Data Layer
KCC Settings
The KCC
exposes some properties to the component inspector as a default configuration. Values are further configurable at runtime.
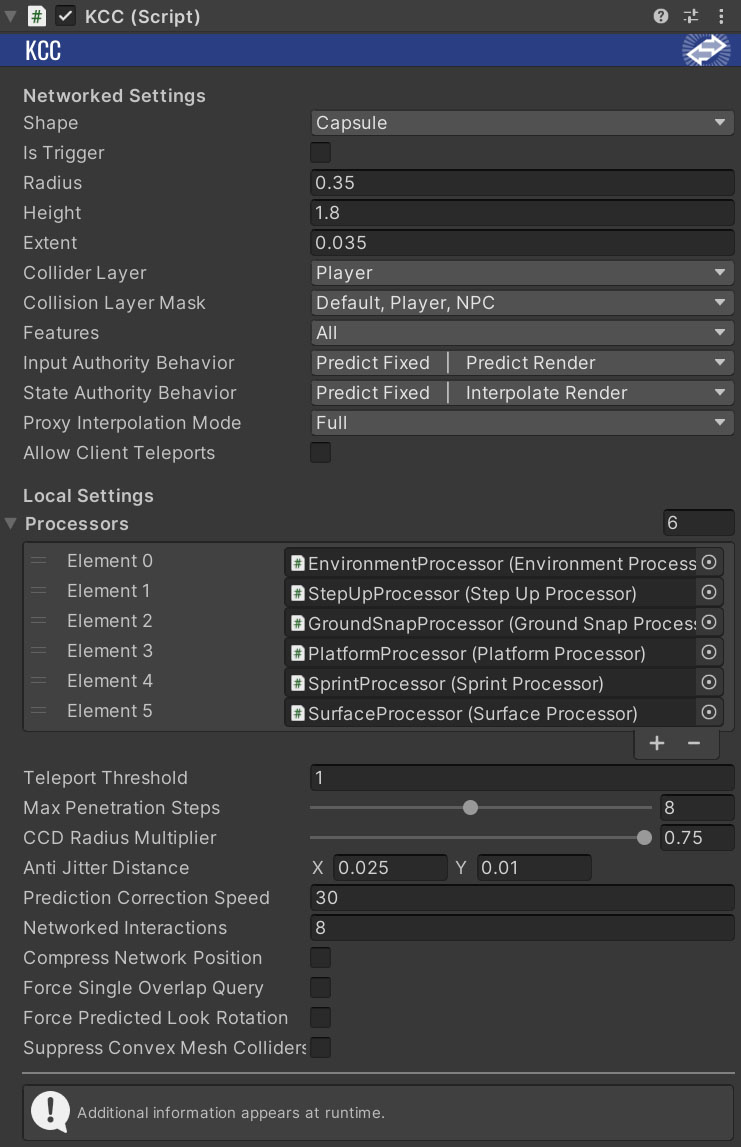
Networked Settings
These settings are synchronized over the network by default, changes on the server will be propagated to all clients automatically. Local overrides on clients are reverted before next resimulation tick.
Shape
- Defines KCC physics behavior.None
- Skips internal physics query, collider is despawned. Other logic can still be executed.Capsule
- Full physics processing, Capsule collider is instantiated as child game object.
Is Trigger
- KCC collider is marked as trigger.Radius
- Radius of the collider.Height
- Height of the collider.Extent
- Defines additional radius extent for surrounding collider detection. Low value decreases stability and has potential performance impact when executing additional checks. High value increases stability at the cost of increased sustained performance impact. Recommended range is 10-20% of the Radius.Collider Layer
- Layer of the collider game object.Collision Layer Mask
- Layer mask the KCC collides with.Features
- Default configuration of KCC features (runtime value can be overridden). More info can be found in Features section.CCD
- Enables continuous collision detection to prevent passing through geometry.Anti Jitter
- Enables move distance tolerance (position delta applied to Transform).Prediction Correction
- Enables smooth correction in case of incorrect prediction (solves incorrect prediction in both fixed and render updates).
Input Authority Behavior
- Defines update behavior for the KCC with input authority. In case the object has input and state authority at the same time this has priority over State Authority Behavior.Predict Fixed | Interpolate Render
- Full processing/prediction in fixed update, interpolation between last two predicted fixed update states in render update (Default).Predict Fixed | Predict Render
- Full processing/prediction in fixed update, full processing/prediction in render update.
State Authority Behavior
- Defines update behavior for the KCC with state authority.Predict Fixed | Interpolate Render
- Full processing/prediction in fixed update, interpolation between last two predicted fixed update states in render update (Default).Predict Fixed | Predict Render
- Full processing/prediction in fixed update, full processing/prediction in render update.
Proxy Interpolation Mode
- Defines interpolation behavior. Proxies predicted in fixed update are always fully interpolated.Full
- Interpolates all networked properties in KCCSettings and KCCData, synchronizes Transform and Rigidbody components everytime interpolation is triggered (Default).Transform
- Interpolates only position and rotation and synchronizes Transform component. This mode is fastest, but most KCCSettings and KCCData properties won't be synchronized.
Allow Client Teleports
- Allows execution of Teleport RPC initiated by client.
Local Settings
These settings are not synchronized over the network, changes on server or clients are always local.
Processors
- List of default processors which propagates to KCC.LocalProcessors upon initialization. AnyIKCCProcessor
can be linked here. More info about processors can be found in Interactions section.Teleport Threshold
- Defines minimum distance the KCC must move in a single tick to treat the movement as instant (teleport). This also affects interpolation.Max Penetration Steps
- Single Move/CCD step is split into multiple smaller sub-steps which results in higher overall depenetration quality.CCD Radius Multiplier
- Controls maximum distance the KCC moves in a single CCD step. Valid range is 25% - 75% of the radius. Use lower values if the character passes through geometry. This setting is valid only whenEKCCFeature.CCD
is enabled. CCD Max Step Distance = Radius * CCD Radius Multiplier.Anti Jitter Distance
- Defines render position distance tolerance to smooth out jitter caused by depenetration from geometry. Higher values reduces jitter more but may introduce noticeable delay when switching move direction.X
= Horizontal axis.Y
= Vertical axis.
Prediction Correction Speed
- How fast the prediction error interpolates towards zero. More info about prediction correction can be found in Features section.Networked Interactions
- Maximum interactions (collisions, modifiers, ignored colliders) synchronized over network. More info about interactions can be found in Interactions section.Compress Network Position
- Reduces network traffic by synchronizing compressed position over the network at the cost of precision. Useful for non-player characters.Force Single Overlap Query
- Perform single overlap query during move. Hits are tracked on position before depenetration. This is a performance optimization for non-player characters at the cost of possible errors in movement.Force Predicted Look Rotation
- Skips look rotation interpolation in render for local character. This can be used for extra look responsiveness with other properties still being interpolated.Suppress Convex Mesh Colliders
- Enable to always check collisions against non-convex mesh colliders to prevent ghost collisions and incorrect penetration vectors. More info can be found in Known problems section.
Modifying settings from code
Settings are accessible from script through property KCC.Settings
. The safest way to initialize them from another script is by registering a callback with KCC.InvokeOnSpawn()
.
C#
public class Player : NetworkBehaviour
{
protected override void OnSpawned()
{
// We don't know if the KCC is already spawned at this point.
// KCC.InvokeOnSpawn() ensures the callback is executed after KCC.Spawned() and its API called in proper order.
// If the KCC is already spawned the callback is executed immediately.
GetComponent<KCC>().InvokeOnSpawn(InitializeKCC);
}
private void InitializeKCC(KCC kcc)
{
// The KCC.Spawned() has been already called and we can safely use its API.
kcc.Settings.CCDRadiusMultiplier = 0.5f;
// We want to update KCC manually to preserve correct execution order.
kcc.SetManualUpdate(true);
}
}
⚠️ KCC automatically backs up settings in Spawned()
and restores in Despawned()
- making it compatible with object pooling.
KCC Data
KCCData
is core data structure to store runtime data. It contains:
- Metadata - frame, tick, delta time, interpolation alpha, …
- Persistent data - position, look rotation
- Input values - input direction, jump impulse, gravity, …
- Intermediate calculations - dynamic velocity, kinematic velocity, …
- Physics query results - ground normal, is grounded, …
- Move information - has teleported, has jumped, is on edge, …
- Collisions - collection of networked collisions the KCC interacts with
- Modifiers - collection of manually registered modifiers/processors the KCC interacts with
- Ignores - collection of ignored colliders
- Hits - collection of all colliders/triggers the KCC overlaps
Some important properties related to movement calculation:
Alpha
- Relative position of the time between two fixed tick times. Valid range is <0.0f, 1.0f>DeltaTime
- Partial delta time, variable if CCD is active. Valid range is <0.0f,UpdateDeltaTime
>, but can be altered.UpdateDeltaTime
- Delta time of render update / fixed tick (CCD independent).BasePosition
- Base position before move, initialized withTargetPosition
at the start of each KCC step.DesiredPosition
- Desired position before depenetration and post-processing.TargetPosition
- Final calculated (after depenetration) or explicitly set position which is propagated toTransform
.LookRotation
- Look rotation (pitch + yaw) of the KCCTransformRotation
- Yaw rotation (without pitch) of the KCCInputDirection
- Non-interpolated world space input direction - based on Keyboard / Joystick / NavMesh / ...JumpImpulse
- World space jump impulse vectorGravity
- Gravity vectorKinematicVelocity
- Velocity calculated fromInputDirection
,KinematicDirection
,KinematicTangent
andKinematicSpeed
.DynamicVelocity
- Velocity calculated fromGravity
,ExternalVelocity
,ExternalAcceleration
,ExternalImpulse
,ExternalForce
andJumpImpulse
.IsGrounded
- Flag that indicatesKCC
is touching a collider with normal angle lower thanMaxGroundAngle
.
For more information about KCCData
properties please check commented source code in KCCData.cs
file.
The KCC
further distincts data whether it is related to fixed update or render update:
- Fixed Data -
KCCData
instance used by default in fixed update -FixedUpdateNetwork()
(accessible throughKCC.FixedData
property) - Render Data -
KCCData
instance used by default in render update -Render()
(accessible throughKCC.RenderData
property) - History Data -
KCCData
instances (fixed data backups, accessible throughKCC.GetHistoryData(int tick)
) to restore data from local history when new snapshot arrives from the server
⚠️ The KCC
also contains property KCC.Data
, which automatically resolves whether a fixed or render update is currently active and returns the correct instance.
Following diagram shows data flow, mechanism behind render prediction, use of local history and reconciliation of data received from server (stored in network buffer).
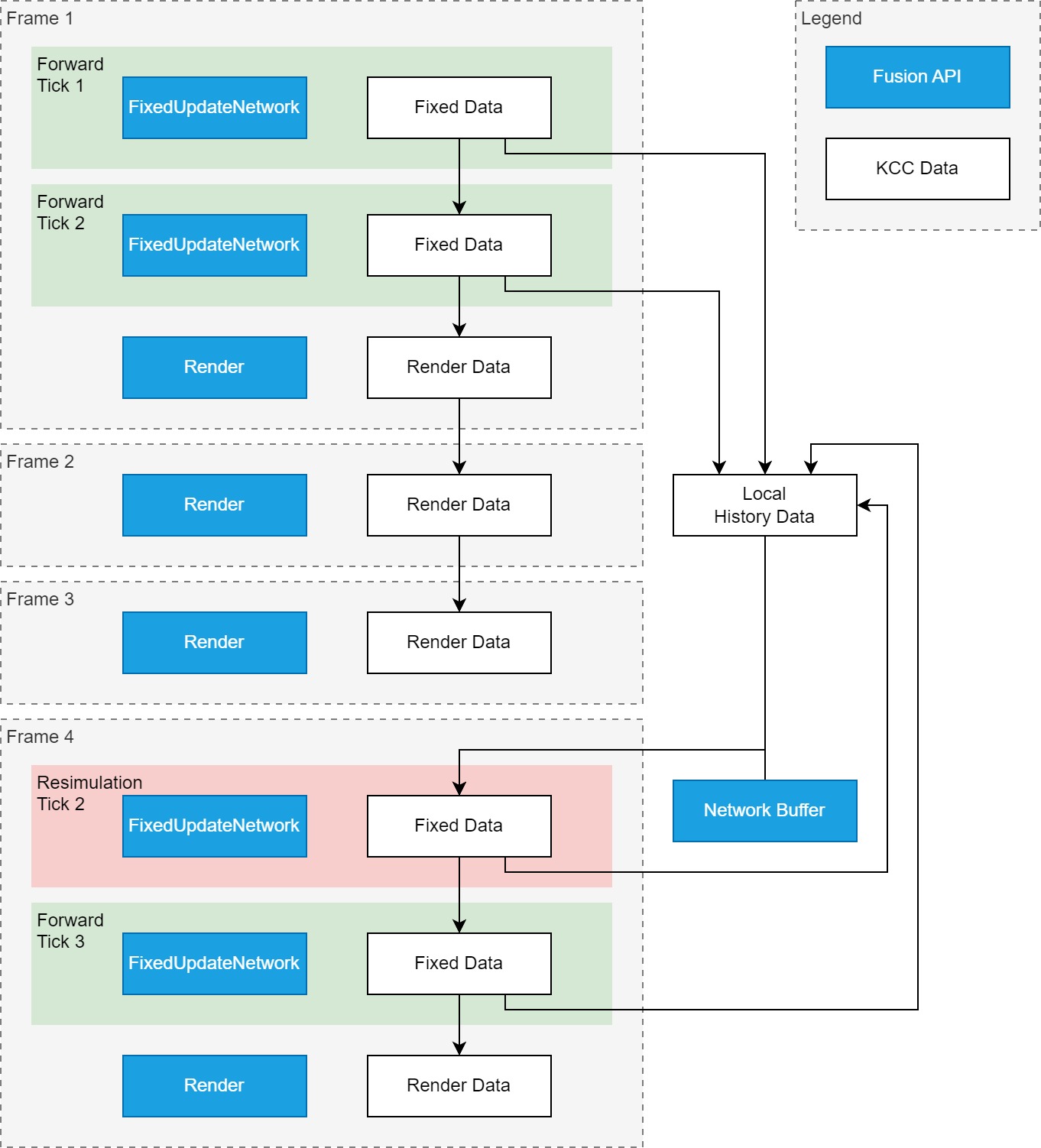
Frame 1
- Two Fusion ticks are simulated,
Fixed Data
is used by KCC during simulation. - After every tick the
Fixed Data
is copied to local history. - On the end of Tick 2 values from
Fixed Data
are also copied toRender Data
(to prepare data for render). - In
Render()
KCC works withRender Data
only.
Frame 2/3
- There’s no enough delta-time to simulate next Fusion tick.
- Only
Render()
is executed and KCC continues withRender Data
from previous frame.
Frame 4
- New data arrived from the server, with Tick 1 snapshot in network buffer.
- Whole
Fixed Data
for Tick 1 is restored from local history. - Values from
Network Buffer
are applied on top of restoredFixed Data
=> effectively overwriting all networked properties with correct state from the server. - Resimulation of Tick 2, on the end
Fixed Data
copied to local history. - Enough time passed for forward simulation of Tick 3, on the end
Fixed Data
is copied to local history and alsoFixed Data
copied toRender Data
(to prepare data for render). - In
Render()
KCC works withRender Data
only.
The approach above ensures that render predictions are completely separated from fixed simulation. Once there is enough delta-time to simulate next fixed tick, results from previous renders are thrown away and following render starts with the state from latest fixed tick.
Back to top