What's new in v1.2.10
Main Changes:
- API Extension for Bolt Connection
- Force Scene Synchronization
- Search Path feature to Bolt Assets
- UDP Platforms Custom Meta Data
For a full list of changes, check the log here.
API Extension for Bolt Connection
On this version, more metric information was exposed about the packages that transit through a Bolt Connection. Now it is possible to get the number of packets received, sent and lost. Also, you can check which type of connection was established with the remote end of the connection, Direct or Relayed.
Here is an example to show those stats and connection type on a Bolt client based on the connection with the Game Server:
C#
using UnityEngine;
namespace Bolt.Samples.GettingStarted
{
[BoltGlobalBehaviour(BoltNetworkModes.Client)]
public class ClientMetrics : Bolt.GlobalEventListener
{
private void OnGUI()
{
var conn = BoltNetwork.Server;
if (!conn) return;
GUI.Label(new Rect(10, Screen.height - 140, 300, 20), string.Format("Sent {0}", conn.PacketsSent));
GUI.Label(new Rect(10, Screen.height - 120, 300, 20), string.Format("Recv {0}", conn.PacketsReceived));
GUI.Label(new Rect(10, Screen.height - 100, 300, 20), string.Format("Lost {0}", conn.PacketsLost));
GUI.Label(new Rect(10, Screen.height - 80, 300, 20), string.Format("Type {0}", conn.ConnectionType));
}
}
}
Force scene synchronization
Extending the feature added in version 1.2.6, alongside disabling the remote scene loading automatically, you can now force the synchronization easily. This can be useful if you don't want to load scenes on the clients on the first moment if you are waiting for a minimum number of player for example, and then just force the sync for the in-game scene when the number is reached.
We consider this an advanced feature, for this reason, it's now available only through code and the UI element on the Bolt Settings window as removed. It should be used with care, as you will need to use other artifices to do the scene management of your game.
Here we show how to disable the scene auto load:
C#
var config = BoltRuntimeSettings.instance.GetConfigCopy();
config.disableAutoSceneLoading = true;
// START SERVER
BoltLauncher.StartServer(config);
// OR
// START CLIENT
BoltLauncher.StartClient(config);
In order to load the same scene loaded by the game server, from the client, you can just call BoltNetwork.LoadSceneSync()
.
This will automatically make the client load the scene currently loaded by the server.
See the example below:
C#
public void SyncScene()
{
if (BoltNetwork.IsClient)
{
BoltNetwork.LoadSceneSync();
}
}
Search Path feature to Bolt Assets
Photon Bolt needs to maintain a list of all Bolt Entities prefabs at compilation time in order to properly register them on the internal database and spawn entities at runtime. In order to facilitate the creation of this database, without intervention of the dev, Bolt needs to look on all folders of the project looking for possible candidates, what could cause delays during the game development. On this version, we've included the option to select only certain paths inside your game project where Bolt will search for Entity prefabs, without the need to load all hierarchy of directories.
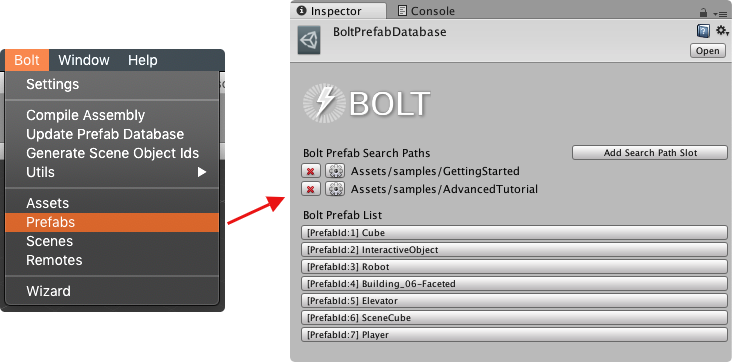
Open the Bolt Prefabs window, and include any number of search paths.
You can remove/edit those paths anytime.
Be aware that if you have folders in the same hierarchy, only the most specific will be used during the lookup (this is signaled by a gray text on the path).
When you run the Bolt compilation (Bolt/Compile Assembly
), the prefabs manager will consider only the selected paths.
UDP Platforms Custom Meta Data
Some platforms integrated with Photon Bolt can generate some metadata related specifically to the running integration.
In most of the cases, this is custom information that are not generally available for other platforms.
With this in mind, we've included on the BoltMatchmaking
utility class a new field, CurrentMetadata
, a dictionary that will contain any meta information exposed by the active platform.
The list of available data may grow over time, feel free to request new information.
On version 1.2.10, we've exposed the current region that your peer is connected when using the Photon Cloud platform (PhotonPlatform
), as shown below:
C#
public string GetCurrentRegion()
{
if (BoltMatchmaking.CurrentMetadata != null && BoltMatchmaking.CurrentMetadata.ContainsKey("region"))
{
return BoltMatchmaking.CurrentMetadata["region"] as string;
}
return null;
}
Back to top