Karts

概述
Fusion Kart 範例展示了如何使用伺服器權威、客戶端已預測模型製作賽車遊戲的方法,玩家可以使用名稱作為房間識別碼來建立和加入房間。
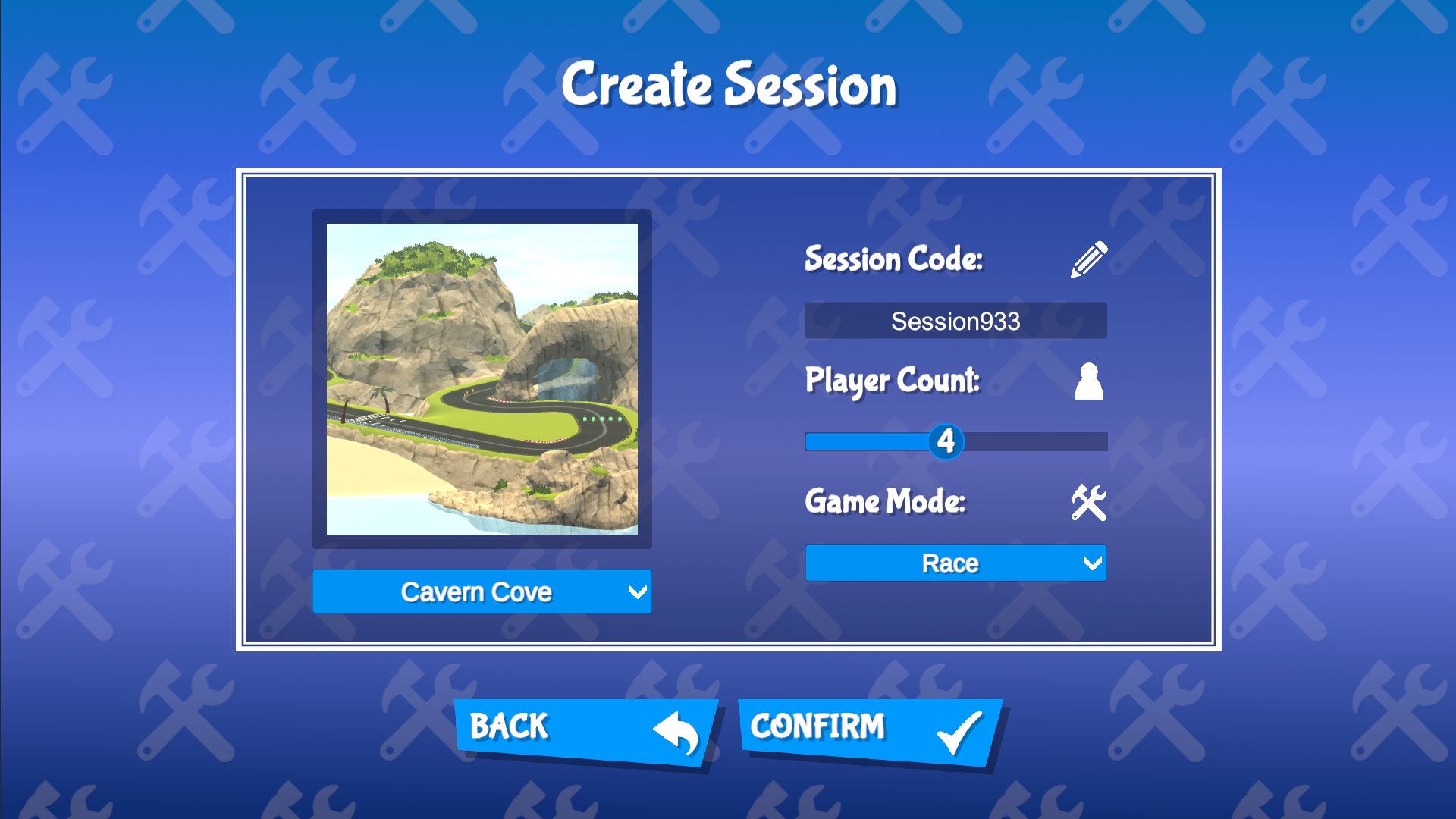
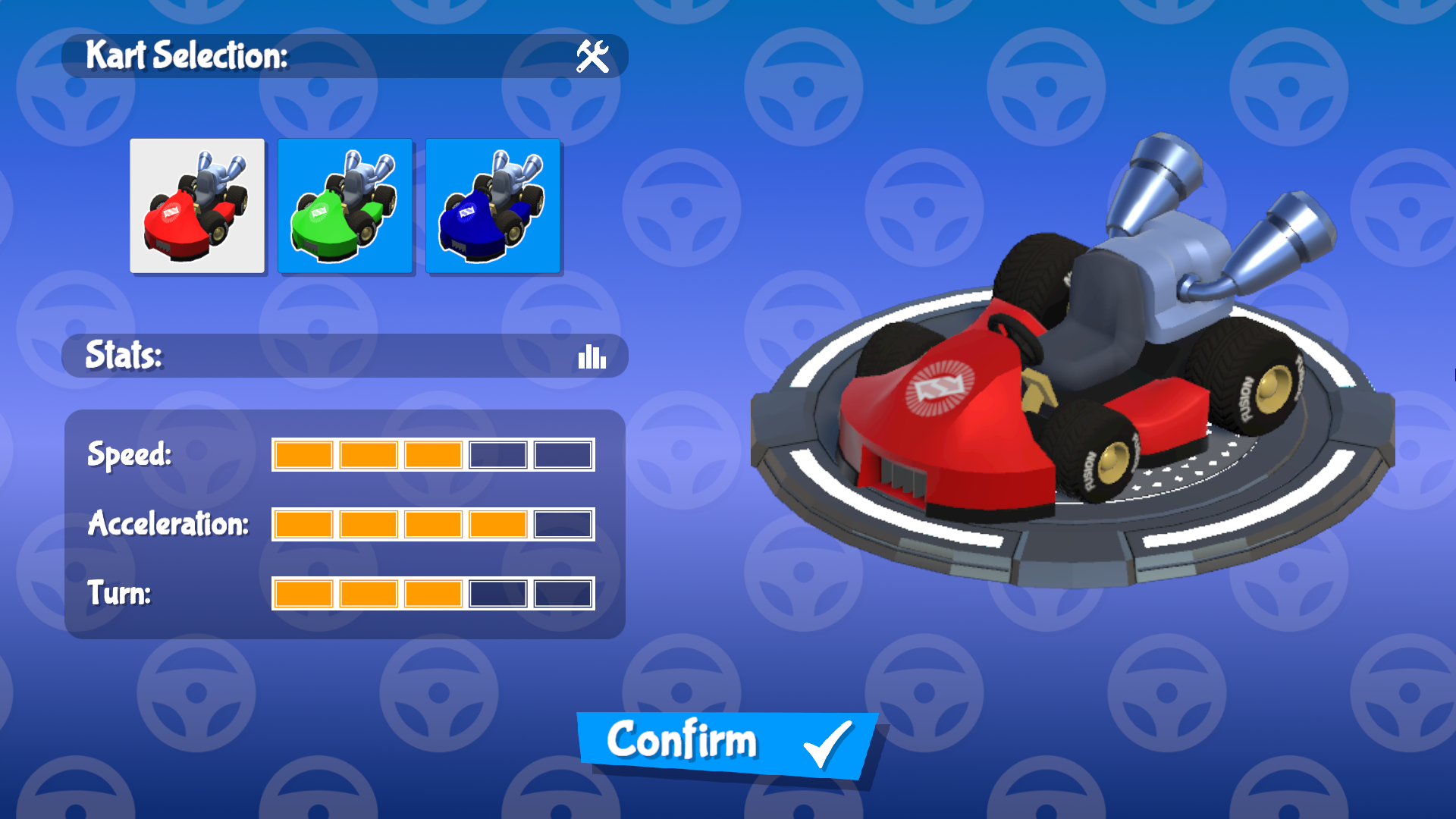
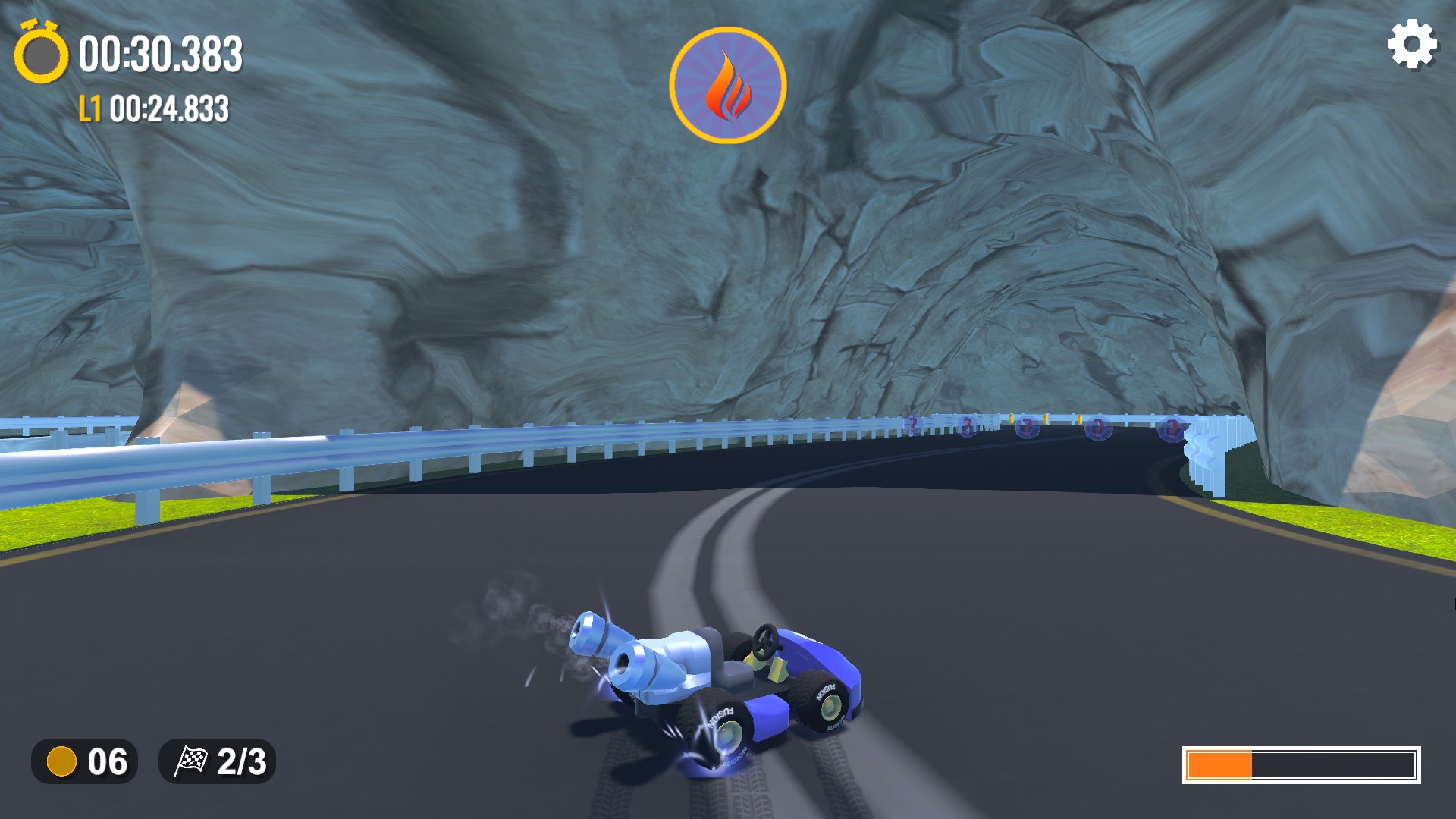
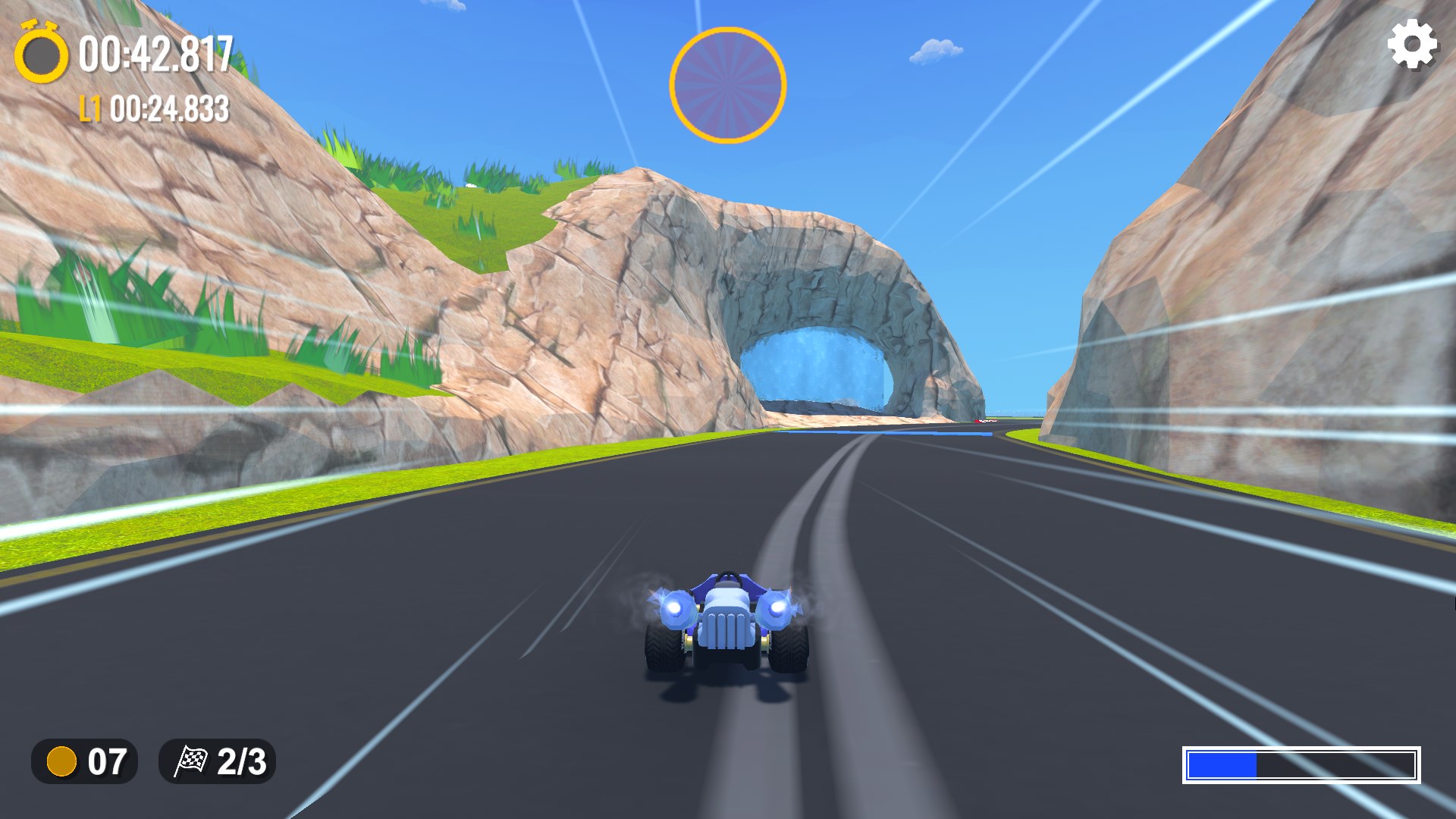
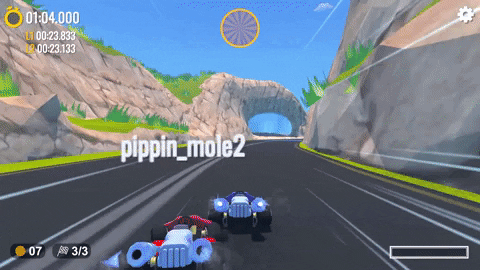
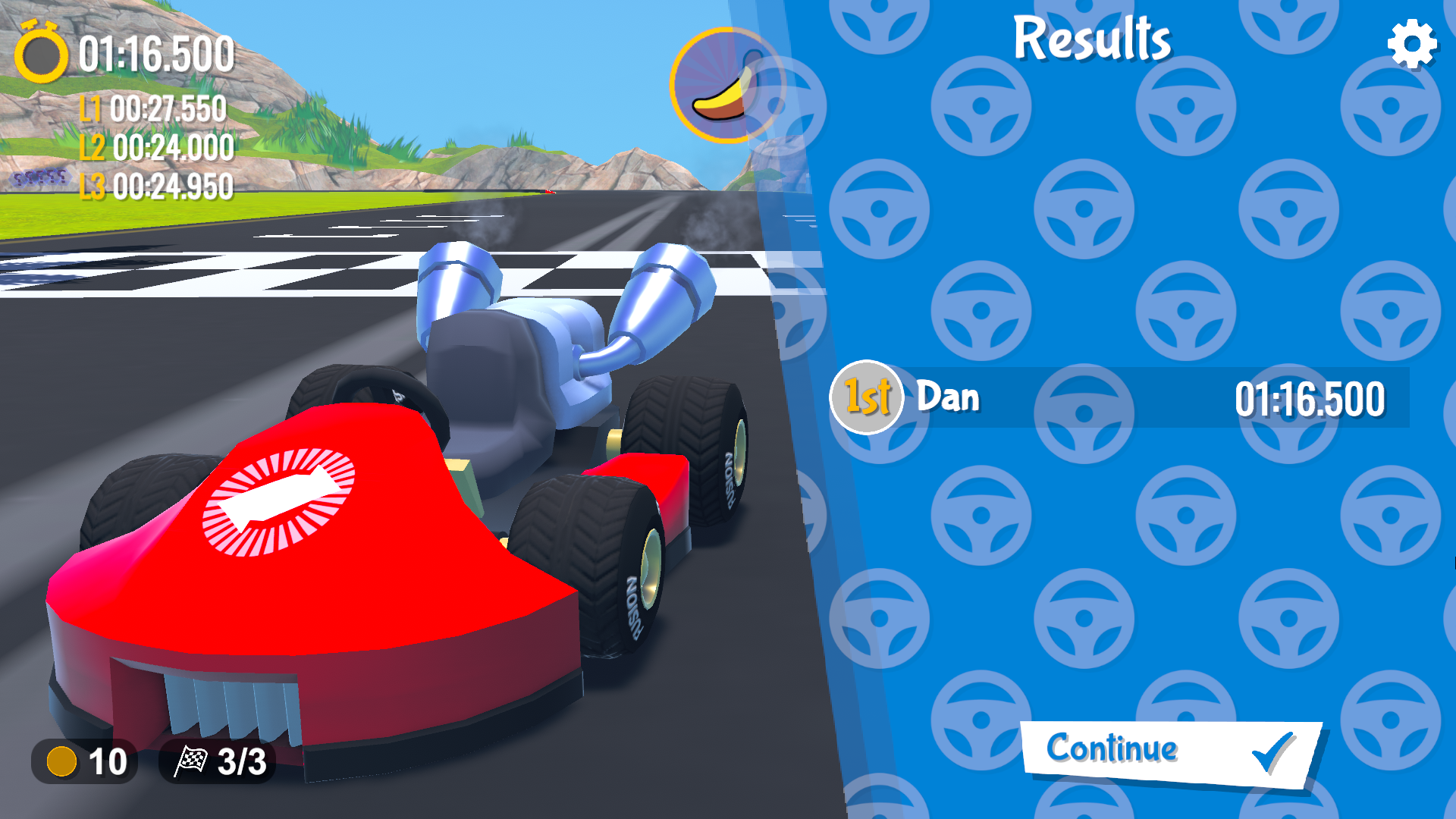
在您開始之前
為了運行範例,首先在Photon引擎儀表板中建立一個Fusion應用程式帳號,然後將它貼上到Real Time設定(可從Fusion選單到達)中的App Id Fusion
欄位。可以從Fusion選單Fusion > Realtime Settings
選擇Photon應用程式設定資產。
下載
版本 | 發佈日期 | 下載 | |
---|---|---|---|
2.0.5 | Mar 04, 2025 | Fusion Karts 2.0.5 Build 824 |
聚焦點
專案有一個完整的遊戲迴圈,最多允許8名使用者加入彼此,進行比賽,看看誰會贏,也可以在沒有計時器的情況下在無限圈的賽道上一起練習。主要功能包括:
- 兩種不同的模式(比賽和練習)
- 兩條賽道
- 具有不同統計資料的卡丁車
- 共享物品,如助推/香蕉障礙物、硬幣
- 基於可指令碼物件的項物品系統,其為可擴展
- 使用讓客戶端加入的代碼將房間設定為主機端,處理諸如「找不到房間」、「房間已滿」、「當前房間處於遊戲階段中」等邊緣情況。
資料夾架構
主指令碼資料夾/Scripts
有子資料夾Networking
與Fusion Helpers
,其屬於網路特定元件,而其他子資料夾包含各種介面和管理器元件等,這些元件控制遊戲遊玩。
遊戲啟動器
GameLauncher.cs
類別負責根據用戶從遊玩模式選單中選擇的選項,將使用者設置為Host
或Client
。它持有參照到UI,並負責管理諸如生成玩家、取消生成玩家和關閉之類的事情。
第一次啟動
首次啟動範例時,將提示使用者提供暱稱(如果需要)並選擇地區。可以隨時從選項選單存取這個畫面。提供的暱稱用於三個地方:
- 當使用者建立或加入一個房間時,會用他們提供的暱稱具現化UI物品。
- 在世界空間畫布中的比賽中,在每個卡丁車上方具現化暱稱。
- 在比賽結束時的成績畫面中,暱稱用於顯示第一、第二、第三名,依此類推。
房間
Fusion Karts範例使用房間,這是Photon Cloud遊戲階段頂層的抽象。對於此範例,一次最多可以有8個用戶連線到一個房間。該上限可以設定在1和8之間。
建立一個房間
CreateGameUI
指令碼在Canvas層次結構中的Create Room Screen
下儲存對各種UI元素的參照。Room Code
的輸入欄位將設定此房間的ID。當使用者嘗試加入大廳時,他們將被提示類似的輸入欄位,並且必須出示可識別的代碼。玩家計數的滑塊允許主機端透過設定CreateGameUI
指令碼中的ServerInfo.MaxUsers
來限制房間中的使用者數量。範例中包含兩種不同的遊戲模式和兩種不同賽道,可以在建立房間期間透過以下方法進行選擇,這些方法在ServerInfo.cs
中設定適當的整數值:
C#
public void SetGameType(int gameType)
{
ServerInfo.GameMode = gameType;
}
public void SetTrack(int trackId)
{
ServerInfo.TrackId = trackId;
trackImage.sprite = ResourceManager.Instance.tracks[trackId].trackIcon;
}
加入一個房間
若要加入房間,使用者必須提供房間代碼。如果使用者嘗試加入一個不存在的房間,他們會收到一條UI消息提示。使用者無法加入當前處於遊戲階段中的房間,也必須確保他們與嘗試連線的檔案室位於同一地區。JoinGameUI
指令碼包含對輸入欄位的參照,以提供所需的代碼。如果輸入欄位中沒有提供文字,則確認按鈕將變得無法互動,因為建立一個沒有代碼的房間是不可能的。
C#
private void SetLobbyName(string lobby)
{
ClientInfo.LobbyName = lobby;
confirmButton.interactable = !string.IsNullOrEmpty(lobby);
}
準備好
每個使用者的準備狀態顯示在玩家清單中他們的暱稱旁邊,使用者已準備就緒的話由綠色勾選標記表示。所有玩家必須在比賽開始前先做好準備。LobbyUI.cs
中的EnsureAllPlayersReady
函數訂閱了每個RoomPlayer
網路行為的PlayerChanged
事件,其IsReady
已連網屬性透過Fusion的Networked
屬性提供的OnChanged
回調。調用時,該函數會檢查每個RoomPlayer
的IsReady
布林值是否設定為真,然後調用LevelManager.LoadTrack
,其中sceneIndex
參數設置為所選賽道的相應索引。這個方法將Fusions設定為活躍中場景,然後調用已登錄的場景物件提供器。
處理輸入
此範例使用新的輸入系統,並利用InputAction
類別來處理對鍵盤和遊戲搖桿控制的支援。新的輸入系統使支援各種控制器變得容易。有開箱即用的鍵盤和搖桿支援,包括觸覺回饋。
鍵盤
- A與D或左與右箭頭鍵來轉向
- W或上箭頭鍵來加速
- S或下箭頭鍵來倒車
- Alt來向後看
- 空白鍵來跳躍/飄移
- Shift來使用喇叭/物品
搖桿
- 左類比操控桿來轉向
- 南按鈕來加速
- 東按鈕來倒車
- D盤下鍵來向後看
- 右緩衝鍵來跳躍/飄移
- 左緩衝鍵來使用喇叭/物品
(支援搖桿震動)
卡丁車
卡丁車由許多個體行為組成,所有這些行為都源於KartComponent
,KartEntity
是它們之間的樞紐,並提供週邊元件。元件包括:
KartAnimator
- 參照到Animator
元件、視覺效果元素(如粒子系統和軌跡)以及播放動畫和效果的方法。KartAudio
- 參照到卡丁車上的每個持久音訊源,負責發動機的音調和音量,並播放飄移音訊。KartCamera
- 控制攝影機的視角、視野和速度線粒子系統KartController
- 大部分邏輯和網路都發生在這裡;加速、轉向、飄移和助推,以及旋轉車輪和定向道路。KartInput
- 本機輸入輪詢並處理InputAction
啟用/停用和回調KartLapController
- 處理卡丁車的當前圈數和檢查點KartItemController
- 處理按下使用物品按鈕時的行為
此外,KartEntity
持有參照到GameUI
HUD介面,該介面本身不是元件結構的一部分,但提供了一種向外向玩家反映卡丁車狀態的管道。
拾取物
拾取物是本範例中用來標記卡丁車可以與之互動的鬆散定義的實體集的術語。所有拾取物都執行了ICollidable
介面,具體行為由ICollidable.Collide
執行方式決定。KartEntity
負責啟動互動,該互動在OnTriggerStay
方法中執行,並向其傳遞對KartEntity
執行方式的參照。使用OnTriggerStay
是由於網路環境中OnTriggerEnter
函數的不可靠性質。
- 硬幣:就遊戲遊玩而言,硬幣沒有任何功能性目的,但具有展示一個基本實體的功能,該實體可以由單個玩家收集,被取消生成,然後新增到UI中顯示的計數器中。
- 物品箱:雖然物品箱本身不是拾取物,但它也執行
ICollidable
,以協助它們的行為。物品箱負責在碰到時透過KartEntity.SetHeldItem
方法為卡丁車分配Powerup
。
強化物
強化物是一種特殊的拾取物,從物品盒中獲得,可以在收集後的任意時間使用,由玩家自行決定。
強化物有兩個組成元件;Powerup
可指令碼物件,和抽象SpawnedPowerup
類別。
SpawnedPowerup
繼承自ICollidable
並虛擬地執行ICollidable.Collide
,以便成為衍生類別的可選執行方式。還提供了一個虛擬Init
方法用於可選初始化,該方法在Powerup.Use
方法生成後立即被調用。
- 香蕉:當使用時,香蕉作為一個物理實體掉在卡丁車後面,對玩家來說是一種危險。
BananaPowerup
覆寫SpawnedPowerup.Collide
以便於旋轉碾過它的卡丁車。 - 速度提升:提供立即的2級速度提升。速度提升沒有香蕉那樣的物理代表,因此在速度提升後會立即取消生成。