Projectiles
Main projectile scripts (HitscanProjectile
and KinematicProjectile
) have two usages:
- Implement
GetFireData
andOnFixedUpdate
methods which are used to generate and manipulate projectile data (HitscanData
orKinematicData
). These methods are called fromFixedUpdateNetwork
on both the State Authority and the Input Authority, and are called directly on prefabs not on prefab instances.
C#
public virtual KinematicData GetFireData(Vector3 firePosition, Vector3 fireDirection) {}
public virtual void OnFixedUpdate(ref KinematicData data) {}
💡Note: Projectile is not a NetworkBehaviour
and is not associated with a NetworkObject
. So all network data used in GetFiredData
or OnFixedUpdate
must be included in the projectile data struct.
- Projectile instances are also used as a visual representation of the projectile. This allows for shared
FUN
(simulation) andRender
functionality such as movement code. Methods that are executed for projectile instances areActivate
,Deactivate
andRender
and are purely visual.
C#
public virtual void Activate(ref KinematicData data) {}
public virtual void Deactivate() {}
public virtual void Render(ref KinematicData data, ref KinematicData fromData, float alpha)
Every projectile can spawn visual effects on impact as well as a new NetworkObject
(e.g. explosion).
Projectile Types
Fusion Projectiles include several projectile types which may be used in many common scenarios.
Hitscan Projectile
Raycast with specified max distance which fires immediately on fire input, and hits are immediately evaluated.
InstantHitscanProjectile
Hitscan projectile with an instant hit effect. A visual trail can be displayed extending from the weapon barrel to the target position. The trail fades over time.
Example weapon: Sniper (disappearing trail), Shotgun (instant visuals)
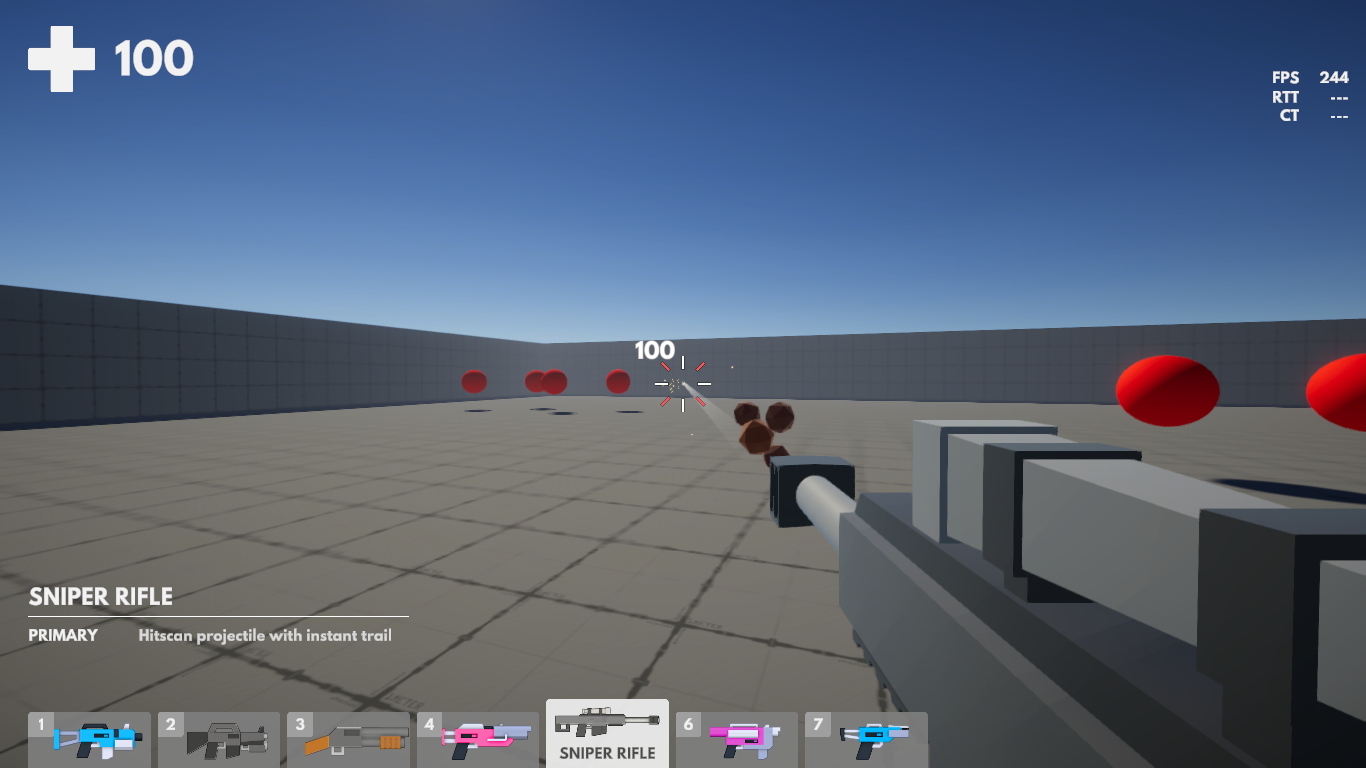
FlyingHitscanProjectile
Hit is processed immediately but there is a dummy flying projectile that travels with specified speed to the target position.
Example weapon: Rifle
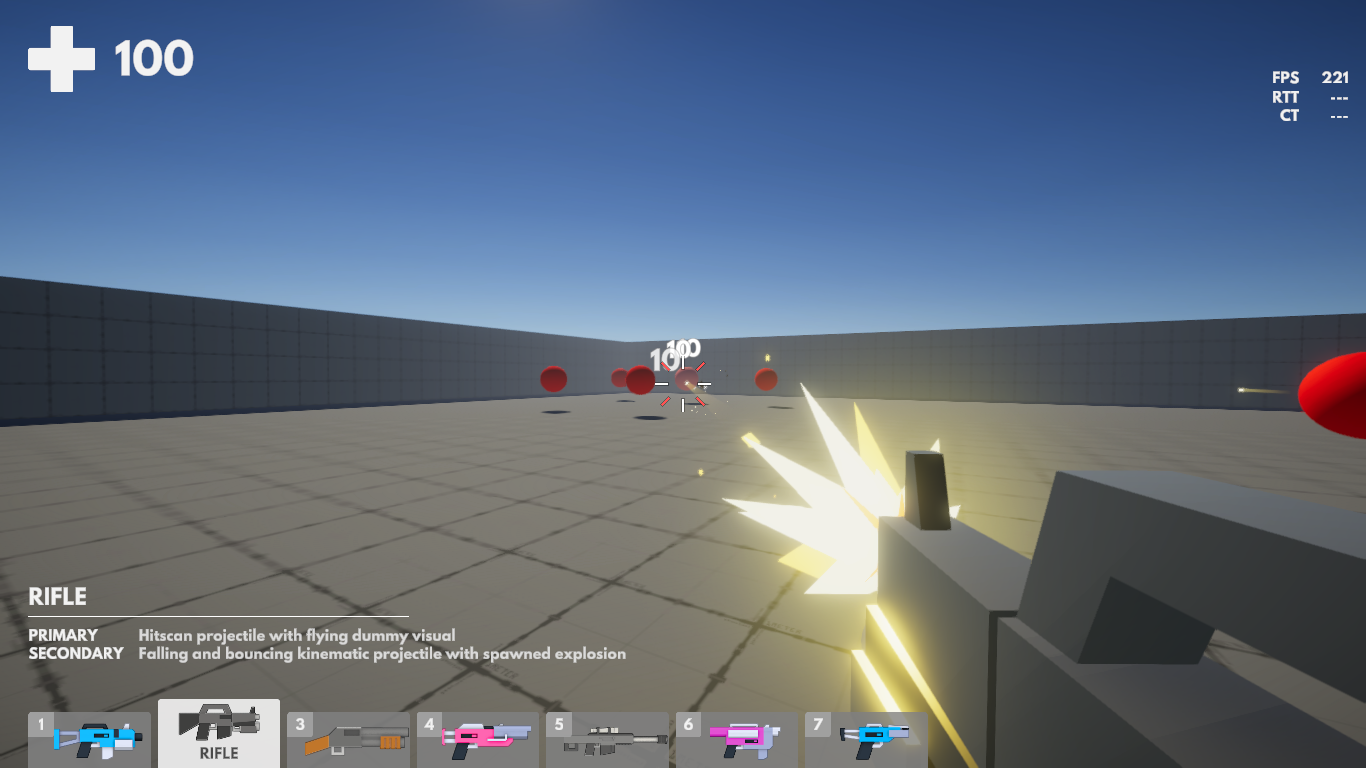
Kinematic Projectile
Projectile that travels over time, executing short raycasts between the previous position and new position every simulation tick.
SimpleKinematicProjectile
Kinematic projectile flying in a straight line.
Example weapon: Pulse Gun
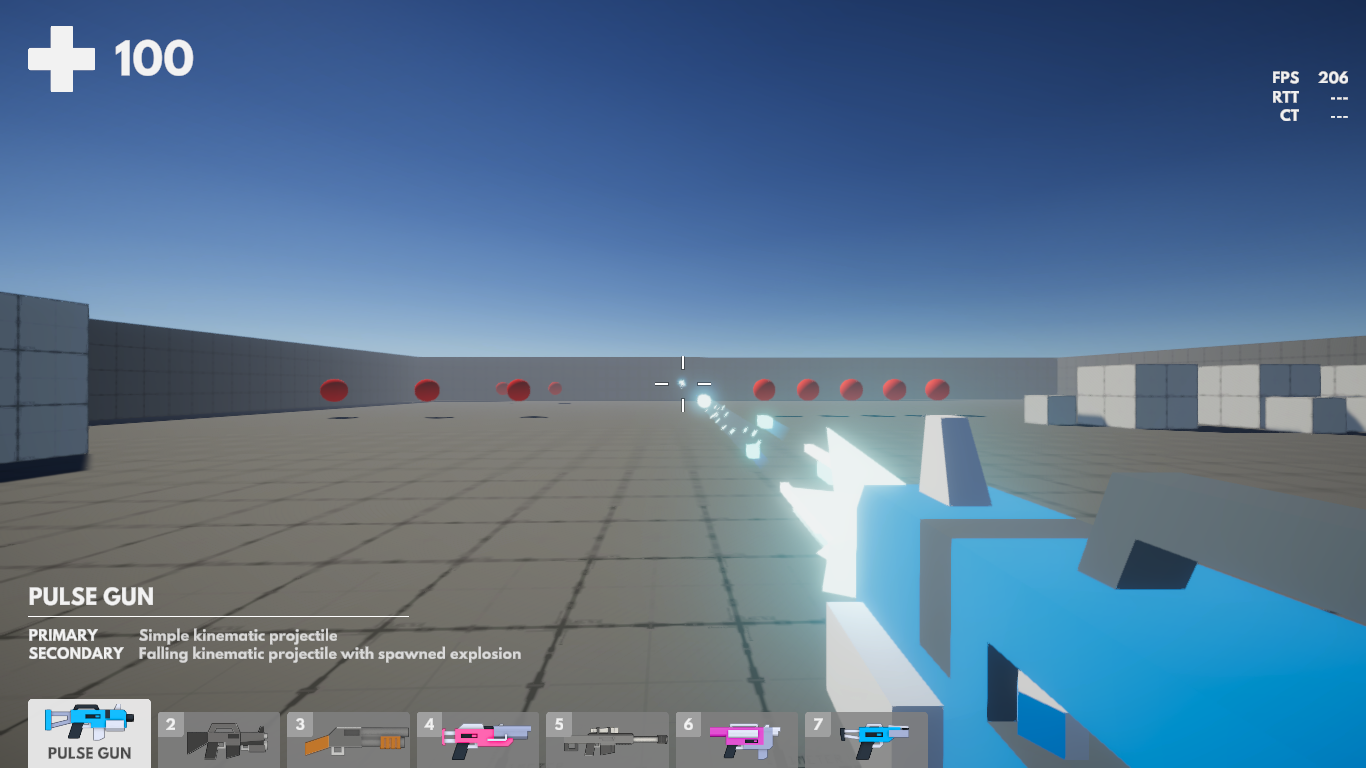
AdvancedKinematicProjectile
Kinematic projectile that can be influenced by gravity and bounce off walls and other objects. Multiple projectile behaviors can be managed by this projectile type:
Falling projectile with explosion on touch
Example weapon: Pulse Gun (alternative fire - Grenade)Falling and bouncing projectile with explosion after some time
Example weapon: Rifle (alternative fire - Grenade)Straight bounce projectile
Example weapon: Ricochet Gun
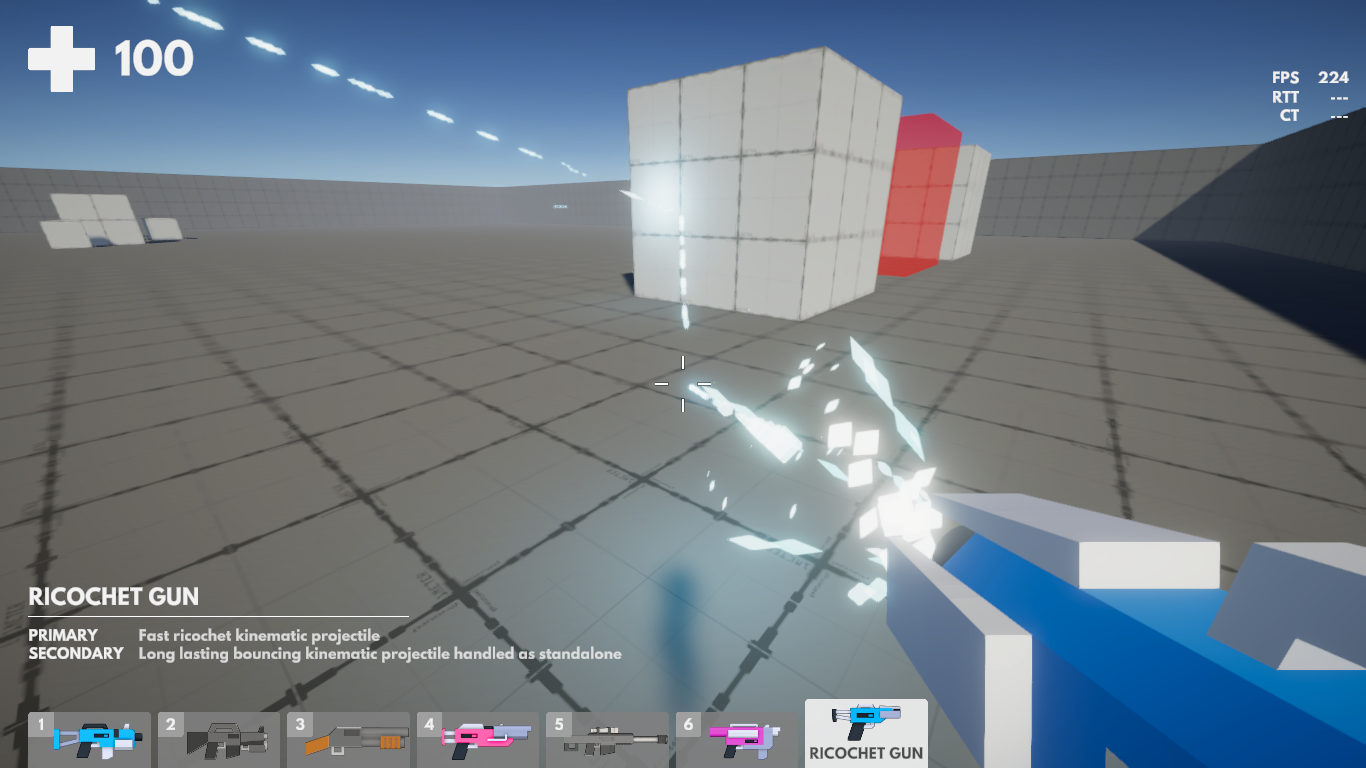
HomingKinematicProjectile
Projectile that actively turns to hit the target. It can predict target position to hit moving targets. Possibility to specify what body part should be targeted, properties of the turn and target seek behavior.
Example weapon: Homing Gun (primary fire for fast homing projectile, secondary fire - Rocket - for slower retargeting projectile)
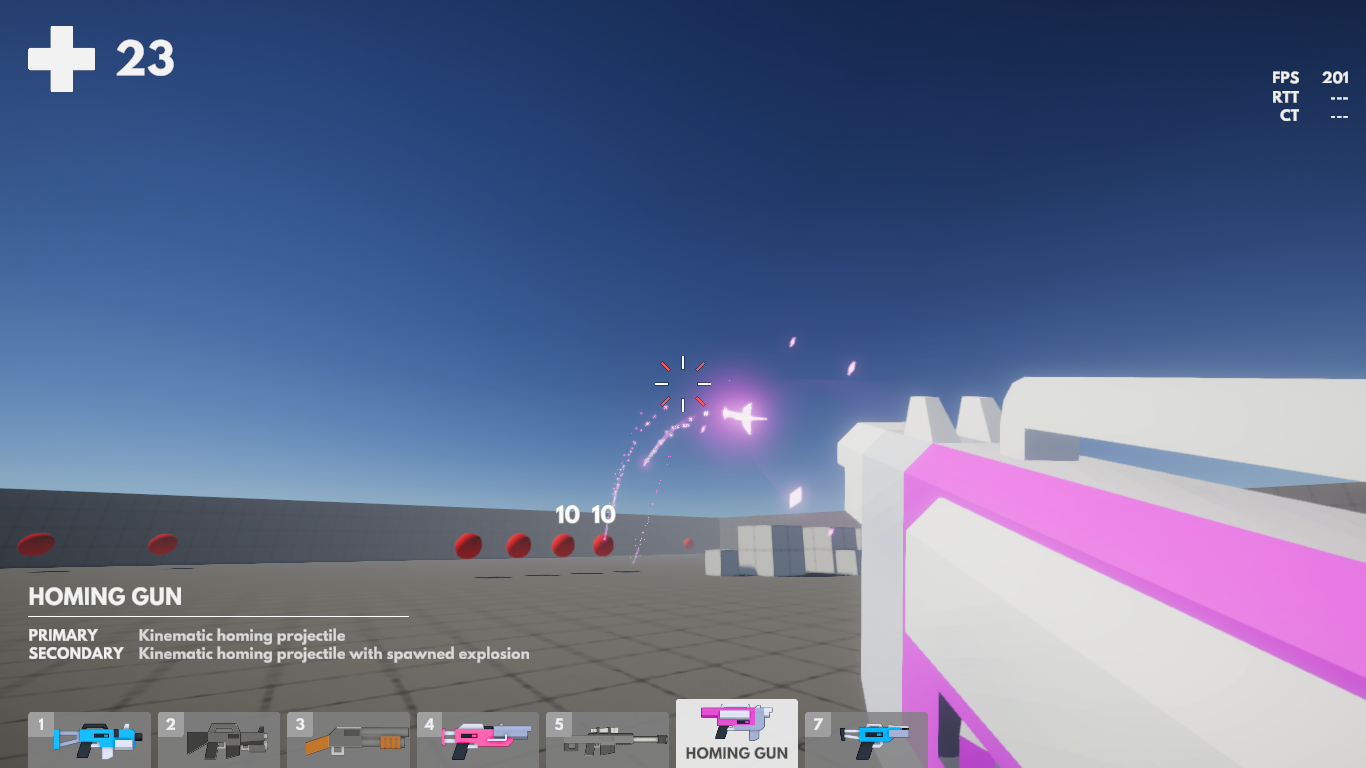
SprayProjectile
A projectile with very specific behavior that mimics a spray. To achieve this effect, the projectile can slow down, rise up or down, or change its diameter over time. When fired with higher dispersion, this projectile can represent the spray-like behavior of various weapons such as flamethrowers, acid guns, healing sprays, icing guns, and similar devices.
Example weapon: Flamethrower
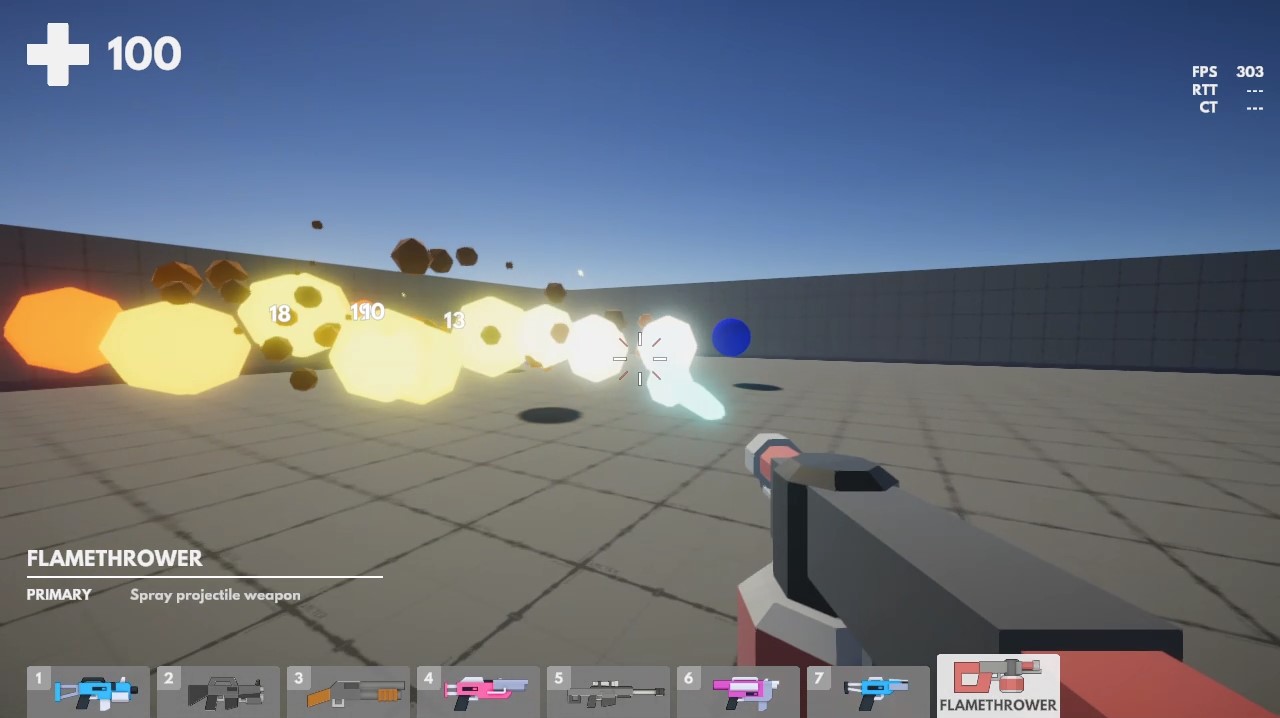
Ray
Constant ray which does damage over time. Ray is implemented using the weapon component WeaponBeam
(more in Weapon Components section), it isn’t really a projectile in this project.
Example weapon: Laser Gun

Standalone Projectile
Projectile that can be spawned as a standalone network object. Should be used only for some special circumstances. See the Choosing The Right Approach section in Projectile Essentials documentation.
StandaloneProjectile
Wrapper for standard projectile functionality. Creates and updates KinematicData
in a similar fashion as KinematicProjectileBuffer
, just does it only for one projectile. Can be spawned in advance using NetworkObjectBuffer
to mitigate any spawn delay when network conditions are not ideal.
Example weapon: Ricochet Gun (alternative fire - Bouncing Drop)
Projectiles Interpolation
When firing projectiles in first person games one can choose to do the projectile calculations directly from the weapon barrel (realistic approach) or from center of the camera (standard approach).
💡Note: Realistic approach is used in a few (hard core) games. It makes firing feel more realistic, but also less stable (fire position is dependent on player animations) and prone to unwanted hits (corner issue) because the weapon is offset from the camera.
Fusion Projectiles uses the more standard approach of firing from the camera center. This method however creates a discrepancy between the simulated path (originating at the camera), and the rendered path (originating from the weapon barrel). This is solved by interpolating projectile position over time from the weapon barrel to the real projectile path. Interpolation values (interpolation time, interpolation ease) can be configured for all kinematic projectiles.
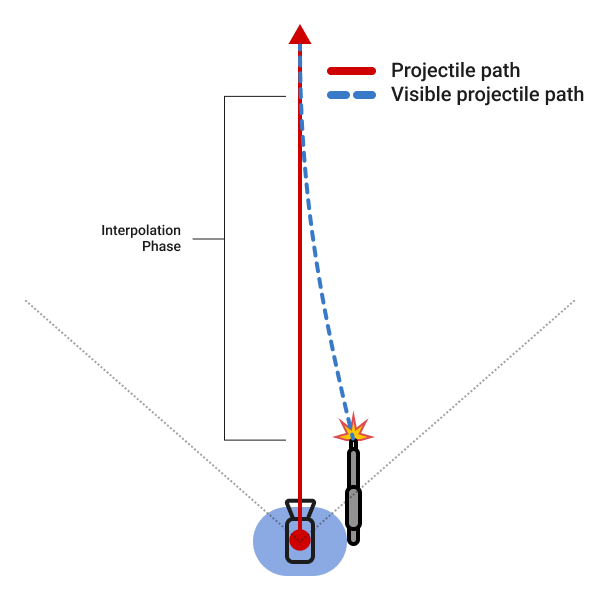
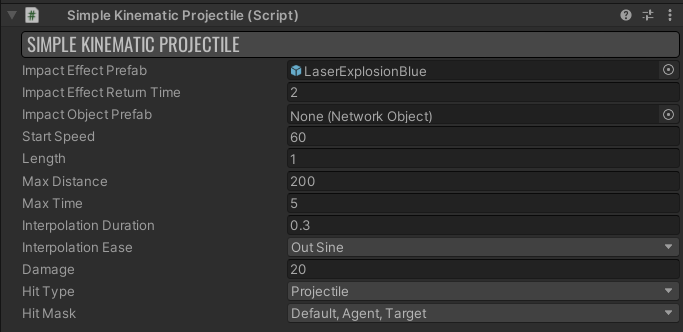
💡Note: Projectile interpolation is needed only for kinematic projectiles. For hitscan projectiles no interpolation is needed because we already know where the projectile will impact so the dummy flying visuals can be moving directly to that point.
Back to top