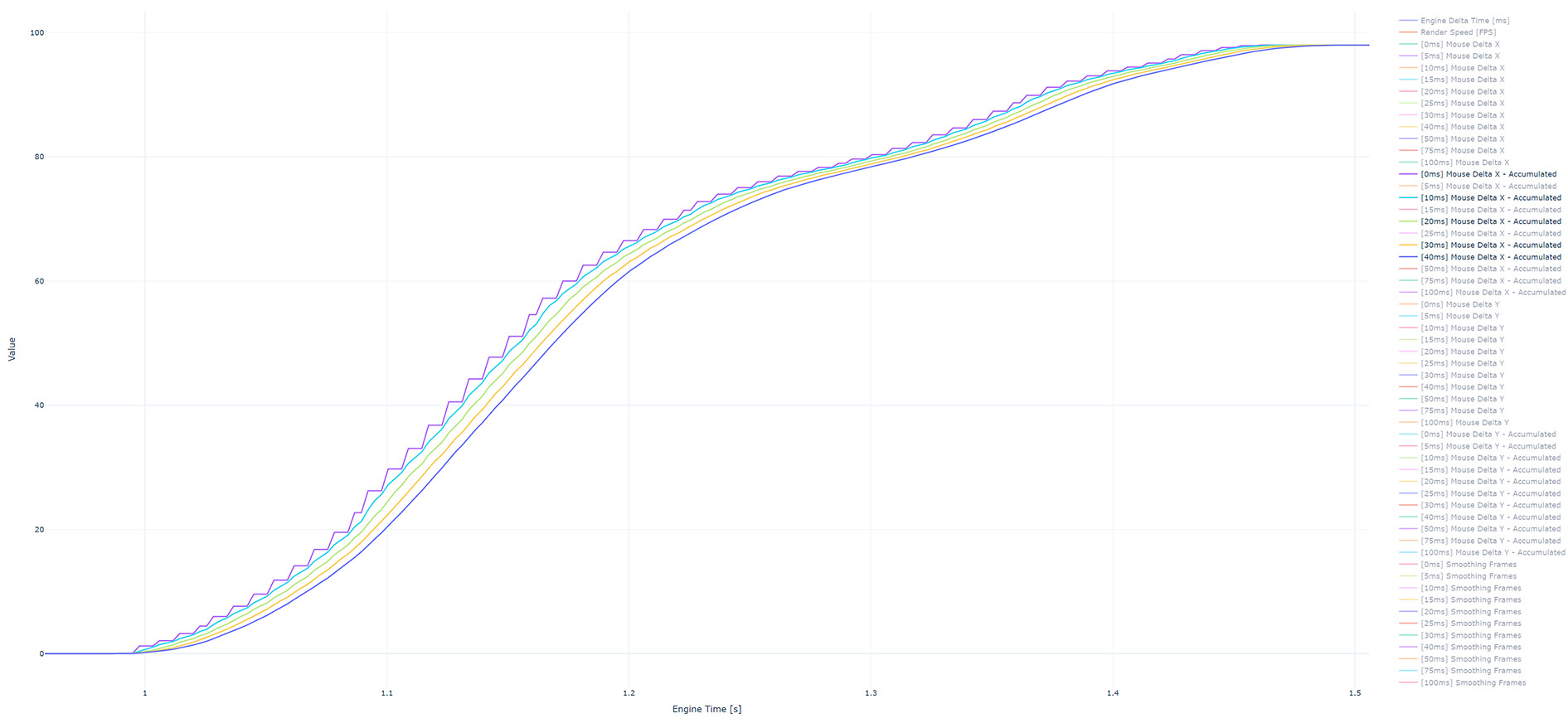
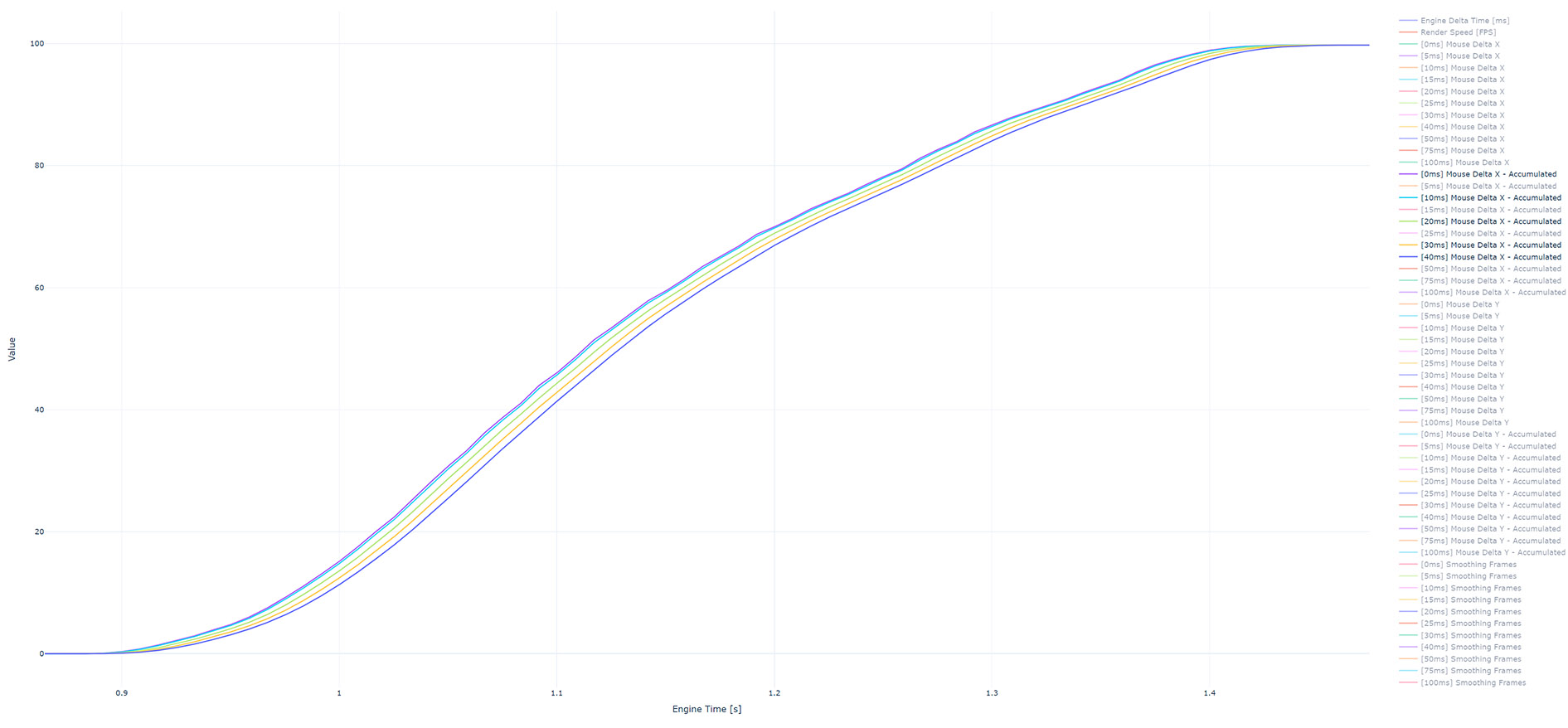
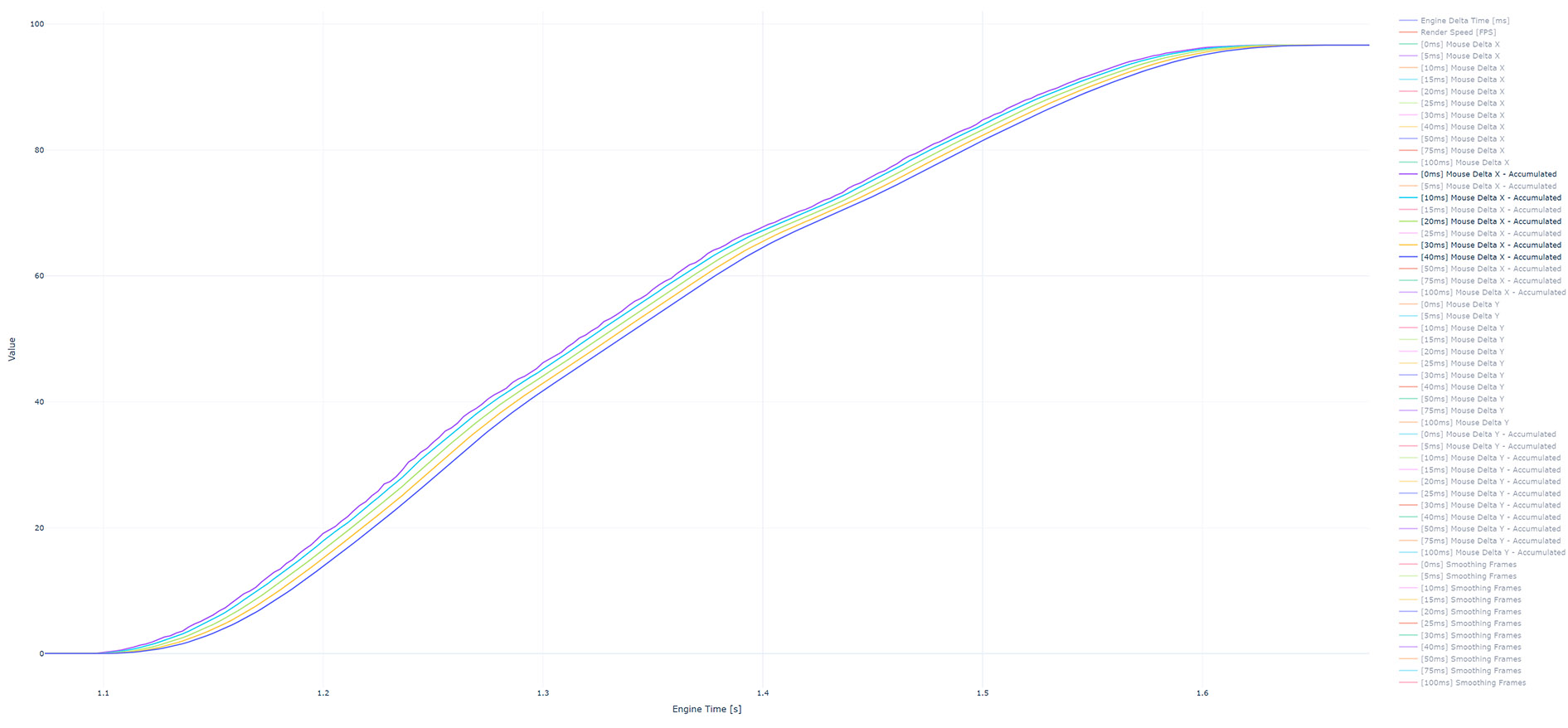
Generally for regular users with 125Hz mouse a 10-20ms smoothing window is a good balance between gain in smoothness and loss in responsivity.
For users with proper gaming hardware (500+Hz mouse, 120+Hz monitor) reaching high engine update rates 3-5ms smoothing window is recommended + option to disable smoothing completely.
Following image shows a detail of look rotation after accumulation of 90°.
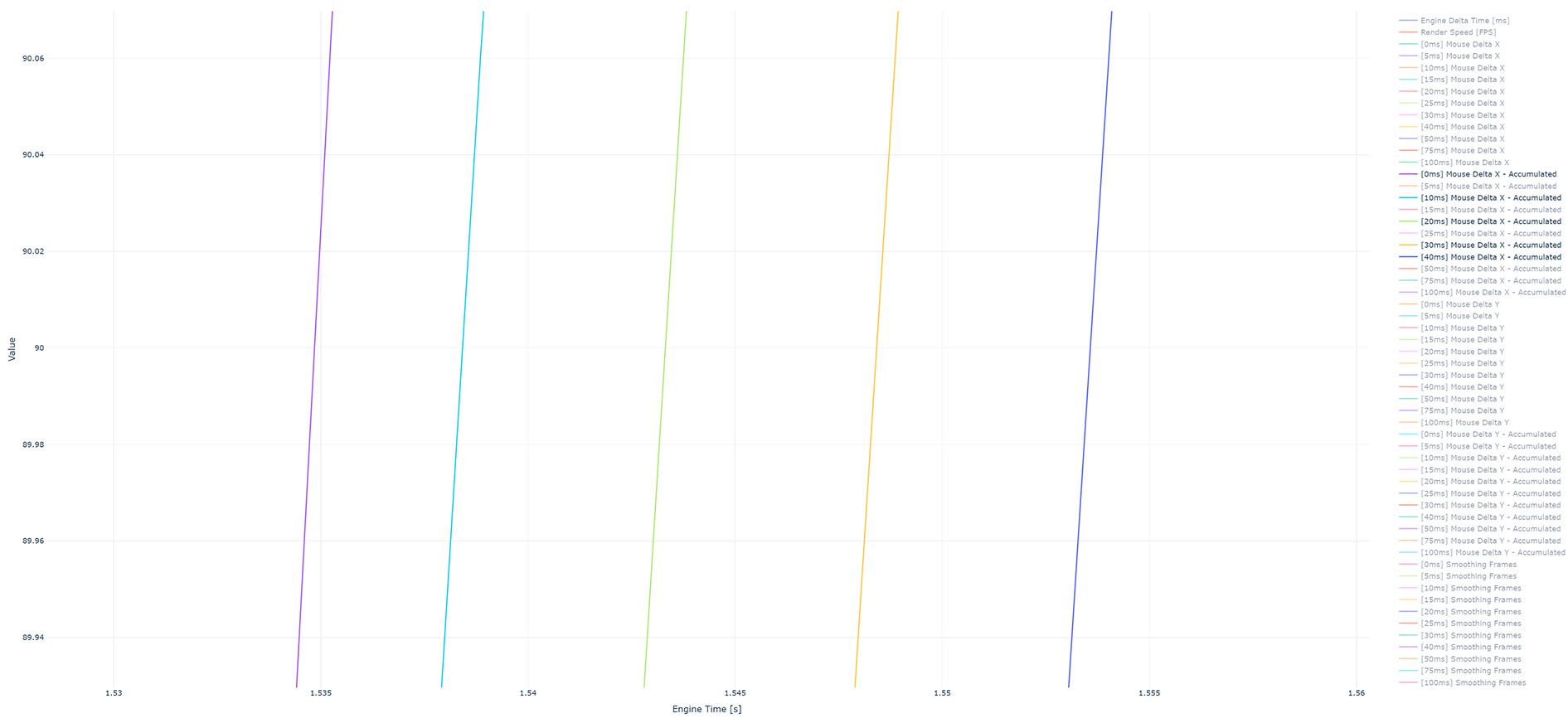
You can see that applying smoothing adds 30-50% of smoothing window length to input lag (+3.6ms input lag using 10ms smoothing window).
⚠️ All graphs were created from Sample Project using InputSmoothing
scene and Recorders.