Quantum Project

Unitypackage Content
The Quantum 3 SDK is distributed as a unitypackage
file. It's roughly separated into two sections: Assets/Photon
and Assets/QuantumUser
. The former represents the Quantum SDK and the latter the mutable user code.
After installing Quantum, the user is presented with the following folder structure:
Unknown
Assets
├───Photon
│ ├───PhotonLibs
│ ├───PhotonRealtime
│ └───Quantum
└───QuantumUser
├───Editor
│ └───CodeGen
├───Resources
├───Scenes
├───Simulation
│ └───Generated
└───View
└───Generated
All the deterministic simulation code should be placed in QuantumUser/Simulation
. Any code extending either Quantum.Unity
or Quantum.Unity.Editor
classes should be placed in View
and Editor
, respectively.
User code can either be put into the predefined folder structure or by using AssemblyDefinitionReferences
.
It is possible to opt out of the Quantum assembly definitions, for example for users migrating from such setup in Quantum 2.1 projects.
Assets/Photon
Assets/Photon
: This folder contains Photon Quantum and other packages. Changes to files in here will be overwritten during upgradesAssets/Photon/PhotonLibs (and PhotonRealtime)
: Contains Photon dependencies that Quantum uses to connect and communicate with the Quantum cloud.Assets/Photon/Quantum/Assemblies
: Contains Quantum libraries for Unity and their debug versions.Assets/Photon/Quantum/Editor
: Quantum Unity editor scripts. Compiles toQuantum.Unity.Editor.dll
.Assets/Photon/Quantum/Editor/Assemblies
: Contains the Quantum CodeGen libraries.Assets/Photon/Quantum/Editor/CodeGen
: Includes the Quantum CodeGen scripts. Compiles toQuantum.CodeGen.Qtn.dll
.Assets/Photon/Quantum/Runtime
: Quantum Unity runtime scripts. Compiles toQuantum.Unity.dll
.Assets/Photon/Quantum/Simulation
: Quantum simulation core scripts. Compiles toQuantum.Simulation.dll
.Assets/Photon/Quantum/Samples
: Contains the demo menu scene and currently the GraphProfiler.
Assets/QuantumUser
Assets/QuantumUser
: Files inside this folder will never be overwritten by an upgrade and are under the developers control. It uses assembly definition references to add code to the Quantum and simulation libraries.Assets/QuantumUser/Editor/CodeGen
: Files that extend the Qtn CodeGen.Assets/QuantumUser/Editor/Generated
: Generated Unity Editor scripts.Assets/QuantumUser/Simulation
: The actual simulation code.Assets/QuantumUser/Simulation/Generated
: Generated Quantum C# code.Assets/QuantumUser/Resources
: Runtime config files.Assets/QuantumUser/Scenes
: The default folder for new Quantum scenes.Assets/QuantumUser/View
: Scripts inside this folder extend the Quantum.Unity.dll.Assets/QuantumUser/View/Generated
: Generated Quantum prototype scripts.
Quantum is split into four assemblies:
Quantum.Simulation
: contains simulation code. Any user simulation code should be added to this assembly withAssemblyDefinitionReferences
. Unity/Odin property attributes can be used at will, but any use of non-deterministic Unity API is heavy discouraged. Code from this assembly can be easily worked on as a standalone.csproj
, similar toquantum.code.csproj
in Quantum 2.Quantum.Unity
: contains code specific to Quantum's integration with Unity. Additionally, CodeGen emitsMonoBehaviours
that wraps component prototypes.Quantum.Unity.Editor
: contains editor code forQuantum.Simulation
andQuantum.Unity
Quantum.Unity.Editor.CodeGen
: contains CodeGen integration code. It is fully independent of other Quantum assemblies, so can always be run, even if there are compile errors - this may require exiting Safe Mode.
Quantum Dependencies
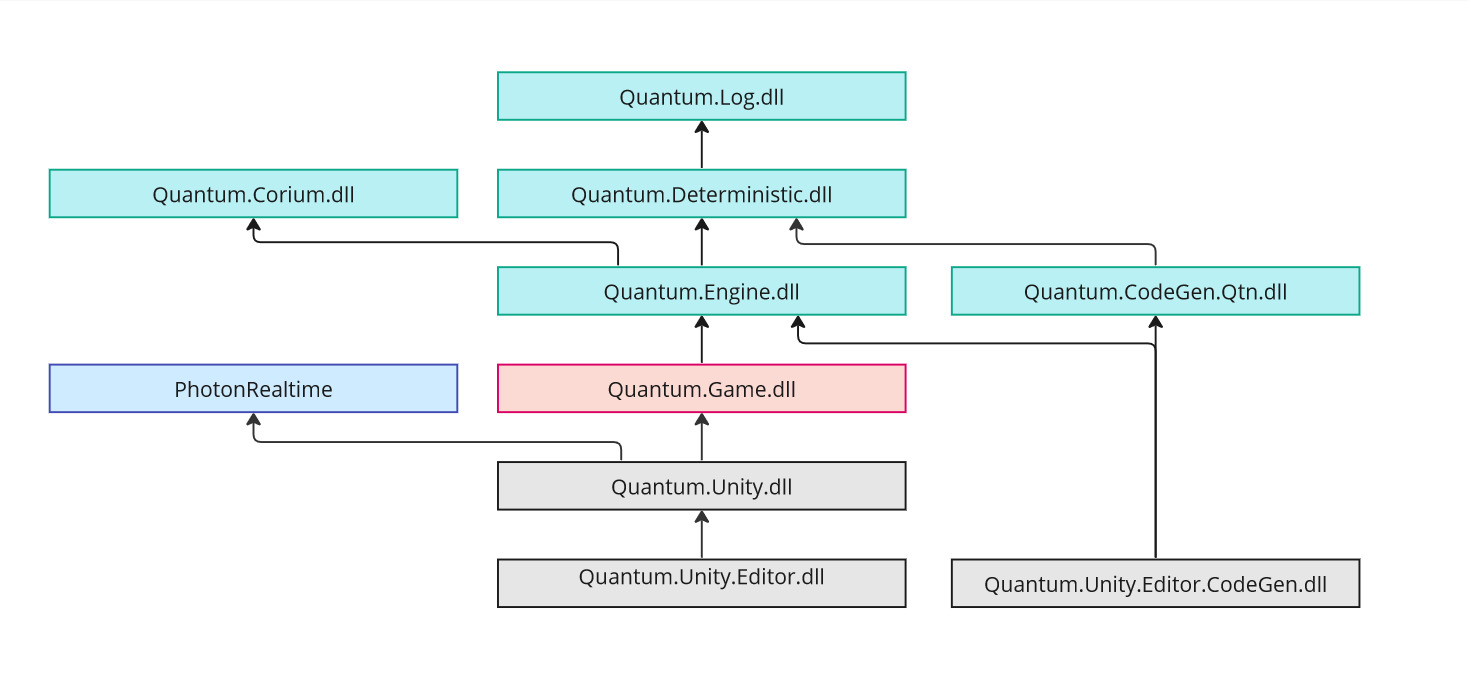
Quantum Hub
Open by pressing Ctrl+H
or via the Quantum menu.
The Hub window will also pop up when a vital Quantum configuration files is missing (e.g. PhotonServerSettings) and recommend pressing the installation button.
The installation process takes care of installing files locally that cannot originate from the unitypackage because they would be overwritten by the next Quantum version upgrade.
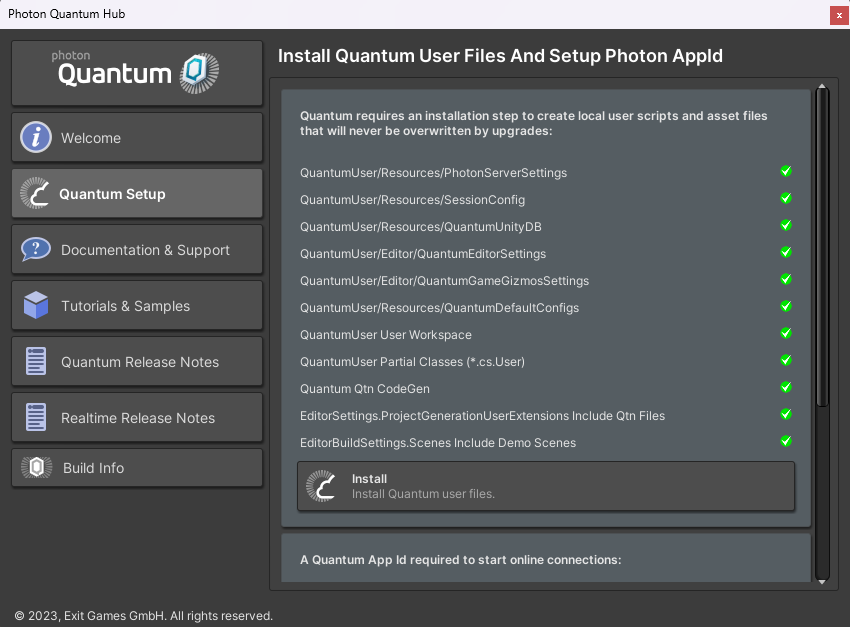
Installing The Quantum Menu
The Quantum menu is an addon that can be installed with the SDK using the Hub. The unitypackage can be found under Asset/Photon/QuantumMenu/Quantum-Menu.unitypackage
.
The menu is a functional and graphical in-game menu to start random online matches or create parties.
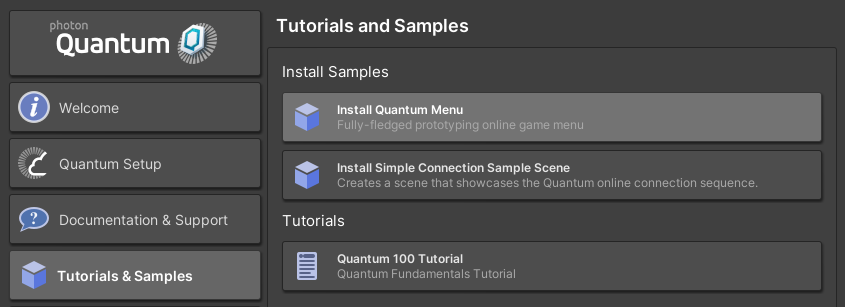
Find more information about customization possibilities here: Sample Menu Customization.
Release And Debug Builds
The Quantum libraries (Quantum.Deterministic.dll, Quantum.Engine.dll and Quantum.Corium.dll) come both in release and debug configurations. To make Unity recognize the correct library the QUANTUM_DEBUG
global scripting define is used.
To toggle between debug and release use the menu:
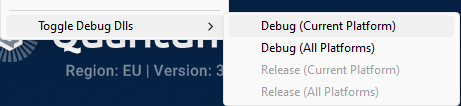
Caveat: because the define needs to be set per platform, there is a risk that the debug version is not disabled for every platform when making release builds for example.
The debug build has significant performance penalties compared to a release build. For performance tests always use a Quantum release build (and Unity IL2CPP). Read more about this in the Profiling section.
The development build contains assertions, exceptions, checks and debug outputs that help during development and which are disabled in release configuration. For example:
Log.Debug()
andLog.Trace()
, when called from the quantum code project, will not be outputting log anymore.- As well as all
Draw.Shape()
methods. NavMeshAgentConfig.ShowDebugAvoidance
andShowDebugSteering
will not draw gizmos anymore.- Assertions and exceptions inside low level systems like physics are disabled.
Logging
Quantum provides the static Quantum.Log
class to log from the simulation code and also to produce all its owns log outputs.
C#
namespace Quantum {
public unsafe class MyQuantumSystem : SystemMainThread
public override void Update(Frame f) {
Log.Debug($"Updating MyQuantumSystem tick {f.Number}");
}
}
}
The Unity SDK has wrapper around that called QuantumUnityLogger
. It will statically initialize itself during RuntimeInitializeOnLoadMethod
and/or InitializeOnLoadMethod
(see QuantumUnityLogger.Initialize()
)
The partial method InitializePartial()
can be used to customize the initialization.
The QuantumUnityLogger
class offers various customizations fields to for example define the color schemes.
Log Level
The global log level is controlled by Quantum.Log.LogLevel
. To set the initial log level use the following scripting defines:
QUANTUM_LOGLEVEL_TRACE
QUANTUM_LOGLEVEL_DEBUG
QUANTUM_LOGLEVEL_INFO
QUANTUM_LOGLEVEL_WARN
QUANTUM_LOGLEVEL_ERROR
They are toggled with the QuantumEditorSettings
inspector.
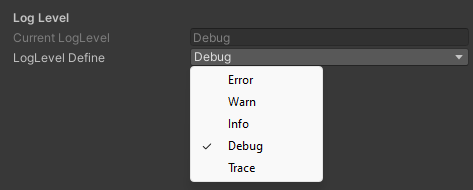
As mentioned in the last section about debug builds Log.Trace()
and Log.Debug()
messages will only be logged with TRACE
and DEBUG
defines respectively. Notice that running the UnityEditor always defines DEBUG
.
Photon Realtime Log
The Photon Realtime library used by Quantum has its own log levels that can be controlled with the Photon.Realtime.AppSettings
found on the PhotonServerSettings
ScriptableObject.
AppSettings.NetworkLogging
: Log level for the PhotonPeer and connection. Useful to debug connection related issues.AppSettings.ClientLogging
: Log level for the RealtimeClient and callbacks. Useful to get info about the client state, servers it uses and operations called.
In non-development builds Realtime logs less than the ERROR
severity will not log unless defining LOG_WARNING
, LOG_INFO
and LOG_DEBUG
.