Temporal Anti Aliasing
Bolt has built-in support for handling the dis-joint relationship between Update
and FixedUpdate
in Unity, to put it a bit more bluntly: The simulation (FixedUpdate
) and rendering (Update
) portion of your game does not always line up 1:1 with each other.
This causes what's commonly known as 'micro-stutter'.
When you define a 'Transform' property on your state in Bolt, you need to assign a transform to it in the Attached
callback to have it sync over the network.
Normally a simple prefab would look like this:
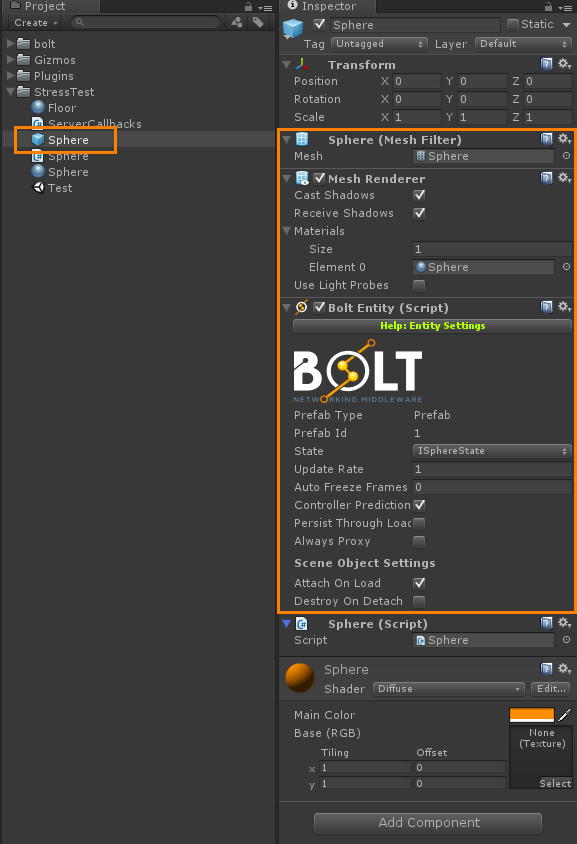
And the code for hooking up the transform like this:
C#
using UnityEngine;
using System.Collections;
public class Sphere : Bolt.EntityBehaviour<ISphereState> {
public override void Attached() {
state.Transform.SetTransforms(state.Transform, transform);
}
}
If you've used bolt past the first part in the 'Bolt 101' series, this should look very familiar.
Since all simulation happens in FixedUpdate
, this setup can lead to 'micro-stutter'.
The way to deal with this is something called Temporal Anti-Aliasing.
Read more about "Temporal Anti-Aliasing".
The first thing we need to do is to just setup a normal class field on our 'Sphere' class, and pass that in as the secondary value to SetTransforms.
C#
public class Sphere : Bolt.EntityBehaviour<ISphereState> {
[SerializeField]
Transform renderTransform;
public override void Attached() {
state.Transform.SetTransforms(state.Transform, transform, renderTransform);
}
}
We then split out the mesh rendering parts from our root game object into a child object and make sure to assign the new 'SphereMesh' child object to the 'Render Transform' property we created on our 'Sphere' script, like this:
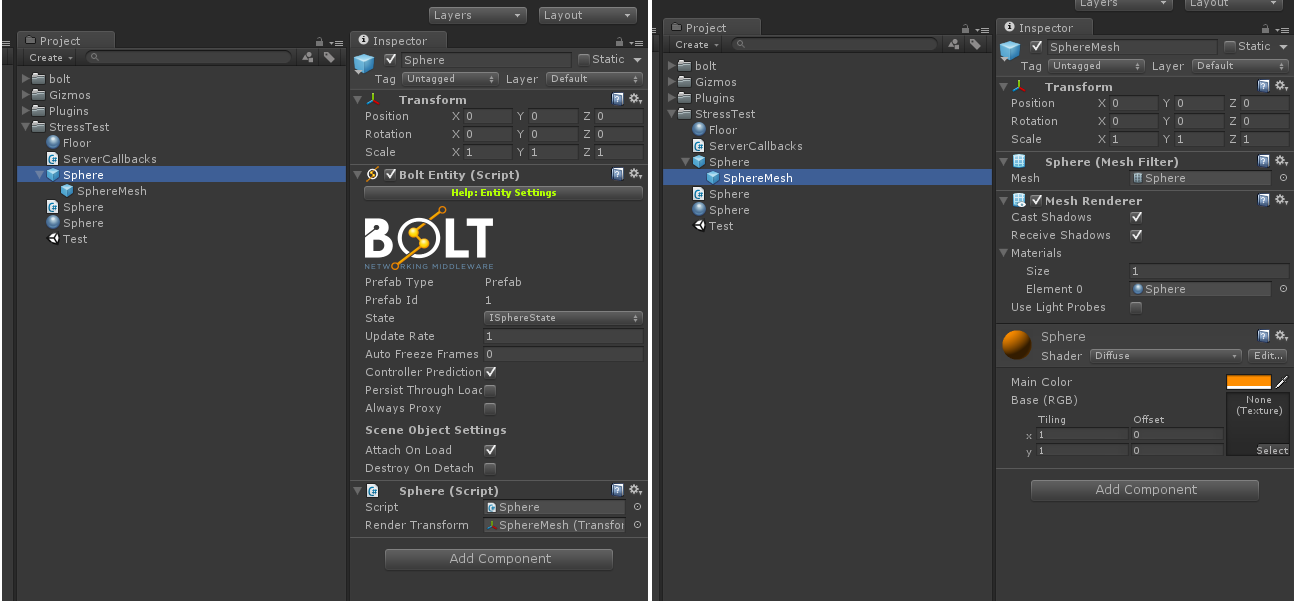
Now Bolt will take care of handling all artifacts which happen from FixedUpdate/Update not lining up perfectly.
Back to top