What's New

Quantum SDK Is Now A Unitypackage
Moving to a Unitypackage makes the initial setup and upgrading easier for everyone and lays the ground to distribute Quantum through the Asset Store and/or UPM in the future.
- The source for
QuantumGame
(formerly known as the quantum.code project) now lives inside Unity Assets folder:Assets/QuantumUser/Simulation/
. - Unity CodeGen step has been removed, all the code generation is now handled by the Qtn/DSL CodeGen and it's running inside Unity Editor.
- To clean up the Unity integration a considerable amount Quantum types and scripts were moved, renamed and merged. During migrations script GUIDs are preserved and to reduce the number of compilation errors obsoleted scripts with legacy names are provided
QUANTUM_ENABLED_MIGRATION
. - Unity/Odin property attributes can be used at will. This includes
[SerializeReference]
and Odin-specific serialization extensions. Any use of non-deterministic Unity API is still heavy discouraged. - New assets will receive an
AssetGuid
that is deterministic. This enables collecting all the Quantum assets possible without loading their contents, dramatically speeding up the process. AssetBase
is no longer needed:AssetObject
s are alreadyScriptableObjects
. This makes asset hierarchies more flexible and no longer limited to partial extensions model for Unity-only properties.- The minimum Unity Version was increased to
2021 LTS
to reduce the amount of legacy code.
See the Quantum Project for details about the SDK content.
Input Delta Compression
Input messages are now by default delta compressed, which greatly reduces the overall bandwidth. The network transport mode for server messages changed to reliable, which simplifies the Quantum input protocol.
While still supporting raw input mode delta compression is enabled by default.
Replays also store delta compressed input and are considerably smaller now.
New Protocol For Adding And Removing Players
The Quantum start protocol was changed to support adding and removing players during runtime without having to reserve slots at the start.
AddPlayer()
and RemovePlayer()
replace the method SendPlayerData()
. Unlike SendPlayerData, AddPlayer can only be send once per player slot.
The simulation can react to added and removed players using the ISignalOnPlayerAdded
and ISignalOnPlayerRemoved
signals.
The view can react to local player slots being added by CallbackLocalPlayerAddConfirmed
and OnLocalPlayerRemoveConfirmed
as well as errors by listening to OnLocalPlayerAddFailed
and OnLocalPlayerRemoveFailed
.
AddPlayer()
has a rate limitation on the server and cannot be spammed.
Throughout the API the wording of parameters has been changed slightly to better differentiate between players (PlayerRef) and player slots (local players).
A Player
always refers to the actual global player index while PlayerSlot
always is a local player slot used for example when controlling multiple players from one client. If only one local player slot is used it will be slot 0
.
The input callback CallbackPollInput
for example now uses the PlayerSlot
property. QuantumGame.AddPlayer(Int32 playerSlot, RuntimePlayer data)
explicitly names the parameter to be identified as a local player slot.
More information in the Player Manual
Predicted Commands
Command are now immediately available on the next predicted frames. This has been a feature request since some time, and it made sense to add it now that there is a new version and improved input protocol
When sending commands they are now added to the next prediction frame and are accessible during non-verified frames. The actual predicted tick number should be close to 99.9% accurate.
This way commands can be more responsive and reflect gameplay changes much more quickly.
Webhooks
Webhooks are an improvement to secure games with a custom backend: room creation, room joining, RuntimePlayer, RuntimeConfig can be intercepted and validated by a backend using HTTP requests.
Webhooks also enable replay streaming directly from the server.
They are enabled and configured using the Photon dashboard.
See the Webhook Online API Documentation for more information.
Quantum 3 AppIds
Quantum 3 applications require explicitly creating a Quantum 3 AppId on the Photon Dashboard.
Read the Quantum 100 - Create And Link A Quantum AppID tutorial for more information.
Photon Realtime 5
The new Realtime major version comes with .Net async extensions to improve writing and handling network connections.
Be sure to read the Photon Realtime release notes found under:
To start a Photon client connection and join a Photon room only one method has to be called and awaited
:
C#
MatchmakingArguments connectionArguments = new MatchmakingArguments {
PhotonSettings = PhotonServerSettings.Default.AppSettings,
PluginName = "QuantumPlugin",
MaxPlayers = 8,
UserId = Guid.NewGuid().ToString(),
NetworkClient = new RealtimeClient { ClientType = ClientAppType.Quantum }
};
RealtimeClient client = await MatchmakingExtensions.ConnectToRoomAsync(connectionArguments);
By default all errors are thrown as exceptions. Calls to async API need to be wrapped into try/catch blocks.
More information about the Realtime extensions are found in the Photon Async Extensions.
The SessionRunner Class
QuantumRunner
and SessionContainer
have been merged into the SessionRunner
class which is located in the Quantum Game project. It uses the new Photon Realtime 5 library.
Quantum game sessions can be started in an async and non-async manner:
C#
SessionRunner.Arguments sessionRunnerArguments = new SessionRunner.Arguments {
RunnerFactory = QuantumRunnerUnityFactory.DefaultFactory,
GameParameters = QuantumRunnerUnityFactory.CreateGameParameters,
ClientId = client.UserId,
RuntimeConfig = runtimeConfig,
SessionConfig = QuantumDeterministicSessionConfigAsset.DefaultConfig,
GameMode = DeterministicGameMode.Multiplayer,
PlayerCount = 8,
StartGameTimeoutInSeconds = 10,
Communicator = new QuantumNetworkCommunicator(client),
};
QuantumRunner runner = (QuantumRunner)await SessionRunner.StartAsync(sessionRunnerArguments);
Awaiting
the start will resume when the Quantum start protocol has finished and possible snapshots have been received.
Try out the QuantumSampleConnection.unity
scene for the most simple way to connect and start an online Quantum simulation.
New Demo Menu
The new demo menu replaces the lobby menu sample from Quantum 2.1. It received a graphical upgrade (in Unity.UI) and implements two simple online modes: random matchmaking and party-code sharing.
The demo menu code and prefabs are distributed with the SDK inside a `unitypackage`` and can be installed with the QuantumHub Tutorials&Sample section.
It was designed to be extendable and handle the complexity of dealing with connection logic. These design goals compete with each other and this solution tries to balance them reasonably. Parts of the menu implementation and prefabs are also used by other SDKs like Fusion.
![]() |
![]() |
![]() |
![]() |
![]() |
The demo scene makes use of TextMeshPro. When opening the demo scene for the first time the TMP installation pop up is shown.
The Quantum menu scripts will still compile when TMP disabled: The Quantum.Unity assembly definition will set the QUANTUM_ENABLE_TEXTMESHPRO
when the TMP Unity package (com.unity.textmeshpro
) is found.
Read here for information about how to customize the menu: Sample Menu Customization
RuntimeConfig And RuntimePlayer Json Serialization
The manual serialization of these files has been replaced by Json. The configs are still send over the Quantum protocol but as compressed Json strings.
The original serialization always required manual maintenance which was inconvenient and a source for errors. Additionally when forwarding the configs via HTTP requests for webhooks, the receiving backend would have to have the original C# code to deserialize it correctly.
The method SerializeUserData()
is now obsolete.
To serialize the configs to a byte array the QuantumGame.RuntimePlayerSerializer
is required which is set when starting a runner using SessionRunner.Arguments
and usually set to the default Quantum Json serializer QuantumJsonSerializer
.
C#
var runtimeConfig = new RuntimeConfig();
var runtimeConfigBinary = RuntimePlayer.ToByteArray(runtimeConfig, new QuantumJsonSerializer());
var runtimeConfigOther = RuntimePlayer.FromByteArray(runtimeConfigBinary, new QuantumJsonSerializer());
// make sure to check if runtimeConfig and runtimeConfigOther are equal to validate the json serialization
The maximum size for a serialized binary RuntimePlayer (or commands) is 24 kB
. Additionally if multiple clients send large chunks of data this way and they do not fit into one input message, they will be accepted by server in consecutive ticks.
Quantum Hub
The Quantum Hub will pop up when vital configs files are not found. The Fusion SDK proved that using a Hub window is the most convenient way to install and generate user files (which are not included in the package). This setup only has to be performed once and the resulting files will be kept inside version control with the rest of the project.
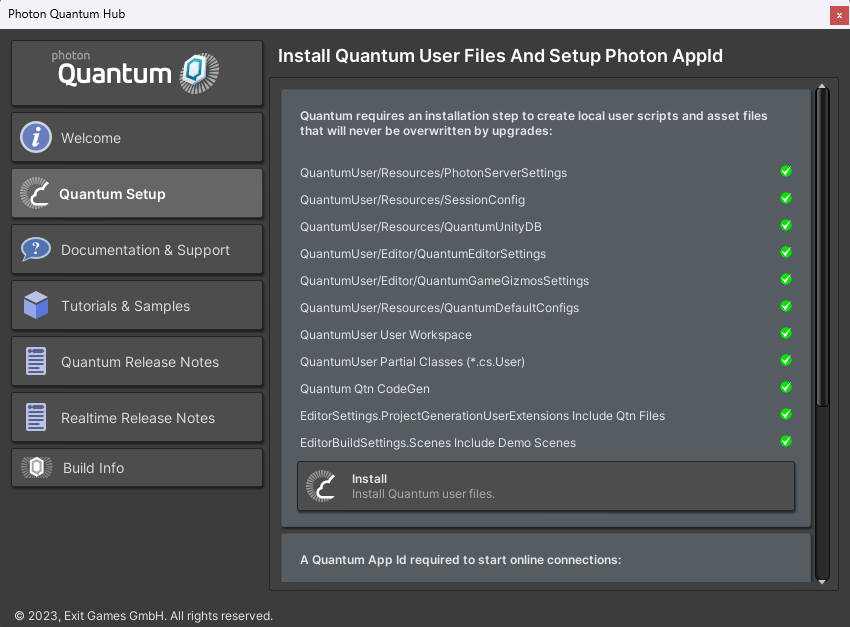
Quantum CodeDoc Inspectors
The Quantum Unity inspectors got a visual upgrade and will include toggle-able help text which is generated from inline XML code comments.

Data-Driven System Setup
The selection of Quantum systems to start can vary on the game mode or the map selection. A data-driven way is introduced using the SystemsConfig
asset. Different combinations of systems and subsystems combined in a Quantum asset can now be referenced by the RuntimeConfig
.
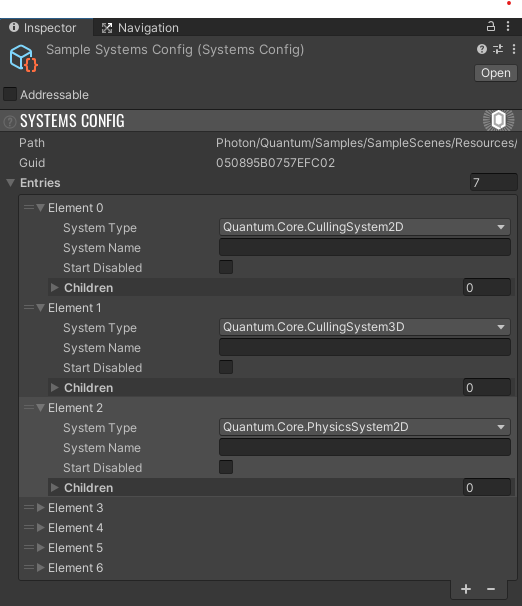
The Quantum 2.1 static SystemSetup.CreateSystems()
will still work but is considered obsolete and will log a warning. The class can be deleted after upgrading a Quantum 2.1 project and migrating the content.
Systems get created in this order (see DeterministicSystemSetup.CreateSystems()
):
- The old
SystemSetup.CreateSystems()
class and method exists (checked by reflection) -> call and and skip the rest - Provided
SystemsConfig
has entries -> create and add systems and continue - Provided
SystemConfig
is invalid or has no entries -> create and add default systems and continue - Finally the partial user method is called
DeterministicSystemSetup.AddSystemsUser()
to allow final touches
Old: file: quantum_code/quantum.code/SystemSetup.cs
C#
namespace Quantum {
public static class SystemSetup {
public static SystemBase[] CreateSystems(RuntimeConfig gameConfig, SimulationConfig simulationConfig) {
return new SystemBase[] {
// ..
}
}
}
}
New: file: Assets/QuantumUser/Simulation/SystemSetup.User.cs
C#
namespace Quantum {
using System.Collections.Generic;
public static partial class DeterministicSystemSetup {
static partial void AddSystemsUser(ICollection<SystemBase> systems, RuntimeConfig gameConfig, SimulationConfig simulationConfig, SystemsConfig systemsConfig) {
systems.Add(new TestSystemMainThreadGroup("TestSystemsGroup", new SystemMainThread[] { new TestSystemImmediateRemoveDestroy(), }));
systems.Add(new TasksTestSystem());
Native Physics Library
The deterministic math library continues to be moved to a common language and building a native physics library to be shared across different Photon products. There are slight performance improvements relative to an IL2CPP version of our C# library, which was already quite optimized, but long-term maintainability is the main reason for the migration, not performance.
At the time when the Preview SDK is released, only clients running on Windows will be running the majority of physics tasks from the native library. These tasks are ensured to be cross-platform deterministic with their existing C# counterparts running on other platforms and the transition into native will be transparent as more tasks are ported and new platforms are supported.
The usage of native physics library is enabled by default, but can be disabled in the Simulation Config during Preview as a fallback for unpredicted issues. Upcoming new features are planned to be implemented solely in the native library, so, once all platforms are supported and stability conditions are ensured, the option to disable its usage will become obsolete and be eventually removed.
3D Capsule Shape
Yes, Capsules are finally coming! The Preview version brings support to 3D Capsules, with 2D ones coming up soon. All the features already supported by other primitive shapes are in, including shape overlap and cast queries, usage on dynamic bodies and CCD.
Partly Deterministic Navmesh Baking
The Quantum navmesh baking is now deterministic, which is interesting for games that generate a navmesh during runtime. The default pipeline still relies on importing a Unity mesh (non-deterministic). Replacing this will eventually come.
The navmesh baking has been moved from Unity to the simulation code in QuantumGame (see individual release note changes).
Dashboard Options
By default app Quantum3 apps will block non-protocol messages and player properties. Leaving such legacy features open could potentially allow ill-intentioned actors to disrupt matchmaking and gameplay flow of Quantum apps, even though actual gameplay can not be interfered with. To unblock the features they have to be explicitly set on the dashboard properties for individual AppIds.
BlockNonProtocolMessages
(true
/false
)BlockPlayerProperties
(true
/false
)
BlockRoomProperties (New)
Type: boolean
(true
/false
)
The plugin will cancel all room properties set by clients after the room creation. With the exception of a property named StartQuantum
. Initial room properties can also be retrieved with a webhook.
Caveat: This affects Open
and IsVisible
as well.
AllowedLobbyProperties (New)
Type: string
(Allowed separators: , or ; or space)
Maximum number of properties: 3
Maximum string
property length: 64
Set a list of properties that are allowed for the client to send as Lobby Properties as a protection for the matchmaking performances on the master servers. If this property is set, then non-listed properties send by clients will be stripped. The plugin logs Restricted LobbyProperty
found once.
Additionally by default the property types are restricted to: bool
, byte
, short
, int
, long
, string
MaxPlayerSlots (New)
Type: int
By default clients could create as many local players as the game supports. Setting this value will limit this for all games running under this AppId. The value can also be set by the webhooks.
StartPropertyBlockedTimeSec (New)
Type: int
If set to a number greater than zero, the starting of Quantum inside a room will be blocked until the minimum amount of seconds has passed since the room has been created. This can be used to ensure that players have enough time to join before the game begins.
StartPropertyForcedTimeSec (New)
Type: int
If set to a number greater than zero, it specifies the maximum amount of seconds that can elapse before starting Quantum inside a room after the room has been created. If the specified time is exceeded, the game will set the "StartQuantum" property in the room's game properties to true if it hasn't already.
HideRoomAfterStartSec (New)
Type: int
If set to a number greater than zero, it defines the number of seconds after which the room will be hidden from public or search listings once Quantum has started within the room. This can help manage room visibility and ensure that new players do not join games already in progress.
CloseRoomAfterStartSec (New)
Type: int
If set to a number greater than zero, it determines the number of seconds after which the room will be closed following the start of Quantum inside the room. Closing a room prevents any new players from joining and can be used to manage the lifecycle of the game session.
Road Map
Physics System
Most new physics features planned for Quantum 3 are not yet available at the time the Preview is released. They are being implemented in the new native physics library (more in the topic below) and should be released along the alpha and beta stages of the SDK. These features include, to name a few:
- New primitive shape: Capsule (2D)
- KCC AddOn: more flexible and capable of handling more complex use-case scenarios
- Convex Mesh: new shape for static or dynamic colliders
- Stateful physics: better stacking capabilities and more realistic resolution of complex systems of constraints
- Entities with static colliders: regular collider components can be static colliders, which no longer need to be pre-baked into a Map asset
Character Controller
Another iteration of the kinematic character controller based on the new capsule collision checks will be added.
Quantum Build Pipeline
Bringing back the option to develop the Quantum simulation outside of Unity is under consideration.
Compiling the Quantum game dll without Unity dependencies for non-Unity applications like the custom server plugin or the spectator app will be added.
Quantum Plugin SDK
A new plugin SDK will be released based on the newest Photon Server 6 which includes multiple samples of common use cases for custom Quantum plugins.
Additionally a demo will be made available that implements a sample backend in ASP.net
to showcases how Quantum webhooks can be tested and processed.
Protocol Optimization
Another protocol bandwidth optimization is planned (raw messages) on the client and server which will be back-wards compatible.
Back to top- Quantum SDK Is Now A Unitypackage
- Input Delta Compression
- New Protocol For Adding And Removing Players
- Predicted Commands
- Webhooks
- Quantum 3 AppIds
- Photon Realtime 5
- The SessionRunner Class
- New Demo Menu
- RuntimeConfig And RuntimePlayer Json Serialization
- Quantum Hub
- Quantum CodeDoc Inspectors
- Data-Driven System Setup
- Native Physics Library
- 3D Capsule Shape
- Partly Deterministic Navmesh Baking
- Dashboard Options
- BlockRoomProperties (New)
- AllowedLobbyProperties (New)
- MaxPlayerSlots (New)
- StartPropertyBlockedTimeSec (New)
- StartPropertyForcedTimeSec (New)
- HideRoomAfterStartSec (New)
- CloseRoomAfterStartSec (New)
- Road Map