Overview

The Simple FPS game is a first-person shooter designed to provide an entry-level game sample with a straightforward code structure. Its primary focus is to serve as a learning resource for beginners and developers interested in creating their own first-person shooter games.
The gameplay revolves around intense deathmatch battles, where players engage in fast-paced combat within a realistic warehouse environment. With its simplistic design and clear code organization, the Simple FPS game offers an accessible starting point for understanding the fundamentals of creating multiplayer games with Fusion.
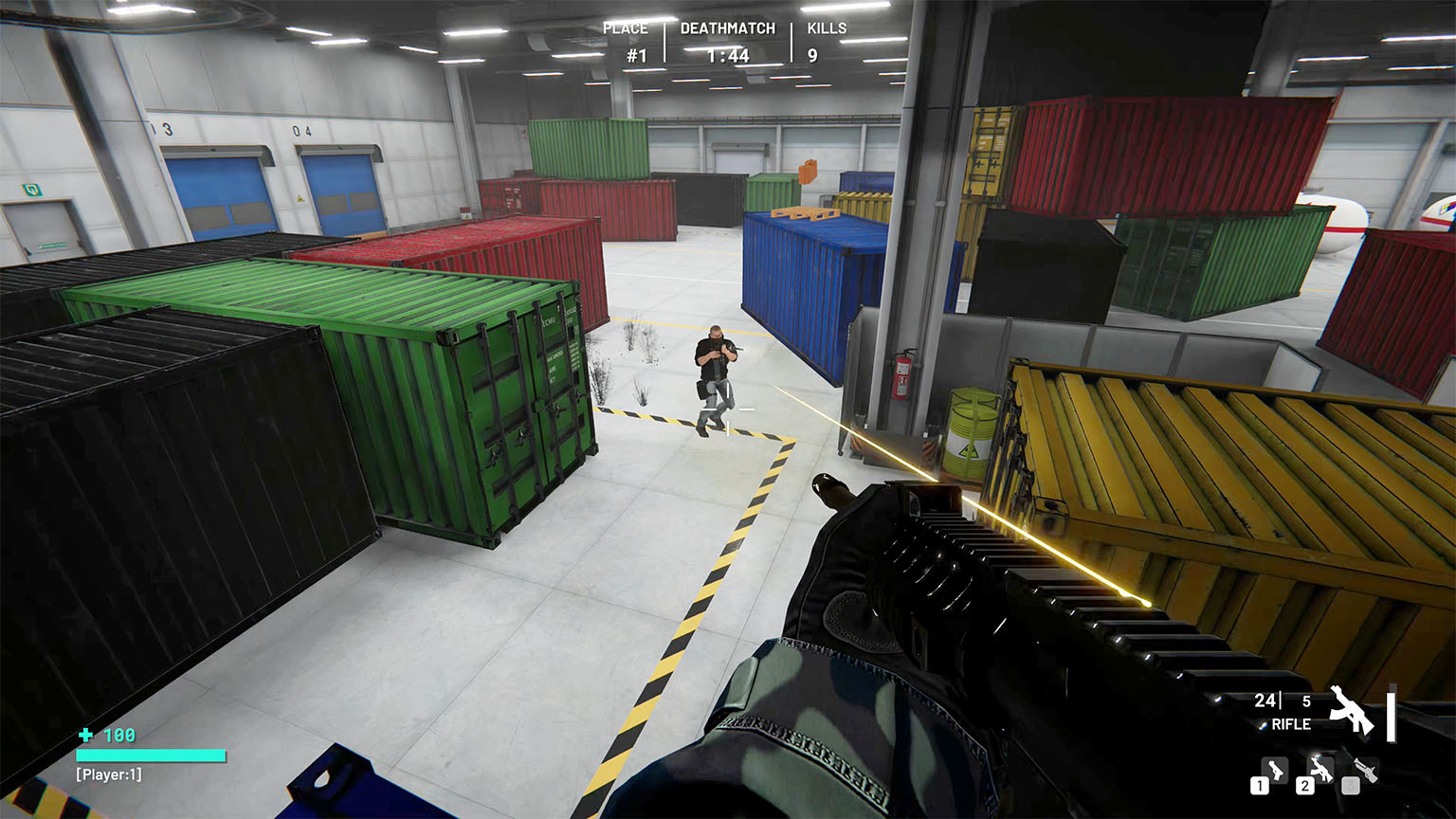
Features
- First-person shooter set in a warehouse environment.
- Deathmatch mode: Players respawn after a short time after death. The player with the most kills wins.
- Three hitscan weapons: Pistol, automatic rifle, and shotgun.
- Weapon and health pickups available throughout the map.
- Double Damage boost that lasts for 30 seconds during the match's final phase.
- Beginner-friendly code structure, facilitating ease of understanding and modification.
- Simple kinematic character controller (Simple KCC) for player movement.
- Photon Menu integration.
The Simple FPS also uses Client Side Prediction and Lag Compensation.
- Client Side Prediction is a popular multiplayer architecture in which clients use their own inputs to predict their movement before receiving confirmation from the server. This allows the gameplay to feel snappy and hides latency.
- Lag Compensation allows the server to temporarily view the world from each client's perspective and decide if players were in fact in a position to take shots.
Third Party Assets
The Simple FPS sample's core is made possible by Fusion; however, games require more than "just" tech! In addition to the multiplayer tech provided by Photon, the Simple FPS has been made possible thanks to the support of a group of excellent creators.