What's new in v1.2.13
Main Changes:
- Packet Encryption system
- Support for Gameye Session Hosting
- New Events API
- Matchmaking API with Lobby Data
- Bolt Debug Start UI Refresh
- Bolt Entity Array UI
For a full list of changes, check the log here.
Packet Encryption system
Security is one the main objectives when developing with Photon Bolt, for this reason, we've included now a native encryption system, that secures each package based on AES and Hashing algorithms.
The encryption setup is as easy as set all necessary keys, and you are done.
Bolt will use those keys to encrypt and decrypt all the packages without any other intervention.
We've also included a small sample showing how you can interact with the EncryptionManager
, the central class to setup the system that you can check inside the SetupEncryptionSystem
folder on the samples
package included in the SDK or directly on our Samples Repo.
The code sample below shows you can setup and reset the encryption system:
C#
void Load()
{
// The Encryption System includes some Utility methods to generate all necessary Keys
var IV = EncryptionManager.GenerateAesIV();
var key = EncryptionManager.GenerateAesKey();
var secret = EncryptionManager.GenerateHashSecret();
EncodedIV = Convert.ToBase64String(IV);
EncodedKey = Convert.ToBase64String(key);
EncodedSecret = Convert.ToBase64String(secret);
// Initlize the system just passing the keys as argument and done
EncryptionManager.Instance.InitializeEncryption(EncodedIV, EncodedKey, EncodedSecret);
}
void Unload()
{
// Reset all configurations on the Encryption System if you want to disable it
EncryptionManager.Instance.DeinitializeEncryption();
}
Support for Gameye Session Hosting
Photon Bolt now officially supports Gameye as Game Server Orchestration solution. You will be able to deploy, manage, and play using dedicated servers running directly on their infrastructure with benefits like reliability, auto-scaling, and low latency. You can read more about the integration and how to use it on your game on our dedicated page here.
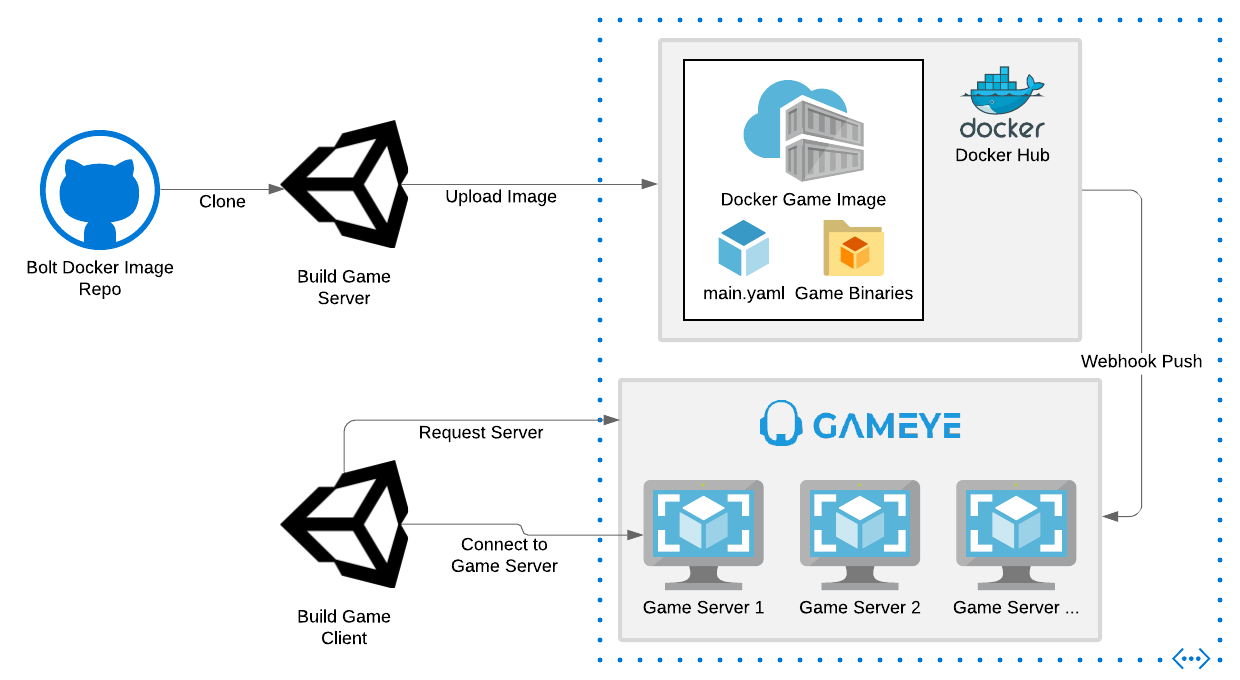
New Events API
We've included a new simplified API for the Bolt Events creation/sending that aims to make your code cleaner if you make extensive use of events for communication between your players/server. See the example below:
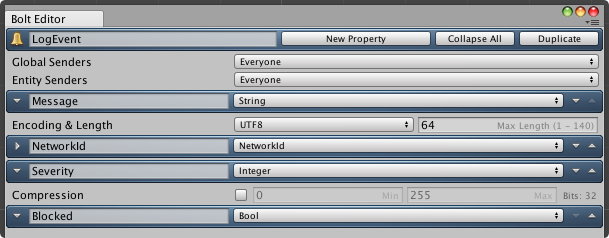
Based on this Bolt Event definition, we can perform the following code update:
C#
void sendEvent()
{
// Old
var evt = LogEvent.Create(GlobalTargets.AllClients, ReliabilityModes.ReliableOrdered);
evt.Message = "Just a message";
evt.NetworkId = GetEntityID();
evt.Severity = 2;
evt.Blocked = false;
evt.Send();
// New
LogEvent.Post(GlobalTargets.AllClients, ReliabilityModes.ReliableOrdered, "Just a message", GetEntityID(), 2, false);
}
The new API includes the new Post
extension method, with versions for all Create
methods that already exist on the current API.
The Create
method is not deprecated, and will continue to works as usual.
You can use both methods without any problems.
Matchmaking API with Lobby Data
The Matchmaking API grows with the addition of more information about the Lobby where your player is currently connected. This information can be useful to improve your matchmaking usage while making available more data to your player.
You can access Platform metadata by checking the property BoltMatchmaking.CurrentMetadata
, which now contains the key LobbyStatistics
.
It's only available for the PhotonPlatform
and contains a reference for a Photon.Realtime.TypedLobbyInfo
class, that you can use to check the number of currently connected players (PlayerCount
) and the number of rooms available (RoomCount
).
Bolt Debug Start UI Refresh
One of the key features of Photon Bolt is its rapid development, for that, it's included a small tool capable of running Bolt sessions with just a click.
This is called Bolt Debug Start
and is available as a custom window via the Bolt/Debug Start (Scenes)
menu item.
On this version, we've made a small UI refresh and included some new features:
- Build Options: you can now select some build options that will be used to compile the standalone executables. The options available are:
Development
, andBuildScriptsOnly
. - Build & Run and Run: on the previous version, you were able only to run the game by building it every time (
Build & Run
button, formerlyDebug Start
), now you will also be able to just re-run (Run
button) the executables, without the need to build it.
The rest of the buttons are self-explanatory based on the footer legend.
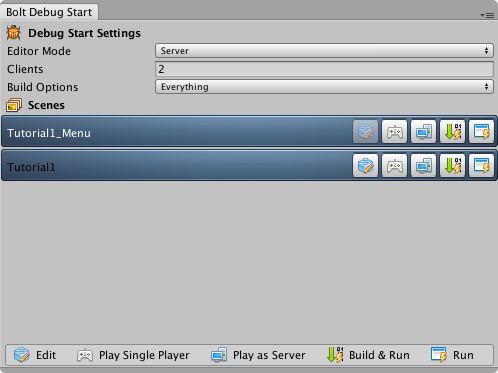
Bolt Entity Array UI
The Bolt Entity is a central part of the Bolt architecture, it uses a State
definition to describe the data to be synchronized between the players.
Two types of properties available are the objects and arrays of properties, that can be used to group related information within a single reference.
On this update, we've updated the Bolt Entity
component UI when dealing with those kinds of properties, aiming to reduce the CPU usage needed to draw all properties at once and make it more intuitive to visualize the data as an expandable dropdown list.
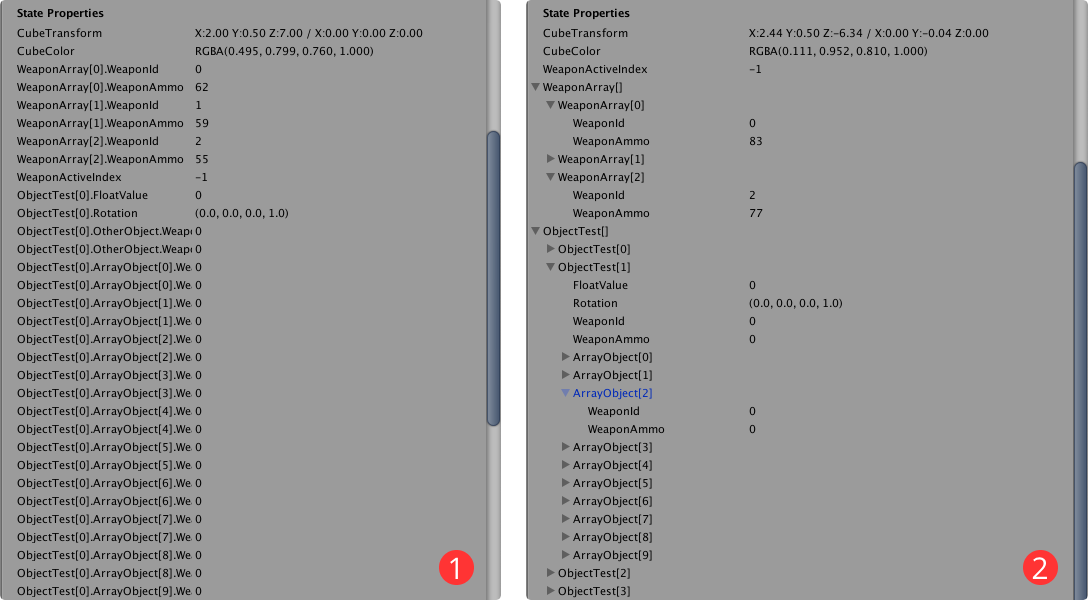