Entity Prototypes
Introduction
To facilitate data driven design, Quantum features entity prototypes.
A Quantum Entity Prototype is a serialized version of an entity that includes:
- Composition (i.e. which components it is made of); and,
- Data (i.e. the components' properties and their initial value).
This allows for a clean separation of data and behaviour, while enabling designers to tweak the former without programmers having to constantly edit the latter.
Setting up a Prototype
Entity prototypes can be set up in the Unity Editor.
Basic
To create it, add the QuantumEntityPrototype
component to any GameObject.
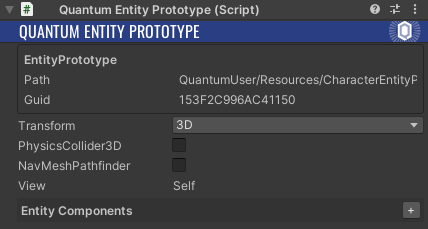
The QuantumEntityPrototype
script allows to set up and define the parameters for the most commonly used components for both 2D and 3D.
- Transform (including Transform2DVertical for 2D);
- PhysicsCollider;
- PhysicsBody;
- NavMeshPathFinder;
- NavMeshSteeringAgent;
- NavMeshAvoidanceAgent.
The dependencies for the Physics and NavMesh related agents are respected.
For more information, please read their respective documentation.
Custom Components
Additional components can be added to an entity prototypes via either:
- The + button in the
Entity Components
list; or, - The regular Unity Add Component button by searching for the right
QPrototype
component.
Note on Collections
Dynamic collections in components are only automatically allocated IF there is at least one item in the prototype. Otherwise, the collection will have to be allocated manually. For more information on the subject, refer to the Dynamics Collection entry on the DSL page.
Hierarchy
In ECS the concept of entity/GameObject hierarchy does not exist. As such entity prototypes do not support hierarchies or nesting.
Although child prototypes are not supported directly, you can:
- Create separate prototypes in the scene and bake them;
- Link them by keeping a reference in a component;
- Update the position of the "child" manually.
Note: Prototypes that are not baked in scene will have to follow a different workflow where the entities are created and linked in code.
It is possible to have hierarchies in game objects (View), however hierarchies in entities (Simulation) will have to be handled by you.
Creating/Instantiating a Prototype
Once an entity prototype has been defined in Unity, there are various ways to include it in the simulation.
Baked in the Scene/Map
If the entity prototype is created as part of a Unity Scene, it will be baked into the corresponding Map Asset. The baked entity prototype will be loaded when the Map is initialized with the values it was baked with.
N.B.: If a Scene's entity prototype is edited or has its values changed, the Map Data has to be re-baked (which depending on the project setup, it might be done automatically during some editor actions like saving the project).
In Code
To create a new entity from a QuantumEntityPrototype
, follow these steps:
- Create a Unity Prefab of the GameObject which has the
QuantumEntityPrototype
component; - Place the Prefab in any folder included in the
QuantumEditorSettings
asset inAsset Search Paths
, which by default includes all theAssets
folder:
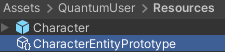
This automatically generates an EntityPrototype
asset which is associated with the prefab itself, as shown on the screenshot above.
- In the editor, it is possible to reference such
EntityPrototype
assets via fields of typeAssetRef<EntityPrototype>
. This is a way to reference the prototype of an entity to be created via the simulation code whilst having the referencing done similarly to the usual way it is done in Unity, performing a drag and drop or selecting from a list of assets.
Just to exemplify, the screenshot below has an example of referencing via a field already declared on theRuntimePlayer
class:
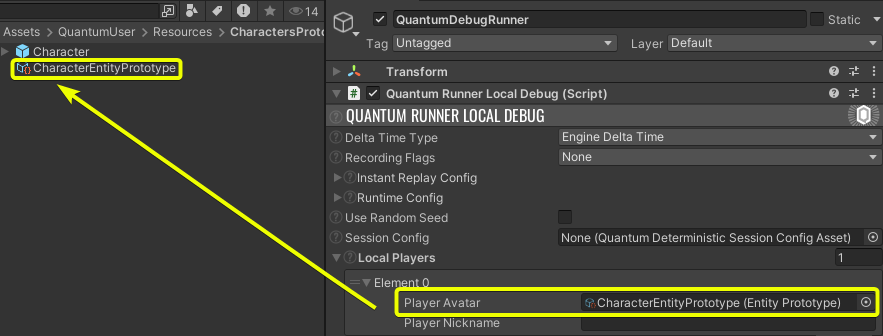
- Use
frame.Create()
to create an entity from the prototype. It is most commonly done by passing a reference to the asset shown above, but it also has other overrides:
C#
void CreateExampleEntity(Frame f) {
// Using a reference to the entity prototype asset
var exampleEntity = f.Create(myPrototypeReference);
}
Note
Entity prototypes present in the Scene are baked into the Map Asset, while prefabed entity prototypes are individual assets that are part of the Quantum Asset DataBase.
Quantum Entity View
The QuantumEntityView
corresponds to the visual representation of an entity.
In the spirit of data driven design, a Quantum Entity Prototype can either incorporate its View
component or point to a separate EntityView
Asset.
Self
To set an entity prototype's view to itself, simply add the QuantumEntityView
component to it.
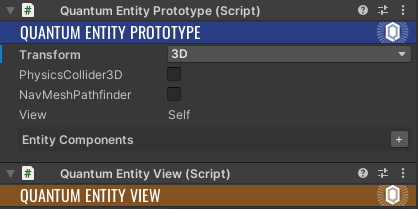
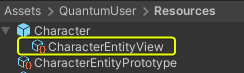
Separate from Prototype
To set up and link a view separate from the Entity Prototype asset:
- Add
QuantumEntityView
to the GameObject which should represent the view; - Create a Prefab of that GameObject;
- This will create an Entity View Asset nested in the prefab.
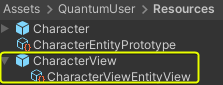
- Link the View field from an Entity Prototype which does not have a
QuantumEntityView
associate with it. Reference the newly created Entity View Asset. This can be done via drag-and-drop or using the Unity context search menu.
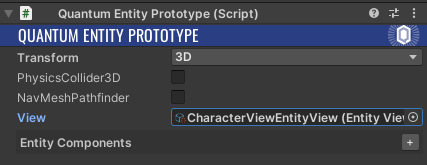
Important
For an Entity View to be visible in Unity, the scene has to have a QuantumEntityViewUpdater
script.